The ability to effectively manage and manipulate data is more crucial than ever. Structured Query Language, or SQL, stands at the forefront of this endeavor, serving as the backbone for database management across various industries. Whether you’re a budding data analyst, a seasoned developer, or simply someone looking to enhance your skill set, mastering SQL opens the door to a wealth of opportunities.
This comprehensive guide is designed to take you on a journey through the fundamentals of SQL, while also delving into advanced techniques that will elevate your data handling capabilities. You’ll learn how to create and manage databases, execute complex queries, and optimize performance—all essential skills in today’s tech landscape. By the end of this article, you will not only grasp the core concepts of SQL but also gain the confidence to apply them in real-world scenarios, transforming raw data into actionable insights.
Join us as we explore the powerful world of SQL, where data becomes more than just numbers—it becomes a strategic asset for decision-making and innovation.
Getting Started with SQL
Setting Up Your Environment
Installing SQL Server
To begin your journey with SQL, the first step is to install a SQL Server. SQL Server is a relational database management system developed by Microsoft. It is widely used for storing and retrieving data as requested by other software applications. Here’s how to install SQL Server:
- Download SQL Server: Visit the official Microsoft SQL Server download page. You can choose from various editions, including the free SQL Server Express edition, which is perfect for learning and small applications.
- Run the Installer: After downloading, run the installer. You will be presented with several installation options. Choose the “New SQL Server stand-alone installation” option.
- Follow the Setup Wizard: The setup wizard will guide you through the installation process. You will need to accept the license terms, choose the SQL Server edition, and select the features you want to install. For beginners, the default features are usually sufficient.
- Configure Server: During the installation, you will be prompted to configure the server. You can choose the authentication mode (Windows Authentication or Mixed Mode) and add SQL Server administrators.
- Complete Installation: Once you have configured the settings, click on the Install button. After the installation is complete, you can launch SQL Server Management Studio (SSMS) to start working with your databases.
Setting Up a Local Database
After installing SQL Server, the next step is to set up a local database. This allows you to create, manage, and manipulate your data without needing an internet connection. Here’s how to create a local database:
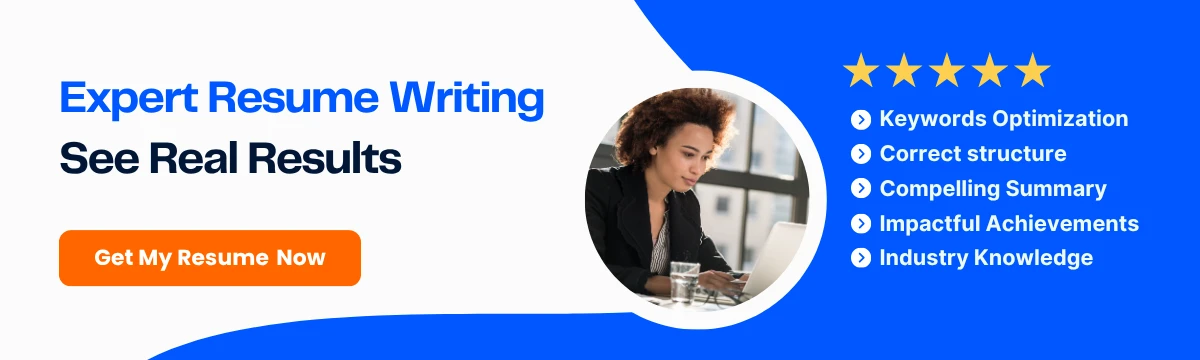
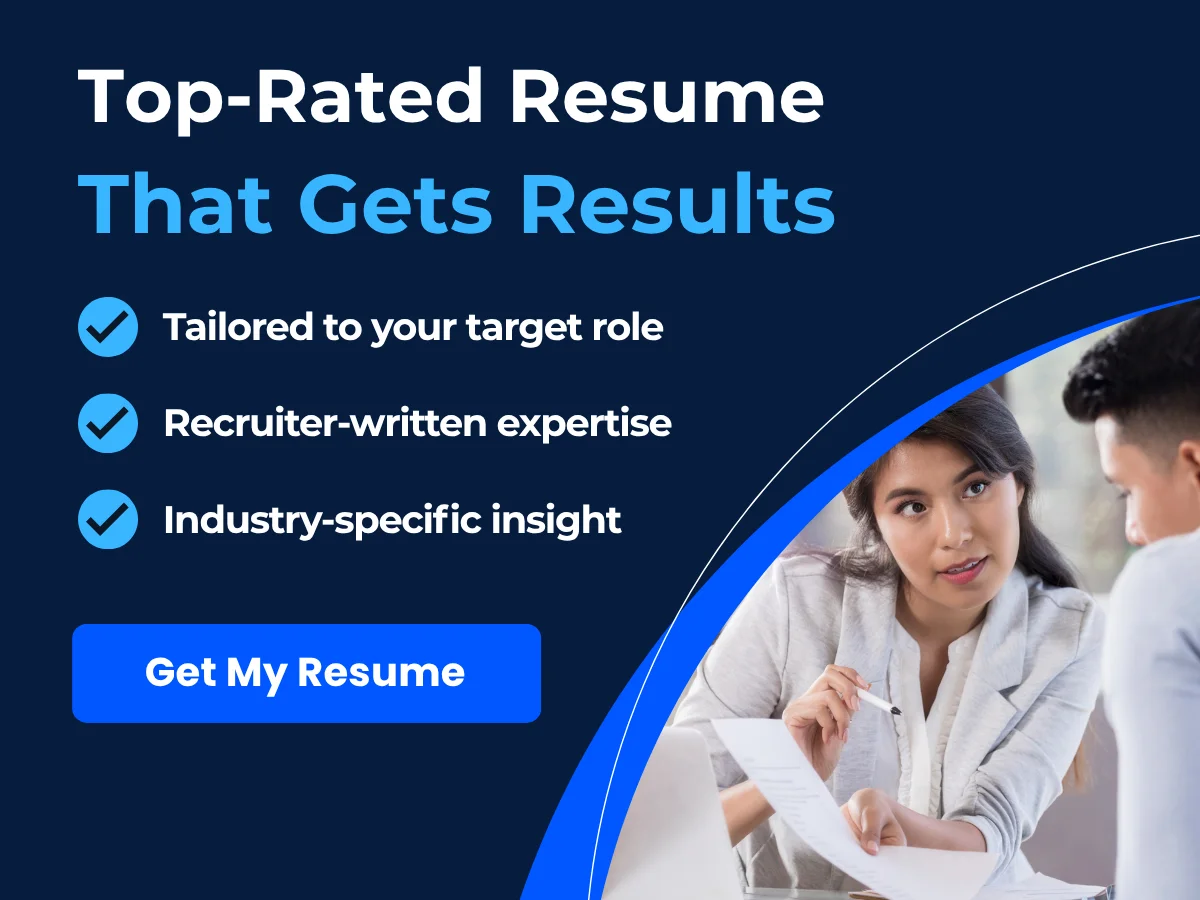
- Open SQL Server Management Studio (SSMS): Launch SSMS and connect to your SQL Server instance using the credentials you set during installation.
- Create a New Database: In the Object Explorer, right-click on the “Databases” node and select “New Database.” A dialog box will appear where you can enter the database name and configure settings such as file paths and sizes.
- Define Database Properties: After entering the database name, you can click on the “Options” page to configure additional properties like collation and recovery model. For most beginners, the default settings are adequate.
- Click OK: Once you have configured the settings, click OK to create the database. You will see your new database listed under the “Databases” node in the Object Explorer.
Cloud-Based SQL Solutions
In addition to local installations, many cloud-based SQL solutions are available, providing flexibility and scalability. These services allow you to access your databases from anywhere with an internet connection. Here are some popular cloud-based SQL solutions:
- Amazon RDS: Amazon Relational Database Service (RDS) makes it easy to set up, operate, and scale a relational database in the cloud. It supports several database engines, including MySQL, PostgreSQL, and SQL Server.
- Google Cloud SQL: Google Cloud SQL is a fully-managed database service that allows you to set up, maintain, manage, and administer relational databases on Google Cloud Platform. It supports MySQL, PostgreSQL, and SQL Server.
- Microsoft Azure SQL Database: Azure SQL Database is a managed cloud database provided as part of Microsoft Azure. It offers high availability, scalability, and security, making it a great choice for enterprise applications.
To get started with any of these cloud solutions, you typically need to create an account, select a database engine, and follow the provider’s setup instructions. Most platforms offer free tiers or trial periods, allowing you to experiment without incurring costs.
Basic SQL Syntax
SQL Statements Overview
Structured Query Language (SQL) is the standard language for interacting with relational databases. SQL statements are used to perform tasks such as querying data, updating records, and managing database structures. Here are the primary types of SQL statements:
- Data Query Language (DQL): This includes the
SELECT
statement, which is used to retrieve data from a database. For example:
SELECT * FROM Employees;
INSERT
, UPDATE
, and DELETE
that are used to manipulate data within tables. For example:INSERT INTO Employees (Name, Position) VALUES ('John Doe', 'Developer');
CREATE
, ALTER
, and DROP
that define and modify database structures. For example:CREATE TABLE Employees (ID INT PRIMARY KEY, Name VARCHAR(100), Position VARCHAR(100));
GRANT
and REVOKE
that control access to data in the database.Case Sensitivity in SQL
Understanding case sensitivity in SQL is crucial for writing effective queries. SQL is generally case-insensitive, meaning that keywords can be written in any combination of upper and lower case. For example, the following statements are equivalent:
SELECT * FROM Employees;
select * from employees;
However, case sensitivity can depend on the database system and the collation settings. For instance, in some systems, table names and column names may be case-sensitive. It’s a good practice to be consistent with your casing to avoid confusion and potential errors.
Comments in SQL
Comments are an essential part of writing SQL code, as they help document your queries and make them easier to understand for others (or yourself in the future). SQL supports two types of comments:
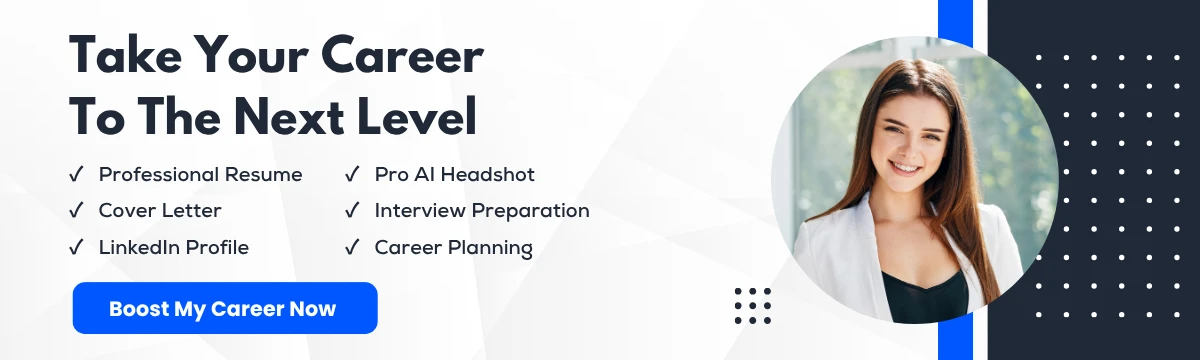
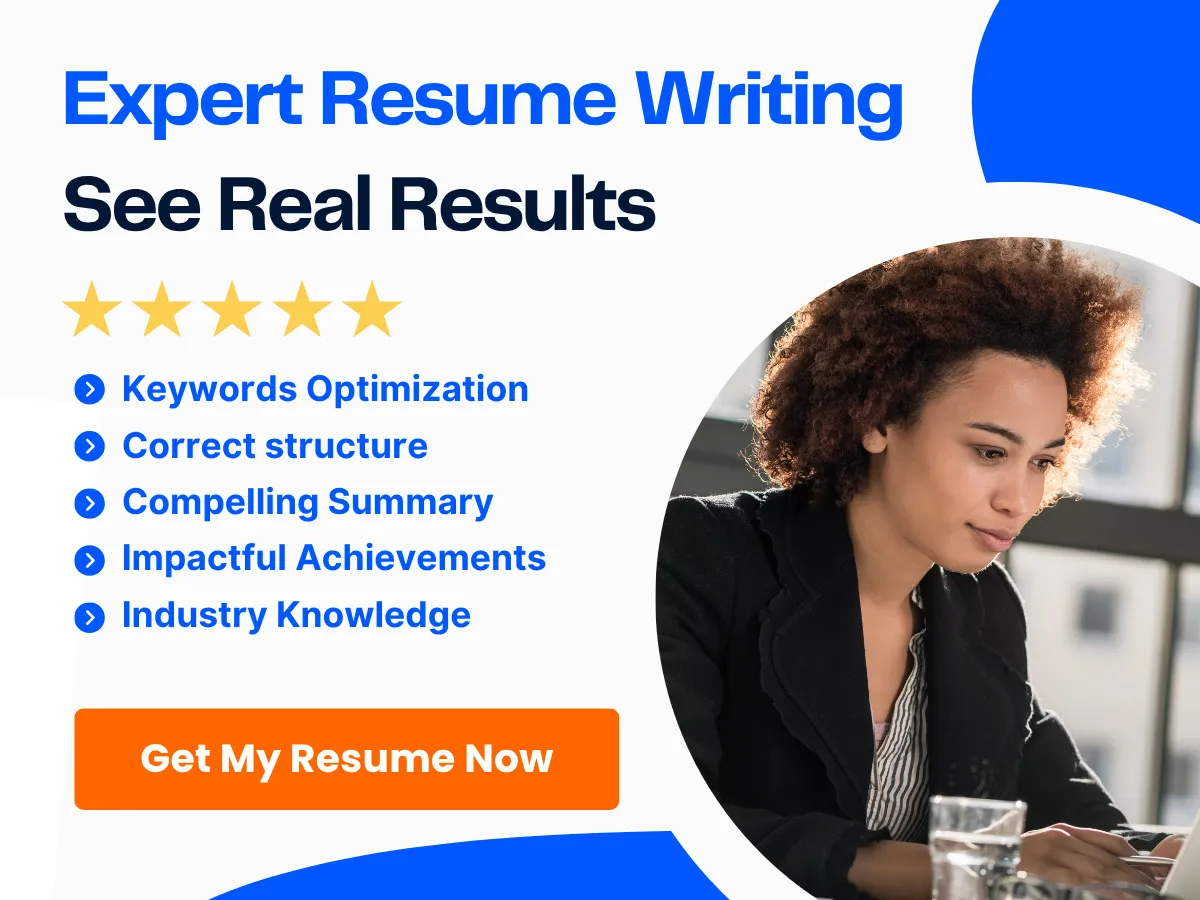
- Single-line comments: These comments start with two dashes (
--
) and continue to the end of the line. For example:
-- This is a single-line comment
SELECT * FROM Employees;
/*
and */
. They can span multiple lines. For example:/*
This is a multi-line comment
that spans multiple lines
*/
SELECT * FROM Employees;
Using comments effectively can greatly enhance the readability of your SQL code, making it easier for others to follow your logic and understand your intentions.
Core SQL Concepts
Data Types
Understanding data types is fundamental to mastering SQL. Data types define the kind of data that can be stored in a column of a table. Each database management system (DBMS) may have its own set of data types, but most share common categories. Here, we will explore the primary data types used in SQL.
Numeric Data Types
Numeric data types are used to store numbers. They can be further divided into two categories: integers and floating-point numbers.
- Integer Types: These are whole numbers without any decimal points. Common integer types include:
TINYINT
: A very small integer that can hold values from 0 to 255 (1 byte).SMALLINT
: A small integer that can hold values from -32,768 to 32,767 (2 bytes).MEDIUMINT
: A medium-sized integer that can hold values from -8,388,608 to 8,388,607 (3 bytes).INT
orINTEGER
: A standard integer that can hold values from -2,147,483,648 to 2,147,483,647 (4 bytes).BIGINT
: A large integer that can hold values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 (8 bytes).- Floating-Point Types: These are used for numbers that require decimal points. Common floating-point types include:
FLOAT
: A floating-point number that can store approximate values (4 bytes).DOUBLE
: A double-precision floating-point number that can store approximate values (8 bytes).DECIMAL
orNUMERIC
: These types are used for fixed-point numbers, allowing for precise storage of decimal values. You can define the precision and scale, e.g.,DECIMAL(10,2)
allows for 10 digits in total, with 2 digits after the decimal point.
String Data Types
String data types are used to store text. They can vary in length and characteristics:
- CHAR: A fixed-length string. If the string is shorter than the defined length, it is padded with spaces. For example,
CHAR(10)
will always store 10 characters. - VARCHAR: A variable-length string. It can store up to a specified number of characters without padding. For example,
VARCHAR(255)
can store up to 255 characters. - TEXT: A string data type for large amounts of text. It can store up to 65,535 characters, depending on the DBMS.
- ENUM: A string object that can have one value chosen from a list of permitted values. For example,
ENUM('small', 'medium', 'large')
allows only these three values.
Date and Time Data Types
Date and time data types are essential for storing temporal data. They allow you to track when events occur:
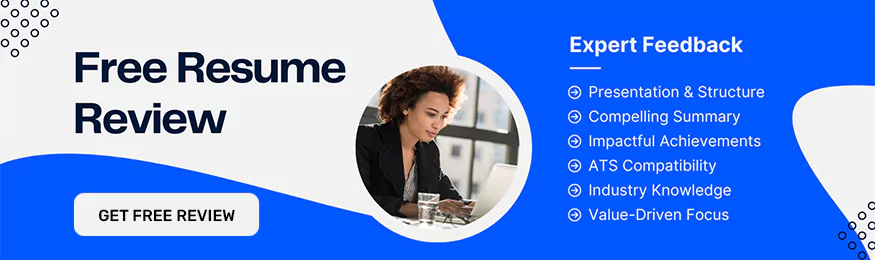
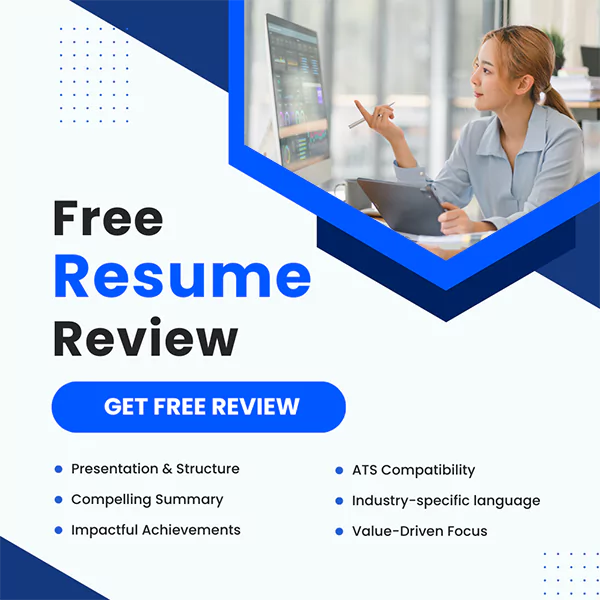
- DATE: Stores a date value in the format
YYYY-MM-DD
. For example,2023-10-01
. - TIME: Stores a time value in the format
HH:MM:SS
. For example,14:30:00
. - DATETIME: Combines date and time into a single value, formatted as
YYYY-MM-DD HH:MM:SS
. For example,2023-10-01 14:30:00
. - TIMESTAMP: Similar to DATETIME but also includes time zone information. It is often used for tracking changes in records.
- YEAR: Stores a year in either 2-digit or 4-digit format. For example,
2023
or23
.
Other Data Types
In addition to the primary categories, SQL supports several other data types:
- BIT: A data type that can hold a single bit of data, typically used for boolean values (0 or 1).
- BLOB: A Binary Large Object used to store binary data, such as images or files.
- JSON: A data type for storing JSON (JavaScript Object Notation) formatted data, allowing for flexible data structures.
- XML: A data type for storing XML (eXtensible Markup Language) formatted data, useful for hierarchical data representation.
Database Design
Database design is a critical aspect of creating efficient and effective databases. It involves structuring the data in a way that supports the intended use cases while ensuring data integrity and performance.
Tables and Schemas
A database is composed of tables, which are the fundamental building blocks of data storage. Each table consists of rows and columns:
- Tables: A table is a collection of related data entries. Each table has a unique name and contains rows (records) and columns (fields). For example, a
Customers
table might have columns forCustomerID
,Name
,Email
, andPhone
. - Schemas: A schema is a blueprint of how the database is constructed. It defines the tables, fields, relationships, and constraints. Schemas help organize and manage the database structure, making it easier to understand and maintain.
Primary Keys and Foreign Keys
Keys are essential for maintaining data integrity and establishing relationships between tables:
- Primary Keys: A primary key is a unique identifier for each record in a table. It ensures that no two rows have the same value in the primary key column(s). For example, in a
Customers
table,CustomerID
could serve as the primary key. - Foreign Keys: A foreign key is a field (or collection of fields) in one table that uniquely identifies a row in another table. It establishes a relationship between the two tables. For instance, if you have an
Orders
table, it might include aCustomerID
foreign key that links to theCustomers
table.
Indexes and Constraints
Indexes and constraints are used to optimize performance and enforce rules within the database:
- Indexes: An index is a database object that improves the speed of data retrieval operations on a table. It works like an index in a book, allowing the database to find rows more quickly. However, indexes can slow down data modification operations (INSERT, UPDATE, DELETE) because the index must also be updated. You can create an index on one or more columns using the
CREATE INDEX
statement. - Constraints: Constraints are rules applied to columns in a table to enforce data integrity. Common types of constraints include:
NOT NULL:
Ensures that a column cannot have a NULL value.UNIQUE:
Ensures that all values in a column are different.CHECK:
Ensures that all values in a column satisfy a specific condition.DEFAULT:
Sets a default value for a column when no value is specified.
By understanding these core SQL concepts, you will be well-equipped to design and manage databases effectively, ensuring data integrity and optimizing performance.
CRUD Operations
CRUD operations are the fundamental building blocks of any database interaction. The acronym stands for Create, Read, Update, and Delete, which represent the four basic functions of persistent storage. We will delve into each of these operations in detail, providing you with the knowledge and skills necessary to manipulate data effectively in SQL.

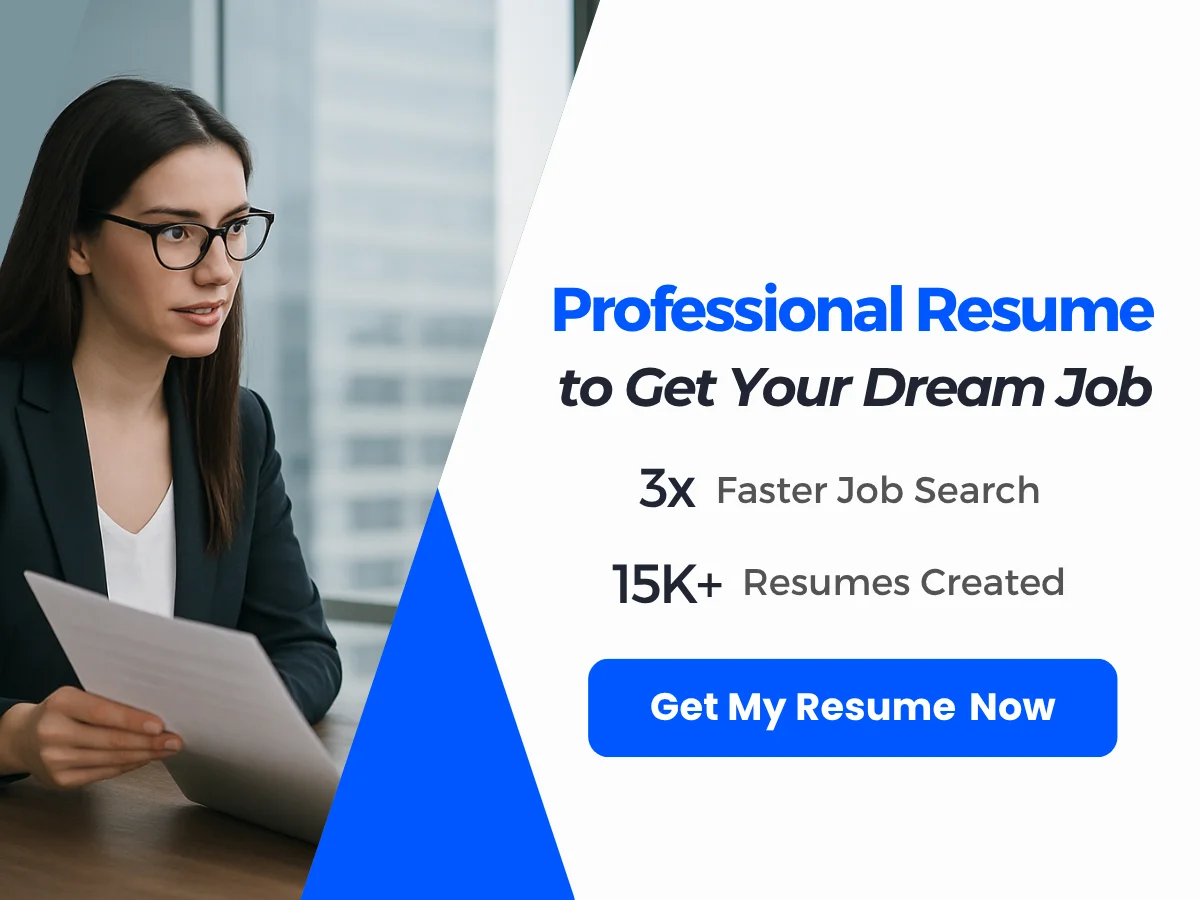
Creating Data
The INSERT Statement
The INSERT
statement is used to add new records to a table. The basic syntax for the INSERT
statement is as follows:
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
For example, if we have a table named employees
with columns first_name
, last_name
, and email
, we can insert a new employee record like this:
INSERT INTO employees (first_name, last_name, email)
VALUES ('John', 'Doe', '[email protected]');
It’s important to ensure that the values you are inserting match the data types defined for each column in the table schema. If you attempt to insert a string into an integer column, for example, you will encounter an error.
Bulk Inserts
Inserting multiple records at once can be done using a single INSERT
statement. This is known as a bulk insert. The syntax is similar to the standard INSERT
statement, but you can provide multiple sets of values:
INSERT INTO employees (first_name, last_name, email)
VALUES
('Alice', 'Smith', '[email protected]'),
('Bob', 'Johnson', '[email protected]'),
('Charlie', 'Brown', '[email protected]');
Bulk inserts are more efficient than inserting records one at a time, especially when dealing with large datasets. They reduce the number of transactions and can significantly improve performance.
Reading Data
The SELECT Statement
The SELECT
statement is used to query the database and retrieve data from one or more tables. The basic syntax is:
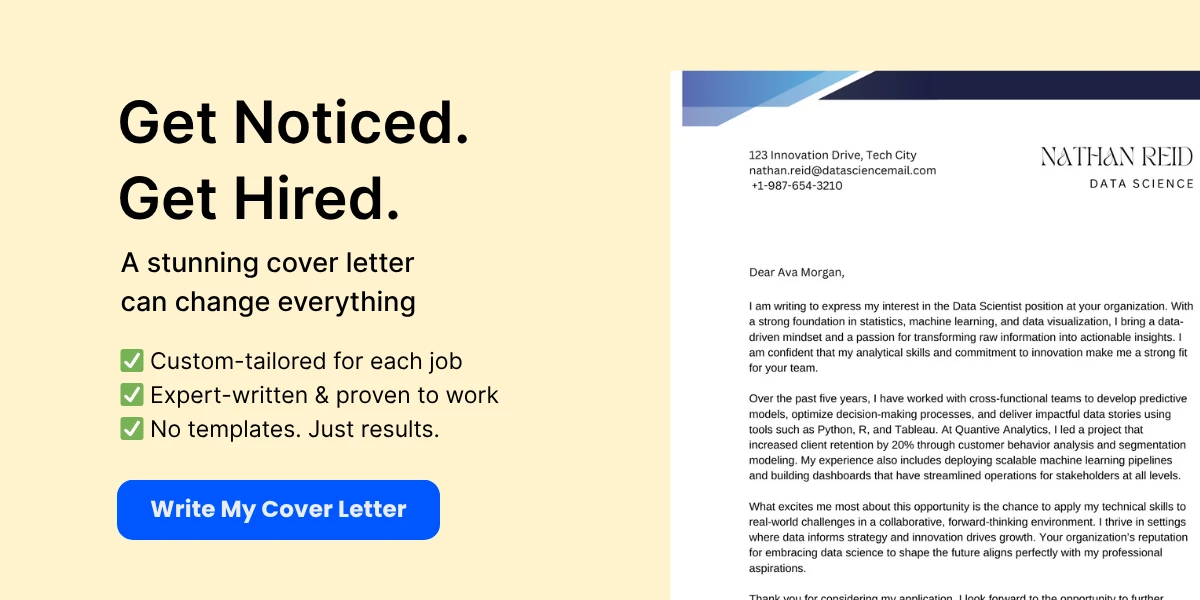
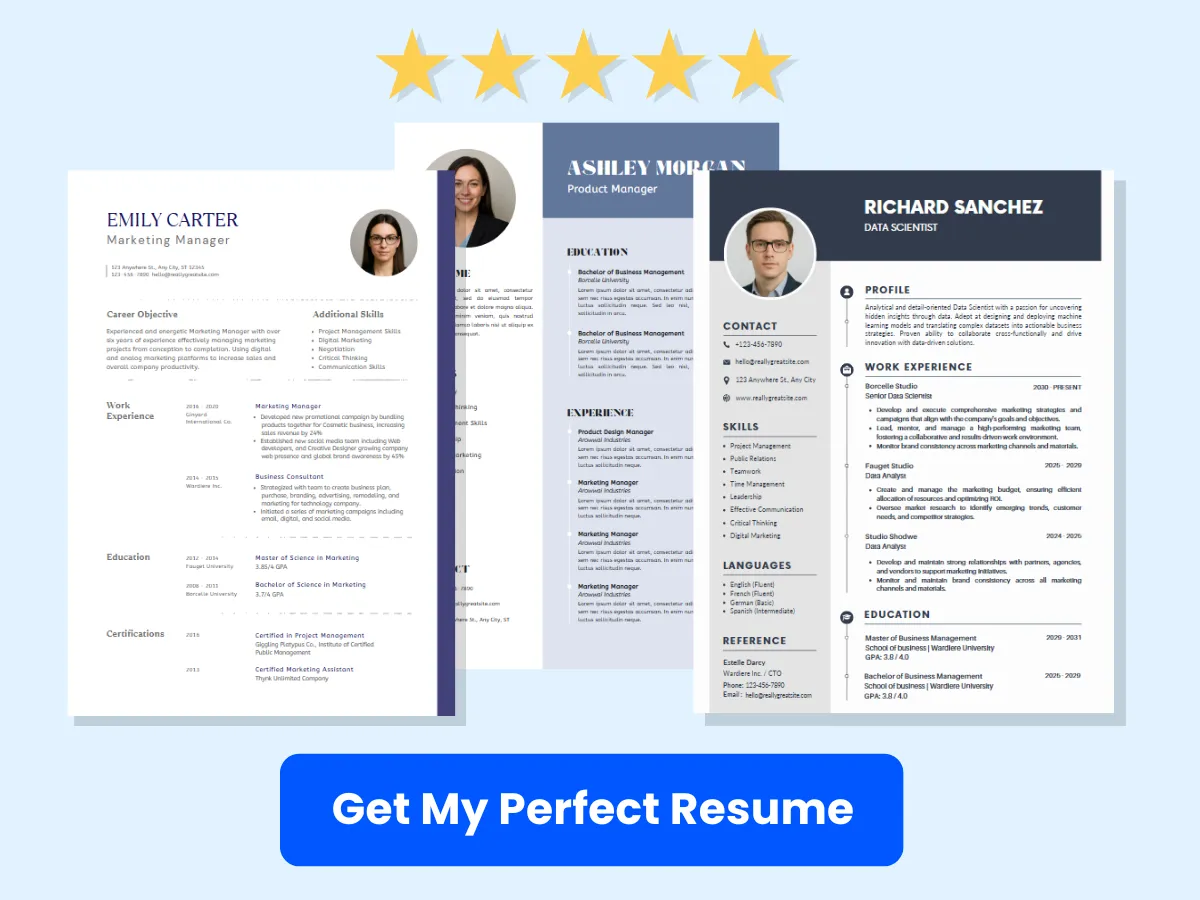
SELECT column1, column2, ...
FROM table_name;
To select all columns from a table, you can use the asterisk (*
) wildcard:
SELECT * FROM employees;
This will return all records and all columns from the employees
table.
Filtering with WHERE
To filter the results returned by a SELECT
statement, you can use the WHERE
clause. This allows you to specify conditions that the data must meet to be included in the results:
SELECT * FROM employees
WHERE last_name = 'Doe';
This query will return all records from the employees
table where the last_name
is ‘Doe’. You can also use logical operators such as AND
and OR
to combine multiple conditions:
SELECT * FROM employees
WHERE last_name = 'Doe' AND first_name = 'John';
Sorting with ORDER BY
To sort the results of a query, you can use the ORDER BY
clause. By default, the results are sorted in ascending order. To sort in descending order, you can specify DESC
:
SELECT * FROM employees
ORDER BY last_name ASC;
To sort by multiple columns, you can list them in the ORDER BY
clause:
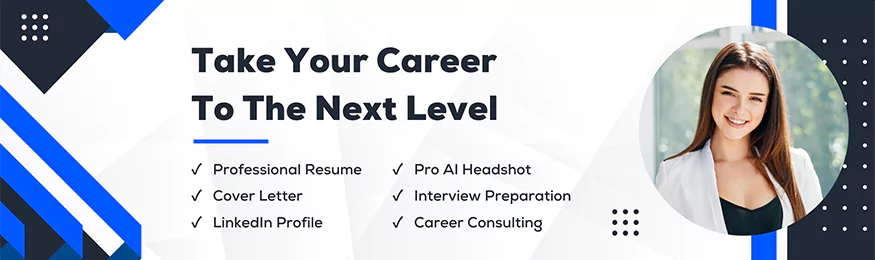
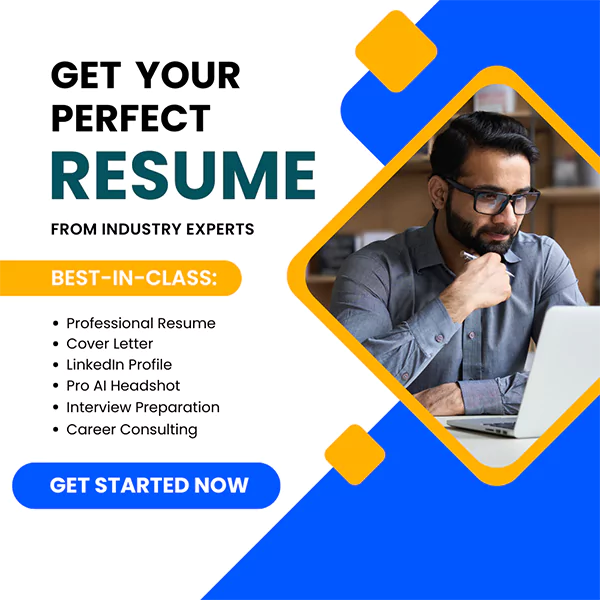
SELECT * FROM employees
ORDER BY last_name ASC, first_name DESC;
Limiting Results with LIMIT and OFFSET
When working with large datasets, you may want to limit the number of records returned by a query. The LIMIT
clause allows you to specify the maximum number of records to return:
SELECT * FROM employees
LIMIT 5;
This query will return only the first five records from the employees
table. You can also use the OFFSET
clause to skip a specified number of records before starting to return rows:
SELECT * FROM employees
LIMIT 5 OFFSET 10;
This will return five records, starting from the eleventh record in the result set.
Updating Data
The UPDATE Statement
The UPDATE
statement is used to modify existing records in a table. The basic syntax is:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
For example, if we want to update the email address of an employee with the last name ‘Doe’, we can do the following:
UPDATE employees
SET email = '[email protected]'
WHERE last_name = 'Doe';
It’s crucial to include a WHERE
clause in your UPDATE
statement to avoid updating all records in the table unintentionally.
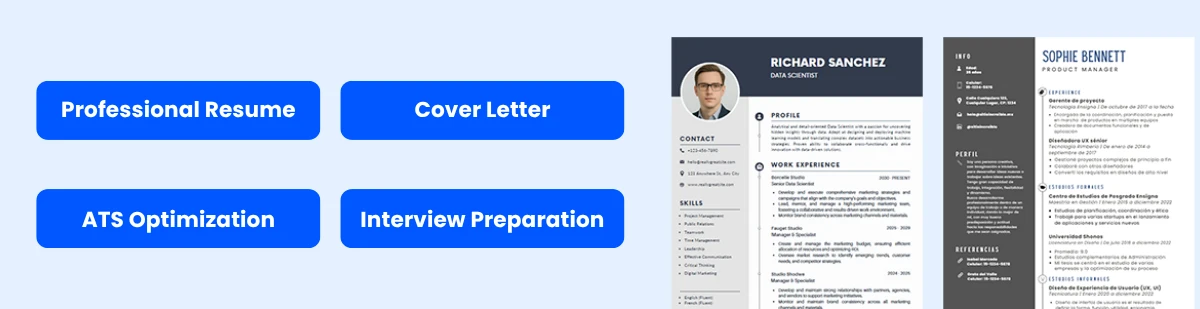
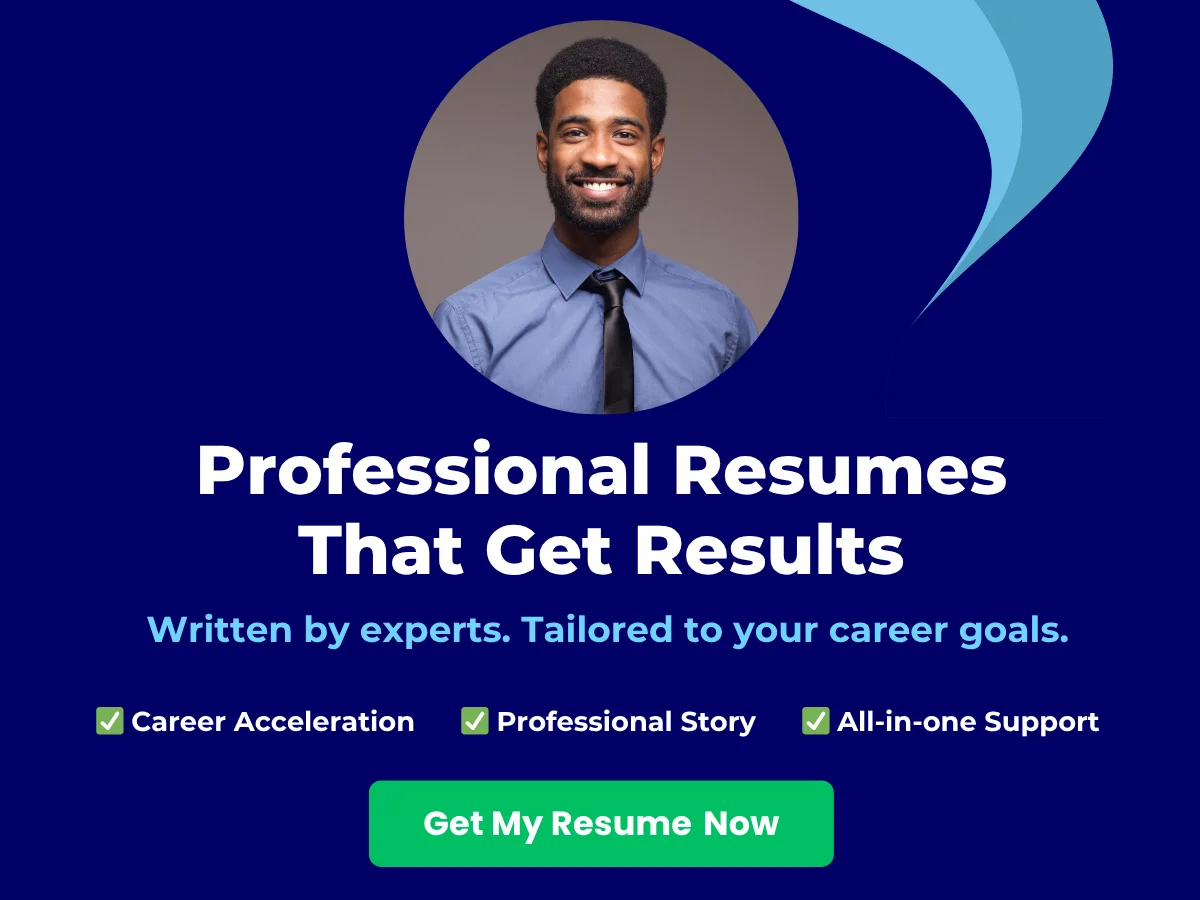
Conditional Updates
Conditional updates allow you to modify records based on specific criteria. You can use various operators in the WHERE
clause to define the conditions:
UPDATE employees
SET email = '[email protected]'
WHERE first_name = 'John' AND last_name = 'Doe';
This query updates the email address only for the employee named John Doe. You can also use subqueries in the SET
clause to update records based on values from other tables.
Deleting Data
The DELETE Statement
The DELETE
statement is used to remove records from a table. The syntax is:
DELETE FROM table_name
WHERE condition;
For example, to delete an employee record where the last name is ‘Doe’, you would write:
DELETE FROM employees
WHERE last_name = 'Doe';
As with the UPDATE
statement, it is essential to include a WHERE
clause to prevent deleting all records in the table.
Truncating Tables
If you want to remove all records from a table without deleting the table itself, you can use the TRUNCATE
statement. This operation is faster than a DELETE
statement without a WHERE
clause because it does not log individual row deletions:
TRUNCATE TABLE employees;
Keep in mind that TRUNCATE
cannot be rolled back in most database systems, so use it with caution. It is a powerful command that resets any auto-increment counters and removes all data from the table instantly.
Mastering CRUD operations is essential for anyone working with SQL databases. Understanding how to create, read, update, and delete data will empower you to manage and manipulate your data effectively, laying a solid foundation for more advanced SQL techniques.
Advanced SQL Queries
Joins
Joins are a fundamental aspect of SQL that allow you to combine rows from two or more tables based on a related column between them. Understanding how to use joins effectively is crucial for querying relational databases. Below, we explore the different types of joins available in SQL.
Inner Join
The Inner Join returns only the rows that have matching values in both tables. It is the most common type of join. For example, consider two tables: employees
and departments
.
SELECT employees.name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.id;
This query retrieves the names of employees along with their corresponding department names, but only for those employees who are assigned to a department.
Left Join
The Left Join (or Left Outer Join) returns all the rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for columns from the right table.
SELECT employees.name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.id;
In this case, all employees will be listed, even those who do not belong to any department. For those employees, the department_name
will be NULL.
Right Join
The Right Join (or Right Outer Join) is the opposite of the Left Join. It returns all the rows from the right table and the matched rows from the left table. If there is no match, NULL values are returned for columns from the left table.
SELECT employees.name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.id;
This query will return all departments, including those that have no employees assigned to them, with NULL for the employee names where applicable.
Full Outer Join
The Full Outer Join combines the results of both Left and Right Joins. It returns all rows from both tables, with NULLs in places where there is no match.
SELECT employees.name, departments.department_name
FROM employees
FULL OUTER JOIN departments ON employees.department_id = departments.id;
This query will return all employees and all departments, showing NULLs where there are no matches in either table.
Cross Join
The Cross Join produces a Cartesian product of the two tables involved, meaning it returns all possible combinations of rows from both tables.
SELECT employees.name, departments.department_name
FROM employees
CROSS JOIN departments;
This query will return a list of every employee paired with every department, which can lead to a very large result set if both tables contain many rows.
Subqueries
Subqueries, or nested queries, are queries within another SQL query. They can be used in various clauses such as SELECT, WHERE, and FROM. Subqueries can be categorized into two types: inline subqueries and correlated subqueries.
Inline Subqueries
An Inline Subquery is a subquery that is used in the SELECT statement or in the WHERE clause. It is executed once for the parent query.
SELECT name, (SELECT COUNT(*) FROM projects WHERE projects.employee_id = employees.id) AS project_count
FROM employees;
This query retrieves the names of employees along with the count of projects they are assigned to, using an inline subquery to calculate the project count for each employee.
Correlated Subqueries
A Correlated Subquery is a subquery that references columns from the outer query. It is executed once for each row processed by the outer query.
SELECT name
FROM employees e
WHERE salary > (SELECT AVG(salary) FROM employees WHERE department_id = e.department_id);
This query finds employees whose salary is above the average salary of their respective departments. The subquery is correlated because it refers to the outer query’s department_id
.
Set Operations
Set operations allow you to combine the results of two or more SELECT statements. The main set operations in SQL are UNION, UNION ALL, INTERSECT, and EXCEPT.
UNION and UNION ALL
The UNION operator combines the results of two or more SELECT statements and removes duplicate rows from the result set. In contrast, UNION ALL includes all duplicates.
SELECT name FROM employees
UNION
SELECT name FROM contractors;
This query retrieves a list of unique names from both the employees
and contractors
tables. If you want to include duplicates, you would use UNION ALL
.
INTERSECT
The INTERSECT operator returns only the rows that are present in both SELECT statements.
SELECT name FROM employees
INTERSECT
SELECT name FROM contractors;
This query retrieves names that are found in both the employees
and contractors
tables.
EXCEPT
The EXCEPT operator returns rows from the first SELECT statement that are not present in the second SELECT statement.
SELECT name FROM employees
EXCEPT
SELECT name FROM contractors;
This query retrieves names of employees who are not contractors, effectively filtering out any names that appear in both tables.
Grouping and Aggregation
Grouping and aggregation are essential for summarizing data in SQL. The GROUP BY
clause is used to arrange identical data into groups, and aggregate functions perform calculations on these groups.
GROUP BY Clause
The GROUP BY
clause groups rows that have the same values in specified columns into summary rows. It is often used with aggregate functions.
SELECT department_id, COUNT(*) AS employee_count
FROM employees
GROUP BY department_id;
This query counts the number of employees in each department by grouping the results based on department_id
.
Aggregate Functions
Aggregate functions perform calculations on a set of values and return a single value. Common aggregate functions include:
- SUM(): Calculates the total sum of a numeric column.
- AVG(): Calculates the average value of a numeric column.
- COUNT(): Counts the number of rows in a set.
- MIN(): Returns the smallest value in a set.
- MAX(): Returns the largest value in a set.
For example, to find the total salary expenditure per department, you could use:
SELECT department_id, SUM(salary) AS total_salary
FROM employees
GROUP BY department_id;
HAVING Clause
The HAVING
clause is used to filter groups based on a specified condition, similar to the WHERE
clause but applied to aggregated data.
SELECT department_id, COUNT(*) AS employee_count
FROM employees
GROUP BY department_id
HAVING COUNT(*) > 10;
This query retrieves departments that have more than ten employees, filtering the results after the grouping has been performed.
Mastering these advanced SQL queries will significantly enhance your ability to manipulate and analyze data effectively. By understanding joins, subqueries, set operations, and aggregation, you can perform complex queries that yield valuable insights from your data.
SQL Functions
SQL functions are essential tools that allow you to manipulate and analyze data effectively. They can be categorized into several types, including string functions, numeric functions, date and time functions, and conversion functions. We will explore each category in detail, providing examples and insights to help you master these functions.
String Functions
String functions are used to perform operations on string data types. They allow you to manipulate text, extract substrings, and measure string lengths. Here are some of the most commonly used string functions:
CONCAT
The CONCAT
function is used to concatenate two or more strings into a single string. This function is particularly useful when you want to combine data from different columns or add static text to your results.
SELECT CONCAT(first_name, ' ', last_name) AS full_name
FROM employees;
In this example, the CONCAT
function combines the first_name
and last_name
columns with a space in between, resulting in a full name for each employee.
SUBSTRING
The SUBSTRING
function extracts a portion of a string based on specified starting position and length. This is useful for retrieving specific parts of a string.
SELECT SUBSTRING(phone_number, 1, 3) AS area_code
FROM contacts;
Here, the SUBSTRING
function extracts the first three characters from the phone_number
column, which typically represents the area code.
LENGTH
The LENGTH
function returns the number of characters in a string. This can be useful for validating data or understanding the size of text entries.
SELECT LENGTH(email) AS email_length
FROM users;
This query retrieves the length of each email address in the users
table, allowing you to analyze the distribution of email lengths.
Numeric Functions
Numeric functions are used to perform calculations on numeric data types. They can help you round numbers, calculate averages, and perform other mathematical operations. Here are some key numeric functions:
ROUND
The ROUND
function rounds a numeric value to a specified number of decimal places. This is particularly useful for financial calculations where precision is important.
SELECT ROUND(salary, 2) AS rounded_salary
FROM employees;
In this example, the ROUND
function rounds the salary
values to two decimal places, making it easier to read and understand.
CEIL
The CEIL
function returns the smallest integer greater than or equal to a given numeric value. This can be useful for scenarios where you need to ensure that a value meets a minimum threshold.
SELECT CEIL(average_score) AS ceiling_score
FROM test_results;
This query retrieves the ceiling value of the average_score
from the test_results
table, ensuring that scores are rounded up to the nearest whole number.
FLOOR
The FLOOR
function returns the largest integer less than or equal to a given numeric value. This is useful for truncating decimal values without rounding.
SELECT FLOOR(discount_rate) AS floored_discount
FROM sales;
In this example, the FLOOR
function truncates the discount_rate
to the nearest whole number, which can be useful for reporting purposes.
Date and Time Functions
Date and time functions are crucial for managing and manipulating date and time data types. They allow you to perform calculations, format dates, and extract specific components. Here are some important date and time functions:
NOW
The NOW
function returns the current date and time. This is useful for timestamping records or calculating time differences.
SELECT NOW() AS current_timestamp;
This query retrieves the current date and time from the database server, which can be used for logging or auditing purposes.
DATEADD
The DATEADD
function adds a specified interval to a date. This is useful for calculating future or past dates based on a given date.
SELECT DATEADD(day, 30, order_date) AS delivery_date
FROM orders;
In this example, the DATEADD
function adds 30 days to the order_date
, providing an estimated delivery date for each order.
DATEDIFF
The DATEDIFF
function calculates the difference between two dates, returning the result in days. This can be useful for determining the duration between events.
SELECT DATEDIFF(NOW(), hire_date) AS days_since_hired
FROM employees;
This query calculates the number of days since each employee was hired, which can be useful for tracking tenure or eligibility for benefits.
Conversion Functions
Conversion functions are used to convert data from one type to another. This is particularly useful when dealing with different data formats or when you need to ensure compatibility between data types. Here are some common conversion functions:
CAST
The CAST
function converts an expression from one data type to another. This is useful for ensuring that data types match when performing operations.
SELECT CAST(price AS DECIMAL(10, 2)) AS formatted_price
FROM products;
In this example, the CAST
function converts the price
column to a decimal format with two decimal places, ensuring consistent formatting for financial data.
CONVERT
The CONVERT
function is similar to CAST
but offers additional formatting options, particularly for date and time conversions.
SELECT CONVERT(VARCHAR, order_date, 101) AS formatted_order_date
FROM orders;
This query converts the order_date
to a string format (MM/DD/YYYY), making it more readable for reports or user interfaces.
Understanding and mastering SQL functions is crucial for effective data manipulation and analysis. By leveraging string, numeric, date and time, and conversion functions, you can enhance your SQL queries and gain deeper insights from your data.
Database Management
Transactions
In the realm of database management, transactions are a fundamental concept that ensures data integrity and consistency. A transaction is a sequence of operations performed as a single logical unit of work. A transaction must be completed in its entirety or not at all, which is crucial for maintaining the accuracy of the database.
ACID Properties
Transactions are governed by the ACID properties, which stand for Atomicity, Consistency, Isolation, and Durability. Understanding these properties is essential for anyone looking to master SQL and database management.
- Atomicity: This property ensures that all operations within a transaction are completed successfully. If any operation fails, the entire transaction is aborted, and the database is left unchanged. For example, if a bank transfer involves deducting money from one account and adding it to another, both operations must succeed; otherwise, the transaction fails, and no changes are made.
- Consistency: A transaction must bring the database from one valid state to another, maintaining all predefined rules, including constraints and triggers. For instance, if a transaction violates a foreign key constraint, it will not be allowed to complete.
- Isolation: This property ensures that transactions are executed in isolation from one another. Even if multiple transactions are occurring simultaneously, each transaction should not interfere with others. This is crucial for preventing issues like dirty reads, non-repeatable reads, and phantom reads.
- Durability: Once a transaction has been committed, its changes are permanent, even in the event of a system failure. This means that the database will retain the changes made by the transaction, ensuring data integrity.
COMMIT and ROLLBACK
In SQL, the COMMIT
and ROLLBACK
commands are used to manage transactions. The COMMIT
command is used to save all changes made during the transaction, while the ROLLBACK
command is used to undo changes if an error occurs.
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
COMMIT;
In the example above, if both updates are successful, the changes are committed. However, if an error occurs after the first update, you can use ROLLBACK
to revert the changes:
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
-- An error occurs here
ROLLBACK;
Stored Procedures
Stored procedures are a powerful feature of SQL that allows you to encapsulate complex operations into a single callable routine. They are stored in the database and can be executed with a simple command, making them an efficient way to manage repetitive tasks.
Creating and Executing Stored Procedures
Creating a stored procedure involves defining the procedure with a specific name, parameters, and the SQL statements to be executed. Here’s a basic example:
CREATE PROCEDURE TransferFunds
@FromAccount INT,
@ToAccount INT,
@Amount DECIMAL(10, 2)
AS
BEGIN
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - @Amount WHERE account_id = @FromAccount;
UPDATE accounts SET balance = balance + @Amount WHERE account_id = @ToAccount;
COMMIT;
END;
To execute the stored procedure, you would use the following command:
EXEC TransferFunds @FromAccount = 1, @ToAccount = 2, @Amount = 100.00;
Advantages of Stored Procedures
Stored procedures offer several advantages:
- Performance: Since stored procedures are precompiled and stored in the database, they can execute faster than individual SQL statements sent from an application.
- Security: Stored procedures can help enhance security by restricting direct access to the underlying tables. Users can be granted permission to execute the procedure without having direct access to the tables.
- Maintainability: Changes to business logic can be made in one place (the stored procedure) rather than in multiple application code locations, making maintenance easier.
- Reduced Network Traffic: By executing multiple SQL statements in a single call, stored procedures can reduce the amount of data sent over the network.
Views
Views are virtual tables in SQL that provide a way to present data from one or more tables in a specific format. They can simplify complex queries and enhance security by restricting access to specific data.
Creating and Managing Views
Creating a view is straightforward. You can define a view using the CREATE VIEW
statement followed by a query that selects the data you want to include:
CREATE VIEW AccountBalances AS
SELECT account_id, balance
FROM accounts
WHERE balance > 0;
Once created, you can query the view just like a regular table:
SELECT * FROM AccountBalances;
To modify a view, you can use the CREATE OR REPLACE VIEW
statement:
CREATE OR REPLACE VIEW AccountBalances AS
SELECT account_id, balance, account_type
FROM accounts
WHERE balance > 0;
Materialized Views
Materialized views are a special type of view that stores the result set of a query physically. Unlike regular views, which are virtual and compute their data on-the-fly, materialized views can improve performance for complex queries by caching the results.
To create a materialized view, you would use a syntax similar to that of a regular view, but with the MATERIALIZED
keyword:
CREATE MATERIALIZED VIEW AccountSummary AS
SELECT account_type, SUM(balance) AS total_balance
FROM accounts
GROUP BY account_type;
Materialized views can be refreshed to update their data, either on demand or at scheduled intervals, depending on the database system.
Triggers
Triggers are special types of stored procedures that automatically execute in response to certain events on a particular table or view. They are useful for enforcing business rules, maintaining audit trails, and ensuring data integrity.
Creating and Managing Triggers
To create a trigger, you use the CREATE TRIGGER
statement, specifying the event that will activate the trigger (such as INSERT, UPDATE, or DELETE) and the action to be performed:
CREATE TRIGGER UpdateAccountBalance
AFTER UPDATE ON accounts
FOR EACH ROW
BEGIN
INSERT INTO account_audit (account_id, old_balance, new_balance, change_date)
VALUES (OLD.account_id, OLD.balance, NEW.balance, NOW());
END;
This trigger logs changes to the account balance into an audit table whenever an account is updated.
Use Cases for Triggers
Triggers can be used in various scenarios, including:
- Data Validation: Triggers can enforce business rules by validating data before it is inserted or updated in the database.
- Auditing: Triggers can automatically log changes to data, providing a history of modifications for compliance and auditing purposes.
- Enforcing Referential Integrity: Triggers can help maintain relationships between tables by preventing actions that would violate foreign key constraints.
- Automating Tasks: Triggers can automate routine tasks, such as updating summary tables or sending notifications when certain conditions are met.
Performance Optimization
Indexing
Indexing is a crucial aspect of database performance optimization. It involves creating a data structure that improves the speed of data retrieval operations on a database table at the cost of additional space and maintenance overhead. Proper indexing can significantly enhance query performance, especially in large databases.
Types of Indexes
There are several types of indexes that can be utilized in SQL databases, each serving different purposes:
- B-Tree Indexes: The most common type of index, B-Tree indexes are structured in a balanced tree format. They allow for efficient searching, insertion, and deletion operations. B-Tree indexes are ideal for range queries and are the default index type in many SQL databases.
- Hash Indexes: These indexes use a hash table to find data quickly. They are particularly useful for equality comparisons but are not suitable for range queries. Hash indexes are often used in scenarios where quick lookups are necessary.
- Bitmap Indexes: Bitmap indexes are efficient for columns with a low cardinality (few unique values). They use bitmaps to represent the presence or absence of a value, making them ideal for analytical queries and data warehousing.
- Full-Text Indexes: These indexes are designed for searching text-based data. They allow for complex queries against text columns, enabling features like stemming and relevance ranking.
- Spatial Indexes: Used for geographic data, spatial indexes optimize queries that involve spatial data types, such as points, lines, and polygons.
Creating and Managing Indexes
Creating an index in SQL is straightforward. The basic syntax for creating an index is as follows:
CREATE INDEX index_name ON table_name (column1, column2, ...);
For example, to create an index on the last_name
column of a customers
table, you would use:
CREATE INDEX idx_lastname ON customers (last_name);
Managing indexes involves monitoring their performance and making adjustments as necessary. You can drop an index if it is no longer needed or if it negatively impacts performance:
DROP INDEX index_name ON table_name;
Additionally, some databases support the ability to rebuild indexes to improve performance, especially if they become fragmented over time.
Indexing Best Practices
To maximize the benefits of indexing, consider the following best practices:
- Index Selectively: Avoid indexing every column. Focus on columns that are frequently used in WHERE clauses, JOIN conditions, or as part of an ORDER BY clause.
- Limit the Number of Indexes: While indexes can speed up read operations, they can slow down write operations (INSERT, UPDATE, DELETE). Striking a balance is essential.
- Use Composite Indexes Wisely: Composite indexes (indexes on multiple columns) can be beneficial, but they should be used judiciously. The order of columns in a composite index matters; place the most selective columns first.
- Regularly Monitor Index Usage: Use database tools to analyze index usage and identify unused or underused indexes that can be removed.
- Consider the Impact of Data Changes: As data changes, the effectiveness of an index can diminish. Regularly review and adjust your indexing strategy based on data growth and query patterns.
Query Optimization
Query optimization is the process of improving the performance of SQL queries. It involves analyzing and rewriting queries to ensure they run as efficiently as possible. Effective query optimization can lead to significant performance improvements, especially in large databases.
Analyzing Query Performance
To optimize queries, you first need to analyze their performance. This can be done using various tools and techniques:
- Execution Time: Measure how long a query takes to execute. This can often be done using built-in database functions or profiling tools.
- Resource Usage: Monitor CPU and memory usage during query execution to identify resource-intensive queries.
- Row Counts: Check how many rows are being processed. A query that processes a large number of rows may need optimization.
Using EXPLAIN and Execution Plans
One of the most powerful tools for query optimization is the EXPLAIN
statement, which provides insight into how a query will be executed. It shows the execution plan, detailing how tables are joined, the order of operations, and which indexes are used.
For example, to analyze a query, you can use:
EXPLAIN SELECT * FROM customers WHERE last_name = 'Smith';
The output will include information such as:
- Table: The table being accessed.
- Type: The type of join being used (e.g., ALL, index, range).
- Possible Keys: The indexes that could be used for the query.
- Key: The actual index that is being used.
- Rows: The estimated number of rows that will be examined.
By analyzing the execution plan, you can identify potential bottlenecks and areas for improvement, such as adding indexes or rewriting the query.
Common Performance Pitfalls
When optimizing queries, be aware of common performance pitfalls that can hinder efficiency:
- Using SELECT *: Avoid using
SELECT *
as it retrieves all columns, which can be inefficient. Instead, specify only the columns you need. - Not Using Indexes: Ensure that your queries are designed to take advantage of existing indexes. If a query is not using an index, consider rewriting it.
- Overusing Subqueries: While subqueries can be useful, they can also lead to performance issues. Consider using JOINs instead, as they are often more efficient.
- Ignoring Data Types: Ensure that the data types of columns in WHERE clauses match. Mismatched data types can lead to full table scans instead of index usage.
- Neglecting to Analyze Query Patterns: Regularly review and analyze query patterns to identify opportunities for optimization. As data and usage patterns change, so should your queries.
By understanding and implementing effective indexing and query optimization strategies, you can significantly enhance the performance of your SQL databases, leading to faster response times and improved user experiences.
Security in SQL
In the realm of database management, security is paramount. SQL databases are often the backbone of applications, storing sensitive information that must be protected from unauthorized access and malicious attacks. This section delves into the critical aspects of SQL security, including user management, data encryption, and the ever-looming threat of SQL injection.
User Management
User management is the first line of defense in securing your SQL database. It involves creating, managing, and monitoring user accounts to ensure that only authorized personnel have access to sensitive data.
Creating and Managing Users
Creating users in SQL is a straightforward process, but it requires careful consideration of the permissions granted to each user. Most SQL databases, such as MySQL, PostgreSQL, and Microsoft SQL Server, provide commands to create users and assign them specific roles.
-- MySQL example
CREATE USER 'newuser'@'localhost' IDENTIFIED BY 'password';
In this example, a new user named ‘newuser’ is created with a password. The user is restricted to connecting from the local machine. It’s essential to use strong passwords and to follow best practices for password management.
Once users are created, managing them involves updating their permissions as needed. This can include granting or revoking access to specific databases or tables.
-- Granting privileges
GRANT SELECT, INSERT ON database_name.* TO 'newuser'@'localhost';
-- Revoking privileges
REVOKE INSERT ON database_name.* FROM 'newuser'@'localhost';
Regular audits of user accounts and permissions are crucial. This ensures that users who no longer require access are removed and that permissions are not overly permissive.
Roles and Permissions
Roles are a powerful feature in SQL that allows you to group permissions together. Instead of assigning permissions to individual users, you can create roles that encapsulate a set of permissions and then assign those roles to users. This simplifies user management and enhances security.
-- Creating a role in PostgreSQL
CREATE ROLE read_only;
-- Granting permissions to the role
GRANT SELECT ON ALL TABLES IN SCHEMA public TO read_only;
-- Assigning the role to a user
GRANT read_only TO newuser;
By using roles, you can easily manage permissions for multiple users at once. For example, if a new employee joins the team, you can simply assign them the appropriate role rather than configuring individual permissions.
Data Encryption
Data encryption is a critical component of database security. It ensures that even if data is intercepted or accessed without authorization, it remains unreadable without the proper decryption keys.
Encryption at Rest
Encryption at rest refers to the protection of data stored on disk. This is particularly important for sensitive information such as personal identification numbers, credit card details, and confidential business data. Most modern SQL databases support encryption at rest.
For example, in MySQL, you can enable encryption at rest by configuring the InnoDB storage engine:
-- Enabling encryption in MySQL
SET GLOBAL innodb_encrypt_tables=ON;
SET GLOBAL innodb_encrypt_log=ON;
Once enabled, all new tables created in the InnoDB storage engine will be encrypted. Existing tables can also be encrypted using the ALTER TABLE
command.
Encryption in Transit
Encryption in transit protects data as it travels over the network. This is crucial for preventing eavesdropping and man-in-the-middle attacks. Most SQL databases support SSL/TLS connections, which encrypt data during transmission.
To enable SSL in MySQL, you can configure the server and client to use SSL certificates:
-- MySQL server configuration
[mysqld]
require_secure_transport = ON
ssl_cert = /path/to/server-cert.pem
ssl_key = /path/to/server-key.pem
ssl_ca = /path/to/ca-cert.pem
On the client side, you can connect using SSL by specifying the appropriate options:
mysql --ssl-ca=/path/to/ca-cert.pem --ssl-cert=/path/to/client-cert.pem --ssl-key=/path/to/client-key.pem -u user -p
By ensuring that data is encrypted both at rest and in transit, you significantly reduce the risk of data breaches and unauthorized access.
SQL Injection
SQL injection is one of the most common and dangerous security vulnerabilities in web applications. It occurs when an attacker is able to manipulate SQL queries by injecting malicious code into input fields. This can lead to unauthorized access, data leaks, and even complete database compromise.
Exploring SQL Injection
SQL injection attacks typically exploit vulnerabilities in applications that do not properly sanitize user input. For example, consider a simple login form that constructs a SQL query based on user input:
-- Vulnerable SQL query
SELECT * FROM users WHERE username = '$username' AND password = '$password';
If an attacker inputs a specially crafted username, such as ' OR '1'='1
, the query becomes:
SELECT * FROM users WHERE username = '' OR '1'='1' AND password = '';
This query will always return true, allowing the attacker to bypass authentication and gain access to the application.
Prevention Techniques
Preventing SQL injection requires a multi-faceted approach. Here are some effective techniques:
- Parameterized Queries: Use parameterized queries or prepared statements to ensure that user input is treated as data, not executable code. For example, in PHP with PDO:
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username AND password = :password');
$stmt->execute(['username' => $username, 'password' => $password]);
By implementing these prevention techniques, you can significantly reduce the risk of SQL injection attacks and enhance the overall security of your SQL database.
Tools and Resources
SQL Clients and IDEs
When working with SQL, having the right tools can significantly enhance your productivity and efficiency. SQL clients and Integrated Development Environments (IDEs) are essential for writing, testing, and managing SQL queries and databases. Below, we explore some popular SQL clients and the features you should look for when choosing the right one for your needs.
Popular SQL Clients
- MySQL Workbench: This is a popular choice for MySQL database management. It offers a visual tool for database design, SQL development, and server administration. MySQL Workbench provides a user-friendly interface and supports various database management tasks.
- pgAdmin: As the most popular open-source administration and development platform for PostgreSQL, pgAdmin offers a powerful graphical interface for managing PostgreSQL databases. It includes features like a query tool, a dashboard for monitoring server activity, and a comprehensive set of management tools.
- SQL Server Management Studio (SSMS): This is the primary tool for managing Microsoft SQL Server databases. SSMS provides a rich environment for database development, administration, and management, including a powerful query editor and integrated debugging tools.
- DBeaver: A universal database tool that supports a wide range of databases, including MySQL, PostgreSQL, SQLite, Oracle, and more. DBeaver is open-source and offers a robust set of features, including a SQL editor, ER diagrams, and data migration tools.
- HeidiSQL: A lightweight and easy-to-use SQL client for MySQL, MariaDB, and PostgreSQL. HeidiSQL allows users to browse and edit data, create and edit tables, and execute SQL queries with a simple interface.
Features to Look For
When selecting an SQL client or IDE, consider the following features to ensure it meets your needs:
- User Interface: A clean and intuitive user interface can make a significant difference in your productivity. Look for clients that offer customizable layouts and easy navigation.
- Query Editor: A robust query editor with syntax highlighting, code completion, and error detection can help you write SQL queries more efficiently. Some editors also provide features like query formatting and execution plans.
- Database Management Tools: Features such as data import/export, backup and restore, and schema management are essential for effective database administration.
- Support for Multiple Databases: If you work with different database systems, consider a client that supports multiple database types to streamline your workflow.
- Community and Support: A strong user community and available support resources can be invaluable, especially when you encounter issues or need guidance.
Learning Resources
Mastering SQL requires continuous learning and practice. Fortunately, there are numerous resources available to help you enhance your SQL skills, from books and online courses to community forums. Below, we explore some of the best learning resources for SQL.
Books, Courses, and Tutorials
- Books:
- SQL in 10 Minutes, Sams Teach Yourself by Ben Forta: This book is perfect for beginners and provides a quick and practical introduction to SQL.
- Learning SQL by Alan Beaulieu: A comprehensive guide that covers the fundamentals of SQL, including querying, data manipulation, and database design.
- SQL Cookbook by Anthony Molinaro: This book offers practical solutions to common SQL problems, making it a great resource for intermediate to advanced users.
- Online Courses:
- SQL for Data Science on Coursera: This course is designed for beginners and covers the basics of SQL with a focus on data science applications.
- Intro to SQL on Udacity: A free course that teaches the fundamentals of SQL, including querying and data manipulation.
- Learn SQL on Codecademy: An interactive platform that offers hands-on SQL exercises and projects to reinforce learning.
- Tutorials:
- W3Schools SQL Tutorial: A comprehensive online tutorial that covers SQL syntax, commands, and functions with interactive examples.
- SQL Tutorial: A beginner-friendly resource that provides detailed explanations of SQL concepts and practical examples.
- TutorialsPoint SQL Tutorial: A well-structured tutorial that covers SQL basics, advanced topics, and practical examples.
Online Communities and Forums
Engaging with online communities can provide valuable insights, support, and networking opportunities. Here are some popular forums and communities where you can connect with other SQL learners and professionals:
- Stack Overflow: A popular Q&A platform where you can ask questions, share knowledge, and find solutions to SQL-related problems. The SQL tag is one of the most active areas on the site.
- Reddit: Subreddits like r/SQL and r/learnSQL are great places to discuss SQL topics, share resources, and seek advice from fellow learners.
- SQLServerCentral: A community dedicated to SQL Server professionals, offering articles, forums, and resources for learning and sharing knowledge about SQL Server.
- Database Administrators Stack Exchange: A Q&A site specifically for database administrators, where you can ask questions and share expertise on SQL and database management.
By leveraging these tools and resources, you can enhance your SQL skills, stay updated with the latest trends, and connect with a community of like-minded individuals. Whether you are just starting or looking to deepen your knowledge, the right resources can make all the difference in your SQL journey.
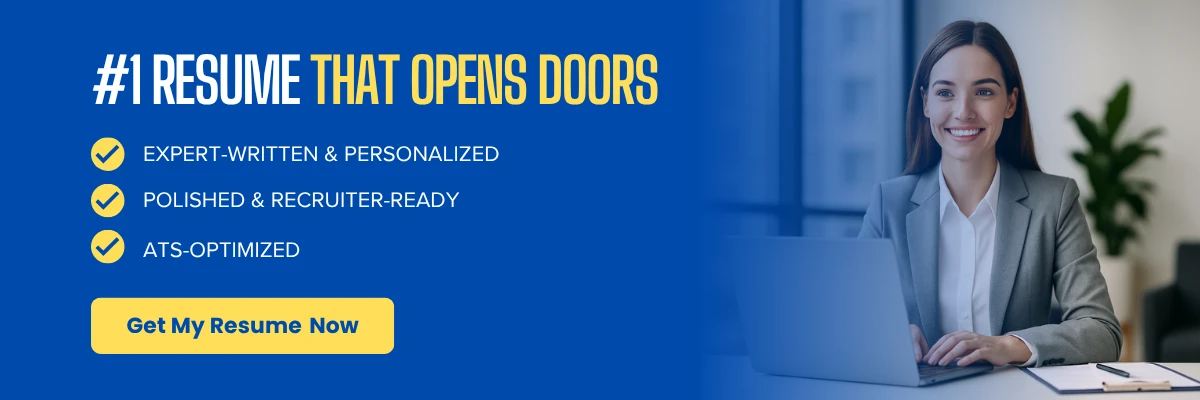
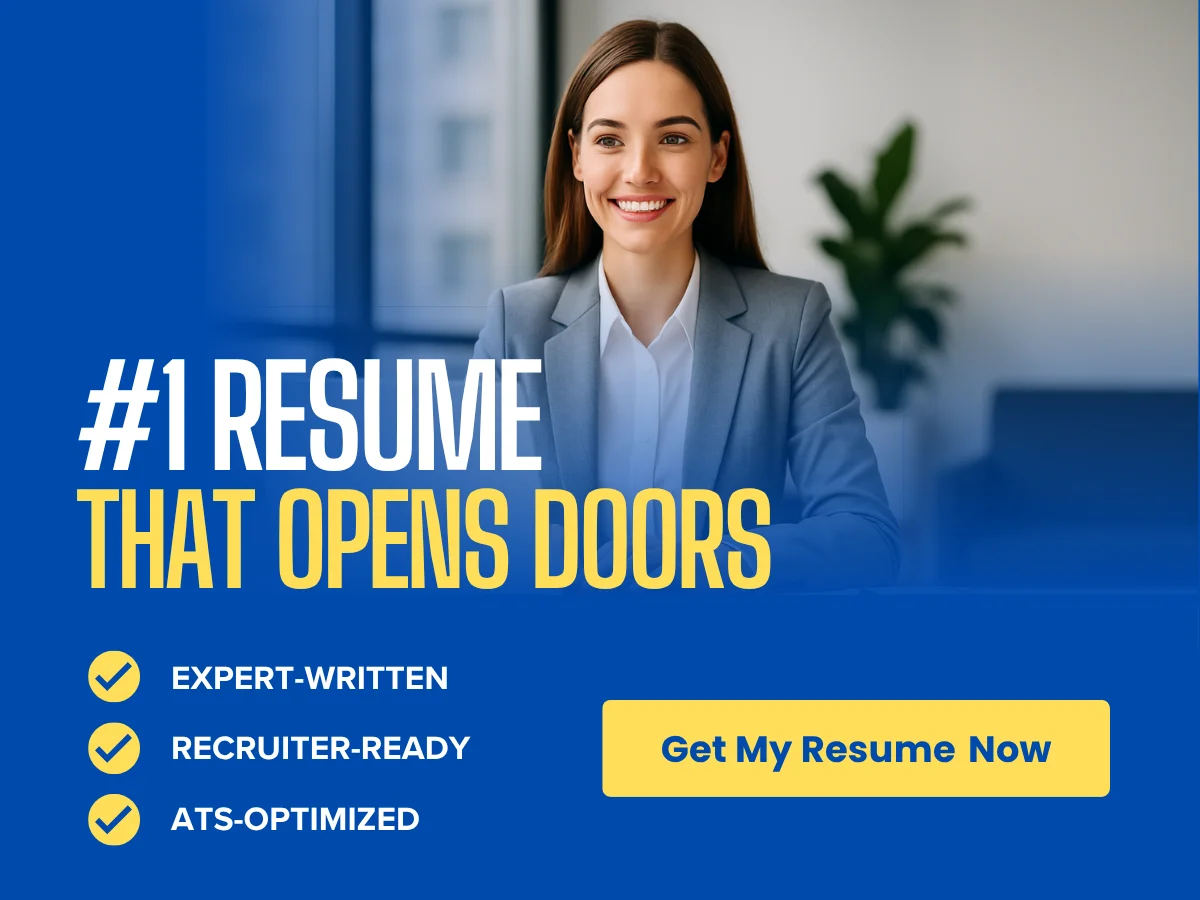