In the ever-evolving landscape of web development, JavaScript stands as a cornerstone technology, powering everything from dynamic user interfaces to complex server-side applications. As the demand for skilled JavaScript developers continues to surge, so does the need for effective preparation for job interviews in this competitive field. Whether you’re a seasoned developer brushing up on your knowledge or a newcomer eager to make your mark, understanding the nuances of JavaScript is crucial for success.
This article presents a comprehensive collection of the top 75 JavaScript interview questions, designed to equip you with the insights and knowledge necessary to excel in your next interview. We’ll cover a wide range of topics, from fundamental concepts to advanced techniques, ensuring that you have a well-rounded grasp of the language. By exploring these questions, you’ll not only enhance your technical skills but also gain confidence in articulating your understanding of JavaScript during interviews.
Prepare to dive deep into the world of JavaScript, where you’ll discover key concepts, best practices, and common pitfalls that can make or break your interview performance. With this ultimate list at your fingertips, you’ll be well on your way to impressing potential employers and securing your dream job in the tech industry.
Basic JavaScript Questions
What is JavaScript?
JavaScript is a high-level, dynamic, untyped, and interpreted programming language that is widely used for web development. It was originally created by Brendan Eich in 1995 and has since become an essential part of the web alongside HTML and CSS. JavaScript enables interactive web pages and is an integral part of web applications. It allows developers to implement complex features on web pages, such as animated graphics, interactive forms, and real-time content updates.
JavaScript is an event-driven, functional, and imperative language. It supports object-oriented programming and is often used in conjunction with various frameworks and libraries, such as React, Angular, and Vue.js, to build modern web applications. Additionally, JavaScript can be executed on the server side using environments like Node.js, allowing for full-stack development using a single programming language.
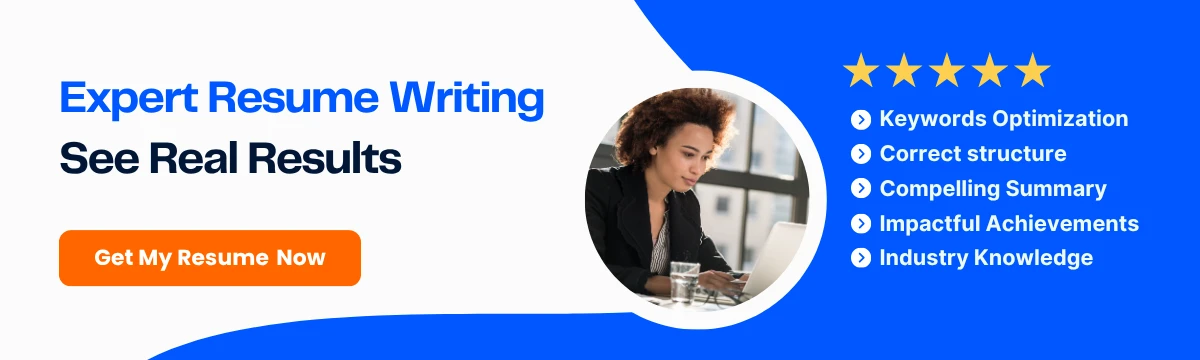
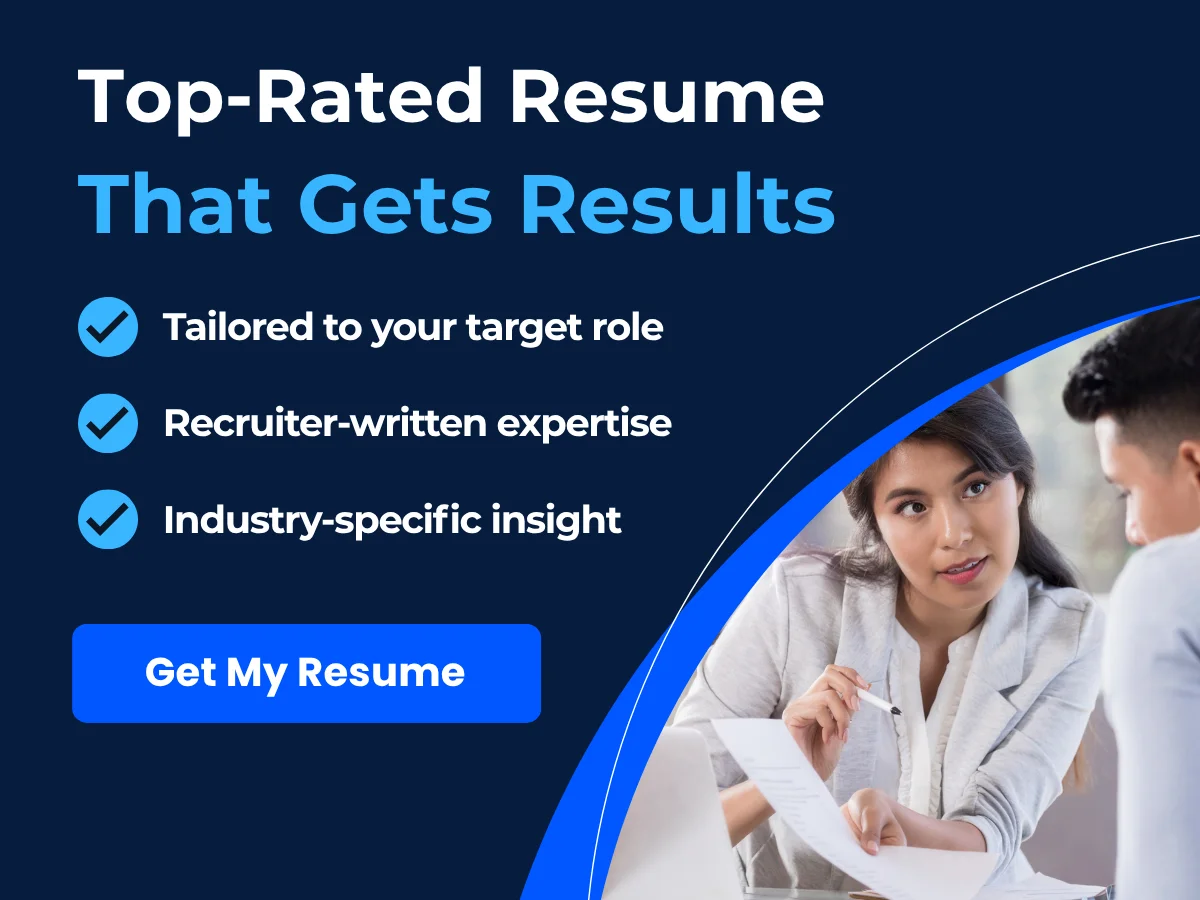
Explain the difference between var
, let
, and const
.
In JavaScript, var
, let
, and const
are used to declare variables, but they have different scopes and behaviors:
var
: This keyword declares a variable that is function-scoped or globally scoped. If a variable is declared withvar
inside a function, it is not accessible outside that function. However, if declared outside any function, it becomes a global variable. Variables declared withvar
can be re-declared and updated.let
: Introduced in ES6 (ECMAScript 2015),let
declares a block-scoped variable. This means that the variable is only accessible within the block (enclosed by curly braces) in which it is defined. Unlikevar
, a variable declared withlet
cannot be re-declared in the same scope, but it can be updated.const
: Also introduced in ES6,const
declares a block-scoped variable that cannot be re-assigned after its initial assignment. However, if the variable holds an object or an array, the contents of that object or array can still be modified. This makesconst
useful for defining constants or references that should not be changed.
Here’s a quick example to illustrate the differences:
function example() {
var x = 1; // function-scoped
let y = 2; // block-scoped
const z = 3; // block-scoped
if (true) {
var x = 10; // same variable, re-declared
let y = 20; // different variable, block-scoped
// z = 30; // Error: Assignment to constant variable
console.log(x); // 10
console.log(y); // 20
}
console.log(x); // 10
console.log(y); // 2
console.log(z); // 3
}
example();
What are JavaScript data types?
JavaScript has several built-in data types that can be categorized into two main groups: primitive types and reference types.
Primitive Data Types
- String: Represents a sequence of characters. Strings are enclosed in single quotes, double quotes, or backticks (for template literals). Example:
let name = "John";
- Number: Represents both integer and floating-point numbers. JavaScript does not differentiate between different types of numbers. Example:
let age = 30;
- Boolean: Represents a logical entity and can have two values:
true
orfalse
. Example:let isActive = true;
- Undefined: A variable that has been declared but has not yet been assigned a value is of type
undefined
. Example:let x;
- Null: Represents the intentional absence of any object value. It is a primitive value that represents “nothing”. Example:
let y = null;
- Symbol: Introduced in ES6, symbols are unique and immutable values that can be used as identifiers for object properties. Example:
let sym = Symbol("description");
- BigInt: A newer addition to JavaScript,
BigInt
allows for the representation of integers larger than253 - 1
. Example:let bigNumber = 1234567890123456789012345678901234567890n;
Reference Data Types
Reference types are more complex and include:
- Object: A collection of key-value pairs. Objects can store multiple values and can be created using object literals or constructors. Example:
let person = { name: "John", age: 30 };
- Array: A special type of object used to store ordered collections of values. Arrays can hold elements of different types. Example:
let fruits = ["apple", "banana", "cherry"];
- Function: Functions in JavaScript are first-class objects, meaning they can be treated like any other object. They can be assigned to variables, passed as arguments, and returned from other functions. Example:
function greet() { return "Hello"; }
Explain type coercion in JavaScript.
Type coercion in JavaScript refers to the automatic or implicit conversion of values from one data type to another. This can happen in various situations, such as when performing operations on different types of values. JavaScript uses two types of coercion: implicit coercion and explicit coercion.
Implicit Coercion
Implicit coercion occurs when JavaScript automatically converts a value to a different type in order to perform an operation. For example:
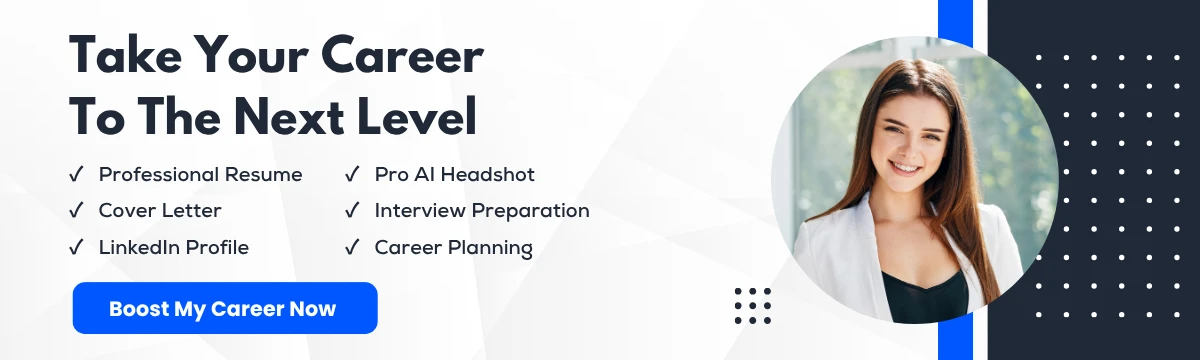
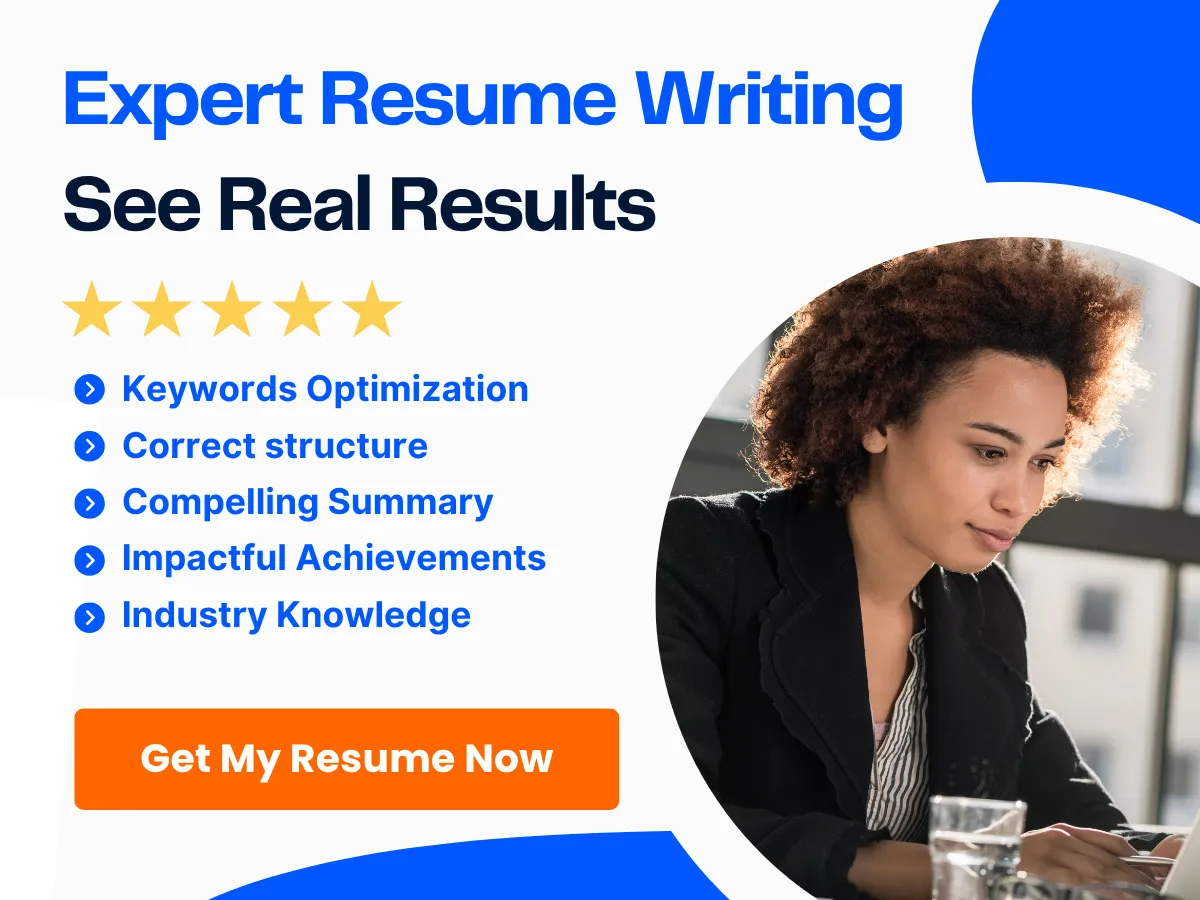
let result = "5" + 1; // "51" (number 1 is coerced to a string)
let sum = "5" - 1; // 4 (string "5" is coerced to a number)
In the first example, the number 1
is converted to a string, resulting in string concatenation. In the second example, the string "5"
is converted to a number, allowing for arithmetic subtraction.
Explicit Coercion
Explicit coercion is when the developer manually converts a value from one type to another using functions or methods. Common methods for explicit coercion include:
String(value)
: Converts a value to a string.Number(value)
: Converts a value to a number.Boolean(value)
: Converts a value to a boolean.
Example of explicit coercion:
let num = "123";
let str = Number(num); // 123 (string to number)
let bool = Boolean(0); // false (0 is falsy)
What is the difference between ==
and ===
?
The difference between ==
(equality operator) and ===
(strict equality operator) lies in how they compare values:
==
(Equality Operator): This operator checks for equality of values but performs type coercion if the values are of different types. For example:
console.log(5 == "5"); // true (string "5" is coerced to number)
console.log(null == undefined); // true (both are considered equal)
===
(Strict Equality Operator): This operator checks for equality of both value and type, meaning no type coercion is performed. For example:console.log(5 === "5"); // false (different types)
console.log(null === undefined); // false (different types)
In general, it is recommended to use ===
to avoid unexpected results due to type coercion, ensuring that both the value and type are the same.
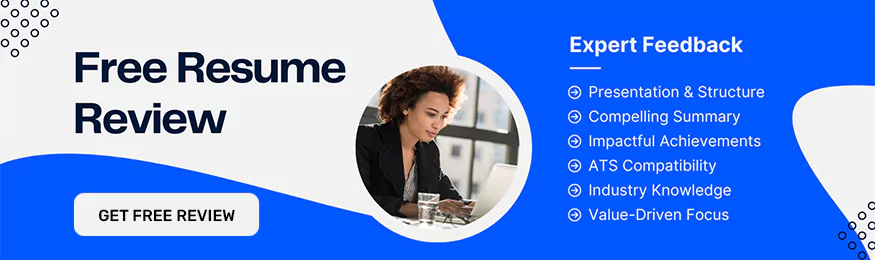
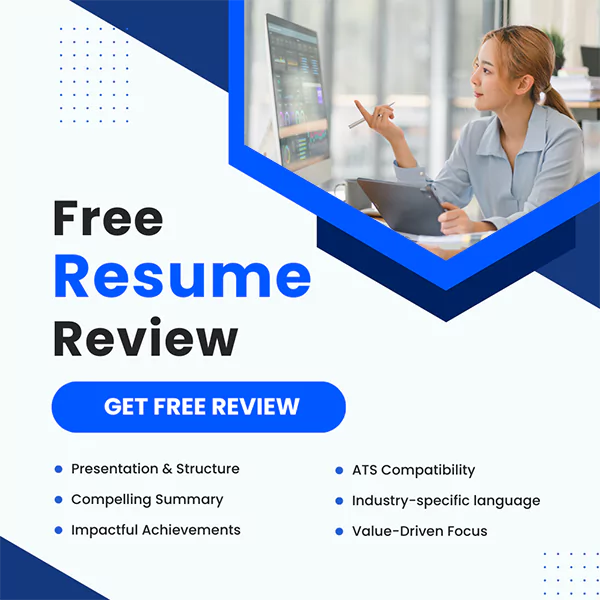
Intermediate JavaScript Questions
What are closures in JavaScript?
Closures are a fundamental concept in JavaScript that allow functions to maintain access to their lexical scope, even when the function is executed outside that scope. In simpler terms, a closure is created when a function is defined inside another function, and the inner function retains access to the variables of the outer function.
To illustrate this, consider the following example:
function outerFunction() {
let outerVariable = 'I am from outer scope';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closureFunction = outerFunction();
closureFunction(); // Output: I am from outer scope
In this example, outerFunction
defines a variable outerVariable
and an inner function innerFunction
. When outerFunction
is called, it returns innerFunction
, which is assigned to closureFunction
. Even though outerFunction
has finished executing, innerFunction
still has access to outerVariable
due to the closure.
Closures are particularly useful for data encapsulation and creating private variables. They are also commonly used in asynchronous programming, where functions need to remember their context when executed later.
Explain the concept of hoisting.
Hoisting is a JavaScript mechanism where variables and function declarations are moved to the top of their containing scope during the compile phase. This means that you can use variables and functions before they are declared in the code. However, it’s important to understand how hoisting works for both variables and functions, as they behave differently.
For variable declarations, only the declaration is hoisted, not the initialization. Consider the following example:
console.log(myVar); // Output: undefined
var myVar = 5;
console.log(myVar); // Output: 5
In this case, the first console.log
outputs undefined
because the declaration of myVar
is hoisted to the top, but its assignment to 5
occurs later in the code.

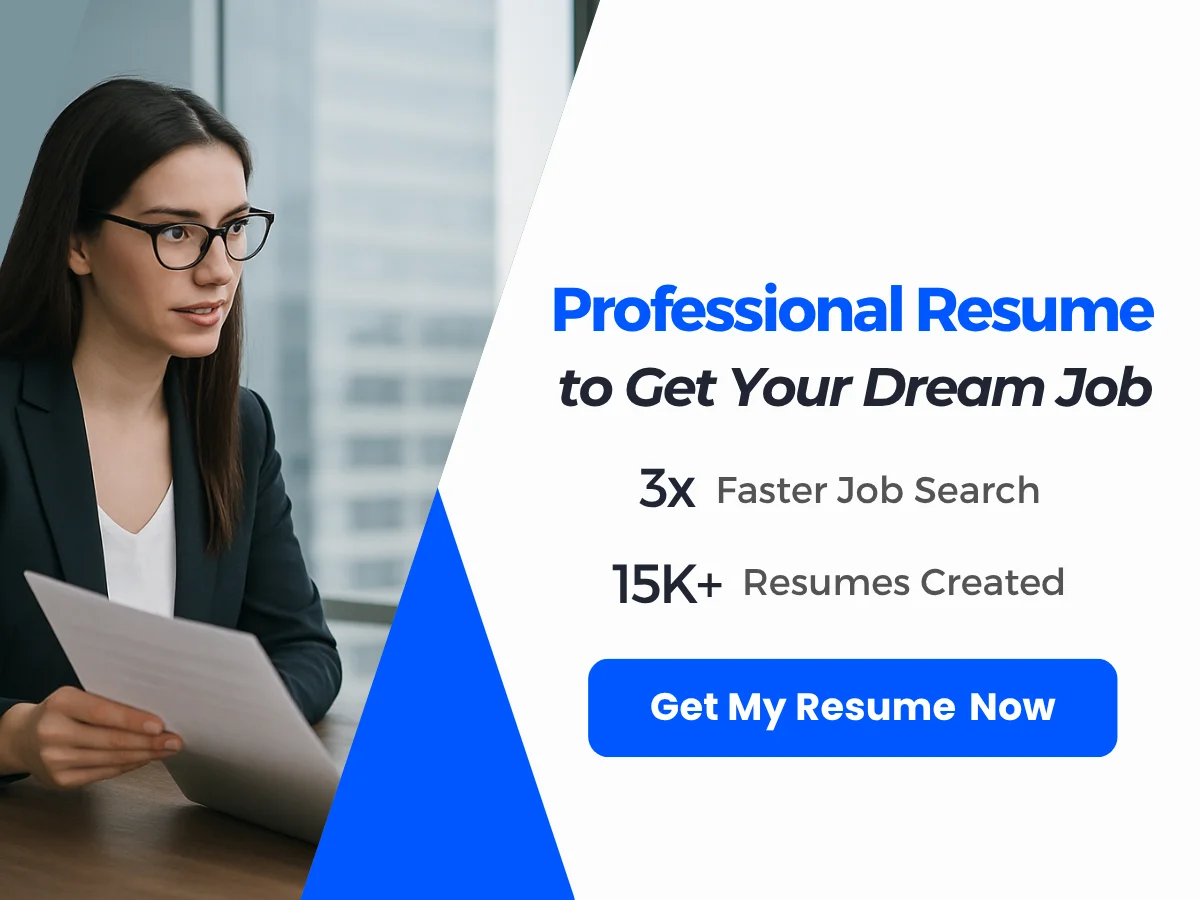
For function declarations, both the declaration and the definition are hoisted:
myFunction(); // Output: "Hello, World!"
function myFunction() {
console.log("Hello, World!");
}
Here, the function myFunction
can be called before its declaration because the entire function is hoisted to the top of the scope.
However, it’s important to note that function expressions (including arrow functions) are not hoisted in the same way:
myFunction(); // TypeError: myFunction is not a function
var myFunction = function() {
console.log("Hello, World!");
};
In this case, attempting to call myFunction
before its assignment results in a TypeError
because only the variable declaration is hoisted, not the function expression.
What is the event loop?
The event loop is a crucial part of JavaScript’s concurrency model, allowing it to perform non-blocking operations despite being single-threaded. It enables JavaScript to handle asynchronous operations, such as user interactions, network requests, and timers, efficiently.
To understand the event loop, it’s essential to know about the call stack, the Web APIs, and the message queue:
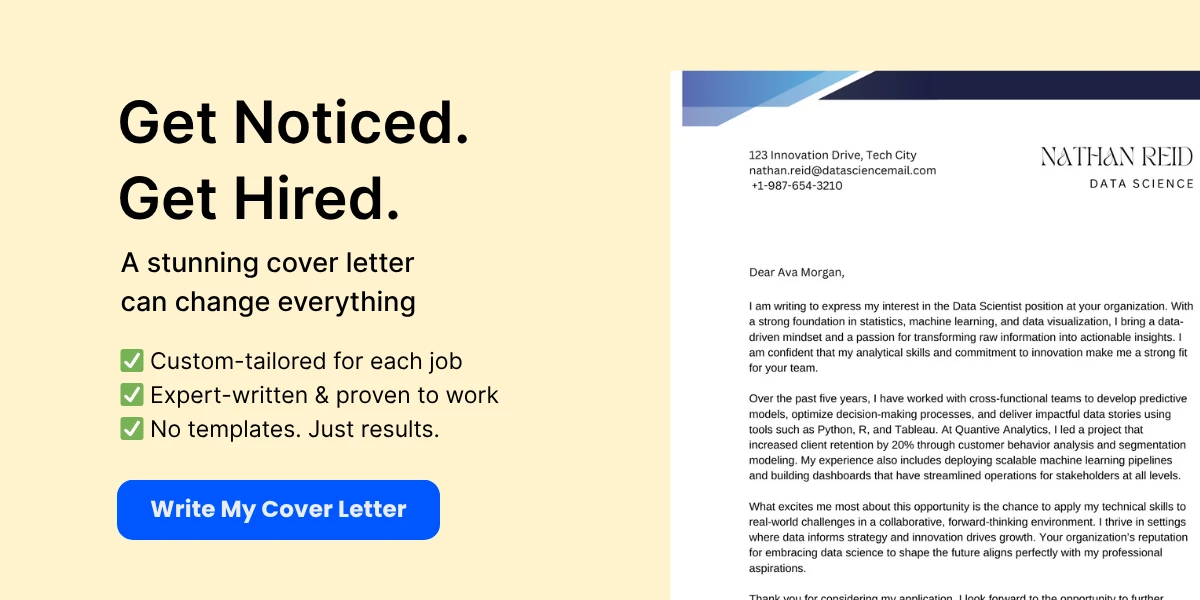
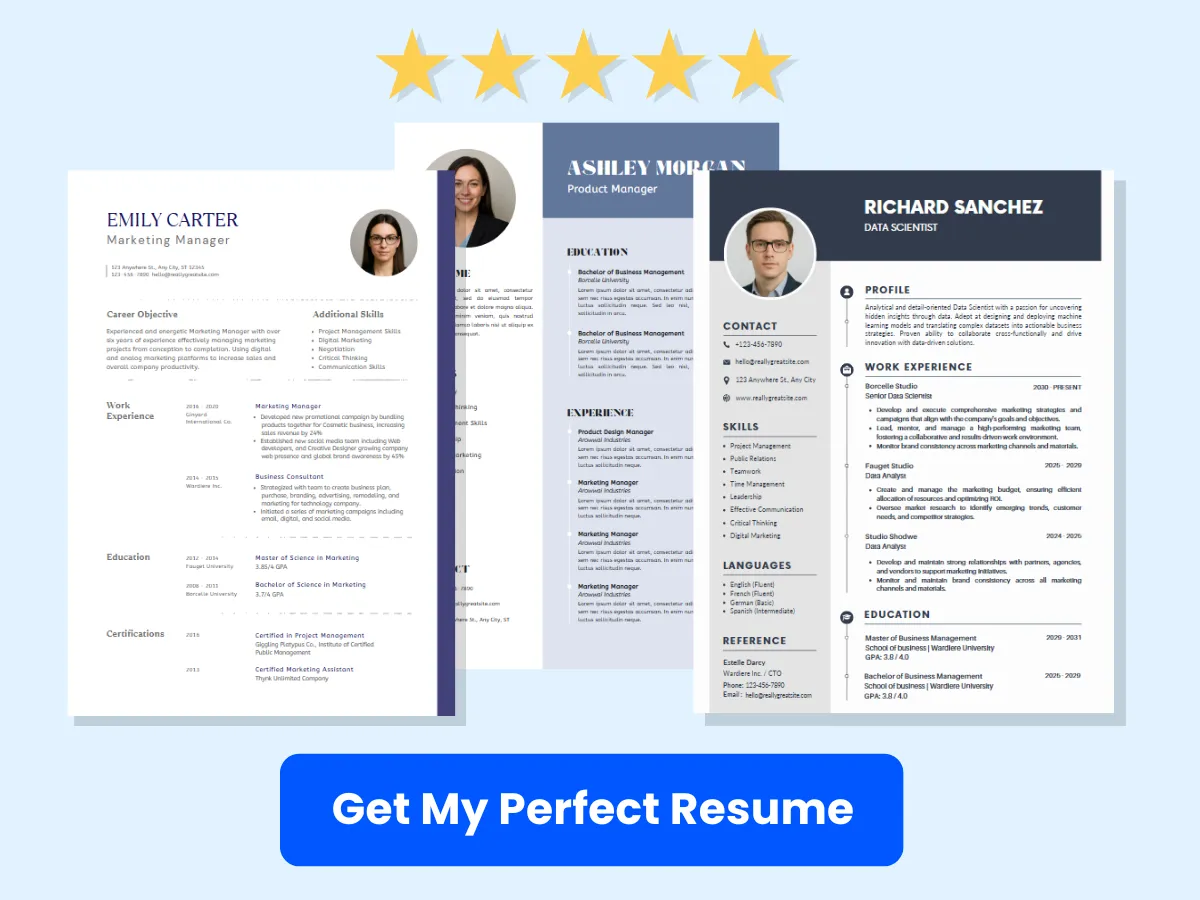
- Call Stack: This is where JavaScript keeps track of function calls. When a function is invoked, it is pushed onto the stack, and when it returns, it is popped off.
- Web APIs: These are provided by the browser (or Node.js) and allow JavaScript to perform asynchronous operations. Examples include
setTimeout
,fetch
, and DOM events. - Message Queue: This is where messages (or callbacks) are queued to be processed after the current execution context is complete.
Here’s a simplified flow of how the event loop works:
- JavaScript executes the code in the call stack.
- When an asynchronous operation is encountered, it is handed off to the Web APIs, and the call stack continues executing the remaining code.
- Once the asynchronous operation is complete, its callback is placed in the message queue.
- When the call stack is empty, the event loop checks the message queue and pushes the first message onto the call stack for execution.
Consider the following example:
console.log("Start");
setTimeout(() => {
console.log("Timeout");
}, 0);
console.log("End");
The output of this code will be:
Start
End
Timeout
In this case, even though the setTimeout
is set to 0
milliseconds, it is still placed in the message queue and will only execute after the current call stack is empty.
How does prototypal inheritance work?
Prototypal inheritance is a core feature of JavaScript that allows objects to inherit properties and methods from other objects. This is different from classical inheritance found in languages like Java or C#. In JavaScript, every object has a prototype, which is another object from which it can inherit properties and methods.
When you try to access a property on an object, JavaScript first checks if the property exists on that object. If it doesn’t, it looks up the prototype chain until it finds the property or reaches the end of the chain (which is null
).
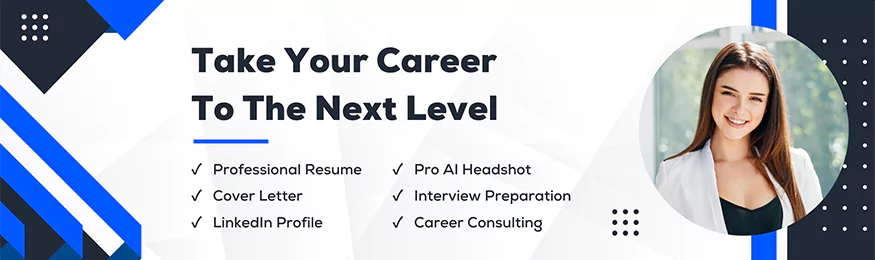
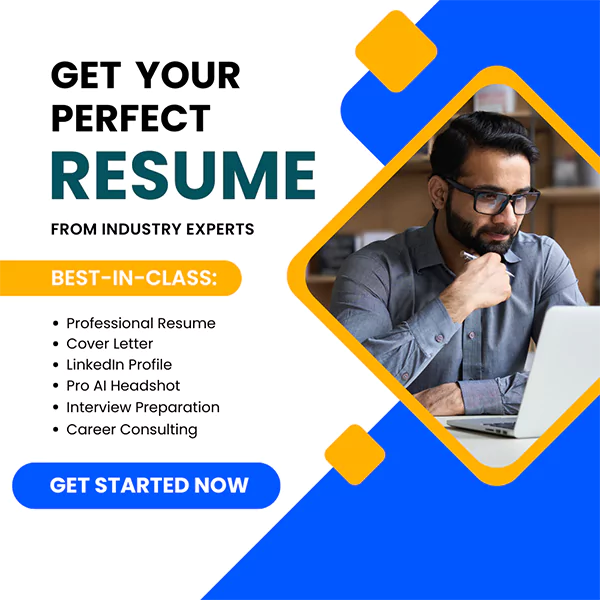
Here’s an example to illustrate prototypal inheritance:
const animal = {
speak: function() {
console.log("Animal speaks");
}
};
const dog = Object.create(animal);
dog.bark = function() {
console.log("Dog barks");
};
dog.speak(); // Output: Animal speaks
dog.bark(); // Output: Dog barks
In this example, we create an animal
object with a speak
method. We then create a dog
object that inherits from animal
using Object.create
. The dog
object has its own bark
method, but it can also access the speak
method from its prototype, animal
.
Prototypal inheritance allows for a more flexible and dynamic way to create objects and share behavior, making it a powerful feature in JavaScript.
What are promises and how do they work?
Promises are a modern way to handle asynchronous operations in JavaScript, providing a cleaner and more manageable approach compared to traditional callback functions. A promise represents a value that may be available now, or in the future, or never. It can be in one of three states:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully, resulting in a resolved value.
- Rejected: The operation failed, resulting in a reason for the failure.
Here’s a simple example of creating and using a promise:
const myPromise = new Promise((resolve, reject) => {
const success = true; // Simulate success or failure
if (success) {
resolve("Operation was successful!");
} else {
reject("Operation failed.");
}
});
myPromise
.then(result => {
console.log(result); // Output: Operation was successful!
})
.catch(error => {
console.error(error);
});
In this example, we create a new promise that simulates an asynchronous operation. If the operation is successful, we call resolve
with a success message; otherwise, we call reject
with an error message. We then use the then
method to handle the fulfilled state and the catch
method to handle the rejected state.
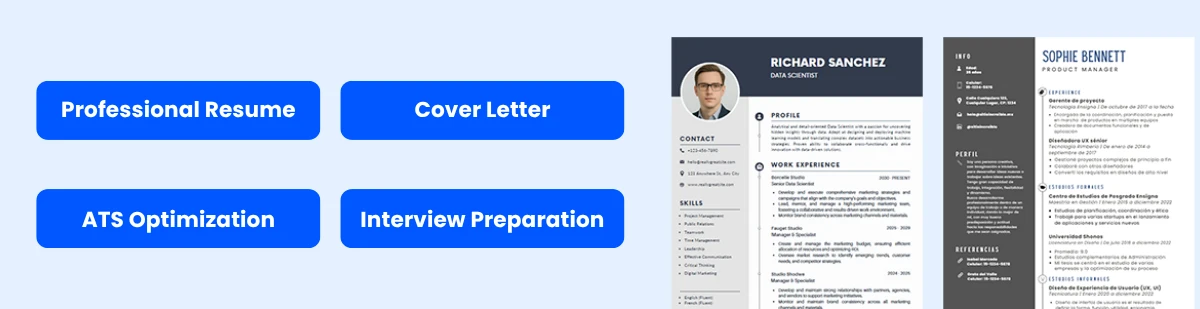
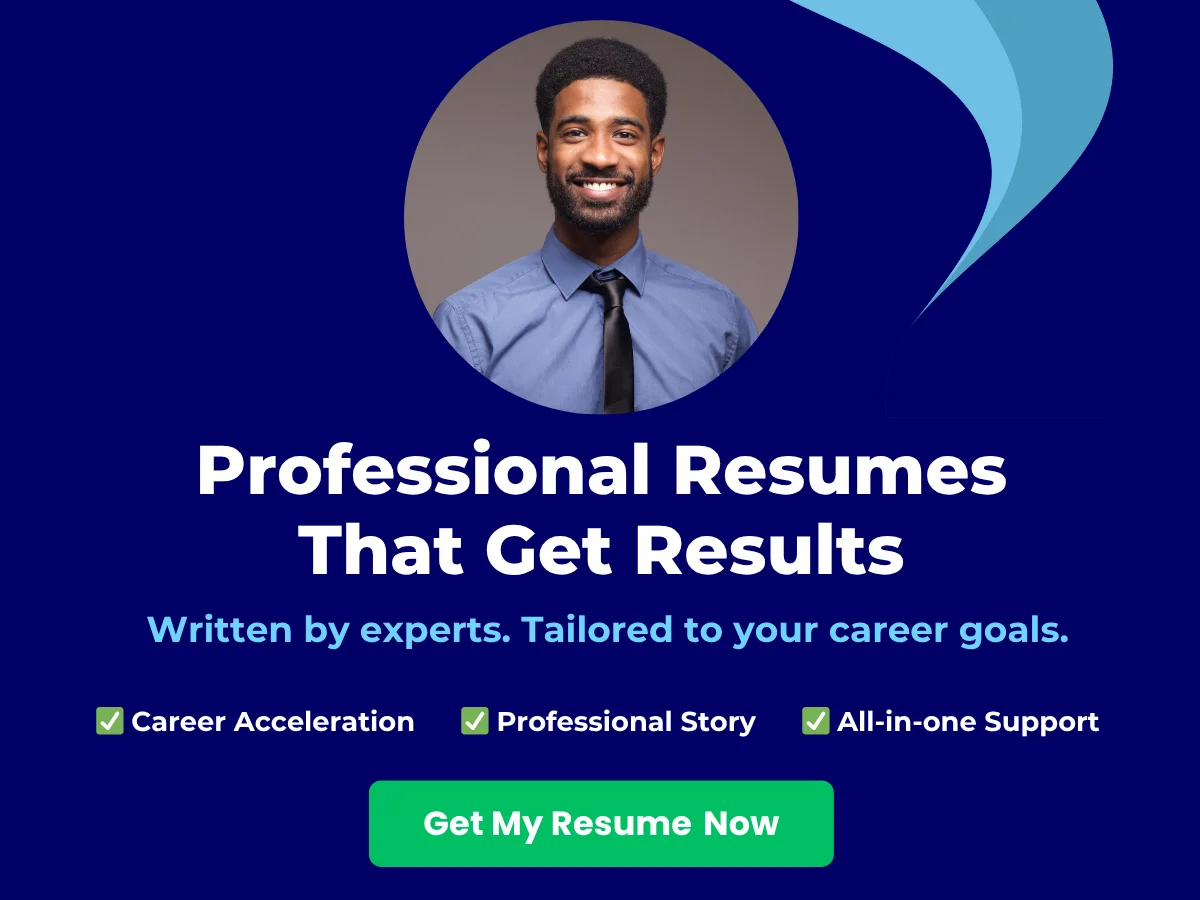
Promises can also be chained, allowing for a sequence of asynchronous operations:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example, we use the fetch
API, which returns a promise. We chain multiple then
calls to handle the response and parse the JSON data, followed by a catch
to handle any errors that may occur during the fetch operation.
Promises are a powerful tool for managing asynchronous code, making it easier to read and maintain, especially when dealing with multiple asynchronous operations.
Advanced JavaScript Questions
What is the difference between call
, apply
, and bind
?
The methods call
, apply
, and bind
are all used to set the this
context of a function in JavaScript, but they do so in different ways.
-
call
The
call
method calls a function with a giventhis
value and arguments provided individually. The syntax is:functionName.call(thisArg, arg1, arg2, ...)
For example:
function greet(greeting) { console.log(greeting + ', ' + this.name); } const person = { name: 'Alice' }; greet.call(person, 'Hello'); // Output: Hello, Alice
-
apply
The
apply
method is similar tocall
, but it takes an array of arguments instead of individual arguments. The syntax is:functionName.apply(thisArg, [argsArray])
For example:
function greet(greeting, punctuation) { console.log(greeting + ', ' + this.name + punctuation); } const person = { name: 'Bob' }; greet.apply(person, ['Hi', '!']); // Output: Hi, Bob!
-
bind
The
bind
method returns a new function, permanently bound to the specifiedthis
value and initial arguments. The syntax is:const newFunction = functionName.bind(thisArg, arg1, arg2, ...)
For example:
function greet() { console.log('Hello, ' + this.name); } const person = { name: 'Charlie' }; const greetCharlie = greet.bind(person); greetCharlie(); // Output: Hello, Charlie
Explain the concept of async/await.
async
and await
are syntactic sugar built on top of Promises, introduced in ES2017 (ES8) to simplify asynchronous programming in JavaScript. They allow developers to write asynchronous code that looks and behaves like synchronous code, making it easier to read and maintain.
Using async
To define an asynchronous function, you prefix the function declaration with the async
keyword. This function will always return a Promise, and if the function returns a value, that value is wrapped in a resolved Promise.
async function fetchData() {
return 'Data fetched';
}
fetchData().then(data => console.log(data)); // Output: Data fetched
Using await
The await
keyword can only be used inside an async
function. It pauses the execution of the function until the Promise is resolved or rejected, allowing for a more linear flow of code.
async function getData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
}
getData();
In this example, the execution of getData
will pause at the await
statements until the Promise returned by fetch
and response.json()
is resolved.
What are generators and how do they work?
Generators are a special class of functions in JavaScript that can be paused and resumed, allowing for the creation of iterators. They are defined using the function*
syntax and use the yield
keyword to yield values.
Creating a Generator
Here’s how you can create a simple generator function:
function* numberGenerator() {
yield 1;
yield 2;
yield 3;
}
const gen = numberGenerator();
console.log(gen.next().value); // Output: 1
console.log(gen.next().value); // Output: 2
console.log(gen.next().value); // Output: 3
Each call to gen.next()
resumes the generator function until the next yield
statement is encountered, returning the yielded value.
Use Cases for Generators
Generators are particularly useful for:
- Implementing iterators for custom data structures.
- Handling asynchronous programming with
yield
in conjunction with Promises. - Creating infinite sequences or streams of data.
What is the module pattern?
The module pattern is a design pattern in JavaScript that allows for encapsulation of private variables and methods while exposing a public API. This pattern is particularly useful for organizing code and avoiding global scope pollution.
Creating a Module
Here’s a simple example of the module pattern:
const CounterModule = (function() {
let count = 0; // private variable
return {
increment: function() {
count++;
console.log(count);
},
decrement: function() {
count--;
console.log(count);
},
getCount: function() {
return count;
}
};
})();
CounterModule.increment(); // Output: 1
CounterModule.increment(); // Output: 2
console.log(CounterModule.getCount()); // Output: 2
In this example, count
is a private variable that cannot be accessed directly from outside the module. The public methods increment
, decrement
, and getCount
provide controlled access to the private variable.
Explain the concept of memoization.
Memoization is an optimization technique used to speed up function calls by caching the results of expensive function calls and returning the cached result when the same inputs occur again. This is particularly useful for functions that are called frequently with the same arguments.
Implementing Memoization
Here’s a simple implementation of a memoization function:
function memoize(fn) {
const cache = {};
return function(...args) {
const key = JSON.stringify(args);
if (cache[key]) {
return cache[key];
}
const result = fn(...args);
cache[key] = result;
return result;
};
}
const factorial = memoize(function(n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
});
console.log(factorial(5)); // Output: 120
console.log(factorial(5)); // Output: 120 (cached result)
In this example, the memoize
function creates a cache object to store results. When the memoized function is called, it checks if the result for the given arguments is already cached. If it is, it returns the cached result; otherwise, it computes the result, stores it in the cache, and then returns it.
JavaScript in the Browser
What is the DOM?
The Document Object Model (DOM) is a programming interface provided by browsers that allows scripts to dynamically access and update the content, structure, and style of a document. In simpler terms, the DOM represents the page so that programs can change the document structure, style, and content. It is an object-oriented representation of the web page, which can be modified with JavaScript.
When a web page is loaded, the browser creates a DOM of the page, which is a tree-like structure where each node represents a part of the document. For example, elements like <div>
, <p>
, and <span>
are all nodes in the DOM tree. The DOM allows developers to interact with these nodes, enabling them to create dynamic web applications.
Here’s a simple example of how the DOM works:
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a paragraph.</p>
</body>
</html>
In this example, the DOM would represent the <html>
element as the root node, with <head>
and <body>
as child nodes, and so on.
How do you manipulate the DOM?
Manipulating the DOM is a core feature of JavaScript, allowing developers to create interactive web pages. There are several methods available to manipulate the DOM, including:
- Selecting Elements: You can select elements using methods like
document.getElementById()
,document.querySelector()
, anddocument.getElementsByClassName()
. - Creating Elements: New elements can be created using
document.createElement()
. - Appending Elements: You can add elements to the DOM using
parentElement.appendChild()
orparentElement.insertBefore()
. - Modifying Elements: You can change the content of an element using
element.innerHTML
orelement.textContent
, and modify attributes usingelement.setAttribute()
. - Removing Elements: Elements can be removed from the DOM using
element.remove()
orparentElement.removeChild()
.
Here’s an example that demonstrates some of these methods:
const newDiv = document.createElement('div');
newDiv.innerHTML = 'This is a new div!';
document.body.appendChild(newDiv);
In this example, a new <div>
element is created and appended to the body of the document.
Explain event delegation.
Event delegation is a technique in JavaScript that allows you to manage events more efficiently by leveraging the event bubbling mechanism. Instead of attaching event listeners to individual elements, you can attach a single event listener to a parent element. This parent element will then handle events triggered by its child elements.
The main advantages of event delegation include:
- Performance: Reduces the number of event listeners, which can improve performance, especially in lists or tables with many items.
- Dynamic Elements: Automatically handles events for dynamically added elements without needing to reattach listeners.
Here’s an example of event delegation:
<ul id="myList">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
In this example, a single click event listener is added to the <ul>
element. When any of the <li>
items are clicked, the event bubbles up to the <ul>
, and the listener checks if the target is an <li>
element before executing the code.
What are web APIs?
Web APIs (Application Programming Interfaces) are interfaces that allow developers to interact with the browser and perform various tasks. They provide a way to access features and functionalities that are not part of the core JavaScript language but are available in the browser environment. Some common web APIs include:
- DOM API: Allows manipulation of the DOM as discussed earlier.
- Fetch API: Provides a modern way to make network requests to servers.
- Canvas API: Enables drawing graphics and animations on a web page.
- Web Storage API: Provides a way to store data in the browser using localStorage and sessionStorage.
- Geolocation API: Allows access to the user's geographical location.
Here’s an example of using the Fetch API to make a network request:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This code snippet fetches data from a specified URL and logs the response to the console. The Fetch API returns a promise, making it easy to handle asynchronous operations.
How do you handle asynchronous operations in the browser?
Handling asynchronous operations in JavaScript is crucial for creating responsive web applications. There are several ways to manage asynchronous code, including:
- Callbacks: Functions that are passed as arguments to other functions and are executed after a certain task is completed. However, callbacks can lead to "callback hell" if not managed properly.
- Promises: Objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises provide a cleaner way to handle asynchronous code compared to callbacks.
- Async/Await: A syntactic sugar built on top of promises that allows you to write asynchronous code in a more synchronous manner. It makes the code easier to read and maintain.
Here’s an example of using async/await to handle asynchronous operations:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
In this example, the fetchData
function is declared as asynchronous using the async
keyword. The await
keyword is used to pause the execution of the function until the promise is resolved, making the code easier to read and understand.
JavaScript Frameworks and Libraries
What is React and how does it work?
React is a popular JavaScript library developed by Facebook for building user interfaces, particularly for single-page applications. It allows developers to create large web applications that can change data without reloading the page. The core philosophy of React is to build encapsulated components that manage their own state, then compose them to make complex UIs.
At its core, React uses a declarative approach, meaning developers describe what the UI should look like for any given state, and React takes care of updating the DOM when the state changes. This is achieved through a virtual DOM, which is a lightweight copy of the actual DOM. When a component's state changes, React first updates the virtual DOM, then compares it with the previous version, and finally updates only the parts of the actual DOM that have changed. This process, known as reconciliation, enhances performance and provides a smoother user experience.
Key Features of React:
- Component-Based Architecture: React encourages the development of reusable UI components, which can be nested and managed independently.
- JSX: React uses JSX, a syntax extension that allows mixing HTML with JavaScript, making it easier to write and visualize the UI structure.
- State Management: React components can maintain their own state, allowing for dynamic and interactive applications.
- Lifecycle Methods: React provides lifecycle methods that allow developers to hook into different stages of a component's life, such as mounting, updating, and unmounting.
Explain the core concepts of Angular.
Angular is a platform and framework for building client-side applications using HTML, CSS, and TypeScript. Developed by Google, Angular is designed to make the development and testing of such applications easier. It follows the MVC (Model-View-Controller) architecture, which separates the application logic from the user interface.
Some of the core concepts of Angular include:
1. Components:
Components are the building blocks of Angular applications. Each component consists of an HTML template, a TypeScript class, and associated styles. Components encapsulate the logic and view of a part of the application, promoting reusability and maintainability.
2. Modules:
Angular applications are modular, meaning they are divided into modules that group related components, directives, pipes, and services. The root module, typically named AppModule
, bootstraps the application.
3. Services and Dependency Injection:
Services are classes that provide specific functionality, such as data fetching or business logic. Angular uses dependency injection to manage service instances, making it easier to share services across components and improve testability.
4. Directives:
Directives are special markers in the DOM that tell Angular to attach a specified behavior to that DOM element or even transform the DOM element and its children. There are three types of directives: components, structural directives (like *ngIf
and *ngFor
), and attribute directives.
5. Routing:
Angular provides a powerful routing module that allows developers to define navigation paths in their applications. This enables the creation of single-page applications where users can navigate between different views without reloading the page.
What is Vue.js and why use it?
Vue.js is a progressive JavaScript framework used for building user interfaces. Unlike other monolithic frameworks, Vue is designed to be incrementally adoptable. This means that you can use it to enhance existing applications or build new ones from scratch.
Vue.js is known for its simplicity and flexibility. It combines the best features of Angular and React, offering a reactive data-binding system and a component-based architecture. Here are some reasons why developers choose Vue.js:
- Easy to Learn: Vue's syntax is straightforward, making it accessible for beginners while still powerful enough for experienced developers.
- Reactive Data Binding: Vue's reactivity system allows for automatic updates to the DOM when the underlying data changes, simplifying the development process.
- Single-File Components: Vue allows developers to encapsulate HTML, CSS, and JavaScript in a single file, promoting better organization and maintainability.
- Rich Ecosystem: Vue has a vibrant ecosystem with a variety of libraries and tools, such as Vue Router for routing and Vuex for state management.
How does jQuery simplify JavaScript?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. It was created to make it easier to work with JavaScript, especially in the context of cross-browser compatibility.
Here are some ways jQuery simplifies JavaScript:
- DOM Manipulation: jQuery provides a simple syntax for selecting and manipulating DOM elements. For example, instead of using
document.getElementById
, you can simply use$('#elementId')
. - Event Handling: jQuery streamlines event handling with methods like
.on()
, allowing developers to attach event handlers easily without worrying about browser inconsistencies. - Ajax Support: jQuery simplifies Ajax calls with methods like
$.ajax()
, making it easier to load data asynchronously without writing complex code. - Animations: jQuery provides built-in methods for creating animations and effects, such as
.fadeIn()
and.slideUp()
, which can enhance user experience without requiring extensive knowledge of CSS animations.
What are some popular JavaScript testing frameworks?
Testing is a crucial part of the software development process, and JavaScript has several frameworks that facilitate testing. Here are some of the most popular JavaScript testing frameworks:
- Jest: Developed by Facebook, Jest is a zero-config, all-in-one testing framework that works well with React applications. It supports snapshot testing, mocking, and provides a rich API for writing tests.
- Mocha: Mocha is a flexible testing framework that allows developers to choose their assertion library (like Chai) and provides a variety of testing styles, including BDD (Behavior-Driven Development) and TDD (Test-Driven Development).
- Jasmine: Jasmine is a behavior-driven testing framework that does not require a DOM and can be used for testing any JavaScript code. It provides a clean syntax for writing tests and includes built-in matchers.
- QUnit: QUnit is a powerful, easy-to-use JavaScript unit testing framework that is particularly well-suited for testing jQuery code. It provides a simple API for writing tests and supports asynchronous testing.
- Cypress: Cypress is an end-to-end testing framework that allows developers to write tests that run in the browser. It provides a rich set of features for testing web applications, including time travel debugging and automatic waiting.
Understanding JavaScript frameworks and libraries is essential for modern web development. Each framework and library has its unique features and advantages, making them suitable for different types of projects. By mastering these tools, developers can create efficient, maintainable, and scalable applications.
JavaScript Performance Optimization
How do you optimize JavaScript code?
Optimizing JavaScript code is crucial for enhancing the performance of web applications. Here are several strategies to consider:
- Minification: This process involves removing unnecessary characters from the code, such as whitespace, comments, and line breaks, without changing its functionality. Tools like UglifyJS and Terser can automate this process.
- Bundling: Instead of loading multiple JavaScript files, bundling combines them into a single file. This reduces the number of HTTP requests, which can significantly improve load times. Webpack and Rollup are popular tools for bundling.
- Code Splitting: This technique allows you to split your code into smaller chunks that can be loaded on demand. This is particularly useful for large applications, as it ensures that only the necessary code is loaded initially, improving the initial load time.
- Using Efficient Algorithms: Always choose the right algorithm for the task. For example, using a hash table for lookups can be more efficient than using an array, especially for large datasets.
- Debouncing and Throttling: These techniques help manage the rate at which a function is executed. Debouncing ensures that a function is only called after a certain period of inactivity, while throttling limits the number of times a function can be called over time. This is particularly useful for events like scrolling or resizing.
- Asynchronous Programming: Utilize asynchronous programming patterns, such as Promises and async/await, to prevent blocking the main thread. This allows the browser to remain responsive while executing long-running tasks.
What are some common performance pitfalls?
When optimizing JavaScript performance, it's essential to be aware of common pitfalls that can hinder your efforts:
- Excessive DOM Manipulation: Frequent changes to the DOM can be costly in terms of performance. Instead of making multiple changes, batch your updates or use Document Fragments to minimize reflows and repaints.
- Memory Leaks: Memory leaks occur when memory that is no longer needed is not released. This can lead to increased memory usage and degraded performance over time. Common causes include global variables, forgotten timers, and event listeners that are not properly removed.
- Blocking the Main Thread: Long-running JavaScript tasks can block the main thread, causing the UI to become unresponsive. Use Web Workers for heavy computations to keep the main thread free.
- Overusing Libraries: While libraries can simplify development, over-reliance on them can lead to bloated code. Evaluate whether a library is necessary for your project or if native JavaScript can achieve the same results.
- Not Using Caching: Failing to cache data can lead to unnecessary network requests. Utilize browser caching and service workers to store frequently accessed data locally.
Explain lazy loading.
Lazy loading is a design pattern that postpones the loading of non-essential resources at the point of page load. Instead, these resources are loaded only when they are needed, such as when they enter the viewport. This technique can significantly improve the performance of web applications, especially those with large images or complex components.
For example, consider an image gallery. Instead of loading all images at once, you can implement lazy loading to load images only when the user scrolls down to them. This reduces the initial load time and saves bandwidth. Here’s a simple implementation using the Intersection Observer API:
const images = document.querySelectorAll('img[data-src]');
const imgObserver = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src; // Set the src attribute to the data-src value
imgObserver.unobserve(img); // Stop observing the image
}
});
});
images.forEach(image => {
imgObserver.observe(image); // Start observing each image
});
This code snippet sets up an observer that watches for images entering the viewport. When an image is about to be displayed, it sets the src
attribute to the value stored in data-src
, effectively loading the image only when necessary.
How do you handle memory leaks in JavaScript?
Memory leaks in JavaScript can lead to performance degradation and unresponsive applications. Here are some strategies to identify and handle memory leaks:
- Use the Chrome DevTools: The Memory panel in Chrome DevTools allows you to take heap snapshots and analyze memory usage. Look for detached DOM trees, which occur when DOM elements are removed but still referenced in JavaScript.
- Remove Event Listeners: Always ensure that event listeners are removed when they are no longer needed. This is especially important for elements that are dynamically created and destroyed.
- Clear Timers: If you use
setInterval
orsetTimeout
, make sure to clear them when they are no longer needed. Failing to do so can keep references to functions and variables, preventing garbage collection. - Weak References: Use
WeakMap
andWeakSet
for storing references to objects that can be garbage collected when there are no other references to them. This helps prevent memory leaks in certain scenarios. - Profiling: Regularly profile your application to monitor memory usage over time. Look for patterns that indicate memory is not being released, and investigate the root causes.
What are web workers and how do they improve performance?
Web Workers are a feature in JavaScript that allows you to run scripts in background threads, separate from the main execution thread of a web application. This means that long-running tasks can be executed without blocking the user interface, leading to a smoother user experience.
Web Workers are particularly useful for computationally intensive tasks, such as data processing, image manipulation, or any operation that would otherwise cause the UI to freeze. Here’s a simple example of how to create and use a Web Worker:
// main.js
const worker = new Worker('worker.js');
worker.onmessage = function(event) {
console.log('Result from worker:', event.data);
};
worker.postMessage('Start processing data');
// worker.js
onmessage = function(event) {
// Simulate a heavy computation
let result = 0;
for (let i = 0; i < 1e9; i++) {
result += i;
}
postMessage(result); // Send the result back to the main thread
};
In this example, the main script creates a new Web Worker that runs the code in worker.js
. The worker performs a heavy computation and sends the result back to the main thread using postMessage
. This allows the main thread to remain responsive while the worker handles the computation.
Optimizing JavaScript performance involves a combination of strategies, awareness of common pitfalls, and leveraging features like lazy loading and Web Workers. By implementing these techniques, developers can create faster, more efficient web applications that provide a better user experience.
JavaScript Best Practices
What are some coding standards for JavaScript?
Coding standards are essential for maintaining consistency and quality in JavaScript code. They help developers understand each other's code, reduce bugs, and improve collaboration. Here are some widely accepted coding standards and practices:
- Use of Semicolons: Always use semicolons to terminate statements. While JavaScript has automatic semicolon insertion, relying on it can lead to unexpected behavior.
- Consistent Naming Conventions: Use camelCase for variables and functions (e.g.,
myVariable
,calculateTotal
), and PascalCase for classes (e.g.,MyClass
). This helps in distinguishing between different types of identifiers. - Indentation and Spacing: Use consistent indentation (2 or 4 spaces) and spacing around operators and keywords to enhance readability. For example:
if (condition) {
doSomething();
}
- Commenting: Write meaningful comments to explain complex logic or important decisions. Avoid obvious comments that do not add value.
- Use of 'const' and 'let': Prefer
const
for variables that do not change andlet
for those that do. Avoid usingvar
to prevent scope-related issues. - Modular Code: Break your code into smaller, reusable functions or modules. This not only improves readability but also makes testing easier.
Explain the importance of code readability.
Code readability is crucial for several reasons:
- Collaboration: In a team environment, multiple developers may work on the same codebase. Readable code allows team members to quickly understand each other's work, facilitating collaboration and reducing onboarding time for new developers.
- Maintenance: Code is often maintained long after it is written. Readable code makes it easier to identify bugs, implement new features, and refactor existing code without introducing new issues.
- Debugging: When debugging, readable code allows developers to follow the logic more easily, making it simpler to spot errors or unexpected behavior.
- Documentation: Readable code serves as its own documentation. When code is clear and well-structured, it reduces the need for extensive external documentation.
To enhance code readability, developers should focus on clear naming conventions, consistent formatting, and logical organization of code. Tools like ESLint and Prettier can help enforce coding standards and improve overall code quality.
How do you handle errors in JavaScript?
Error handling is a critical aspect of JavaScript programming. Proper error handling ensures that applications can gracefully recover from unexpected situations. Here are some strategies for handling errors in JavaScript:
- Try-Catch Blocks: Use
try
andcatch
statements to handle exceptions. This allows you to catch errors and execute alternative code without crashing the application. For example:
try {
// Code that may throw an error
let result = riskyFunction();
} catch (error) {
console.error("An error occurred:", error);
}
- Throwing Errors: You can throw custom errors using the
throw
statement. This is useful for validating input or enforcing business rules:
function validateInput(input) {
if (!input) {
throw new Error("Input cannot be empty");
}
}
- Using Promises: When working with asynchronous code, use
Promise
methods like.catch()
to handle errors. This keeps your code clean and manageable:
fetch('https://api.example.com/data')
.then(response => response.json())
.catch(error => console.error("Fetch error:", error));
- Global Error Handling: For unhandled errors, consider using
window.onerror
orprocess.on('uncaughtException')
in Node.js to log errors and prevent application crashes.
What are some security best practices?
Security is a paramount concern in web development. Here are some best practices to secure your JavaScript applications:
- Input Validation: Always validate and sanitize user input to prevent injection attacks, such as SQL injection or Cross-Site Scripting (XSS). Use libraries like DOMPurify to sanitize HTML input.
- Use HTTPS: Ensure that your application is served over HTTPS to encrypt data in transit and protect against man-in-the-middle attacks.
- Content Security Policy (CSP): Implement a CSP to restrict the sources from which content can be loaded. This helps mitigate XSS attacks by preventing the execution of malicious scripts.
- Secure Cookies: Use the
HttpOnly
andSecure
flags for cookies to prevent access via JavaScript and ensure they are only sent over HTTPS. - Limit Exposure of Sensitive Data: Avoid exposing sensitive information in your JavaScript code. Use environment variables for configuration and keep secrets out of the client-side code.
How do you write maintainable JavaScript code?
Writing maintainable JavaScript code is essential for long-term project success. Here are some strategies to achieve this:
- Follow Coding Standards: Adhere to established coding standards and conventions to ensure consistency across the codebase.
- Use Version Control: Utilize version control systems like Git to track changes, collaborate with others, and manage code history effectively.
- Write Unit Tests: Implement unit tests to verify the functionality of your code. This helps catch bugs early and ensures that changes do not break existing functionality.
- Refactor Regularly: Regularly review and refactor your code to improve its structure and readability. This helps prevent technical debt from accumulating over time.
- Document Your Code: Write clear documentation for your code, including function descriptions, parameters, and return values. This aids other developers in understanding your code and its intended use.
- Use Modern JavaScript Features: Leverage modern JavaScript features (ES6+) such as arrow functions, destructuring, and template literals to write cleaner and more concise code.
By following these best practices, developers can create JavaScript applications that are not only functional but also easy to read, maintain, and scale over time.
JavaScript Tools and Environments
What is Node.js and how is it used?
Node.js is an open-source, cross-platform runtime environment that allows developers to execute JavaScript code server-side. Built on the V8 JavaScript engine developed by Google, Node.js enables the creation of scalable network applications. It uses an event-driven, non-blocking I/O model, which makes it efficient and suitable for data-intensive real-time applications.
Node.js is primarily used for building server-side applications, RESTful APIs, and microservices. It allows developers to use JavaScript for both client-side and server-side scripting, which streamlines the development process and reduces the context switching between different programming languages.
Here’s a simple example of a Node.js application:
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello Worldn');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
In this example, we create a basic HTTP server that listens on port 3000 and responds with "Hello World" to any incoming requests. This demonstrates how Node.js can be used to handle server-side logic with JavaScript.
Explain the role of npm in JavaScript development.
npm, short for Node Package Manager, is the default package manager for Node.js. It plays a crucial role in JavaScript development by allowing developers to install, share, and manage dependencies for their projects. With npm, developers can easily access a vast repository of open-source libraries and tools, which can significantly speed up the development process.
Some key features of npm include:
- Package Management: npm allows developers to install packages (libraries or tools) from the npm registry using simple commands. For example, to install the popular Express framework, you would run
npm install express
. - Version Control: npm helps manage package versions, ensuring that projects use the correct versions of dependencies. This is crucial for maintaining compatibility and avoiding breaking changes.
- Scripts: npm enables developers to define scripts in the
package.json
file, which can automate tasks such as testing, building, and deploying applications. For example, you can define a test script like this:
"scripts": {
"test": "mocha"
}
Then, you can run the test script using npm test
.
What are build tools like Webpack and Gulp?
Build tools are essential in modern JavaScript development as they automate tasks and optimize the workflow. Two popular build tools are Webpack and Gulp.
Webpack
Webpack is a module bundler that takes modules with dependencies and generates static assets representing those modules. It allows developers to bundle JavaScript files for usage in a browser, but it can also transform, bundle, or package any resource or asset, such as HTML, CSS, and images.
Webpack uses a configuration file (usually webpack.config.js
) to define how the application should be built. Here’s a simple example:
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist')
},
module: {
rules: [
{
test: /.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
};
This configuration specifies the entry point of the application, the output file, and how to handle JavaScript files using Babel for transpilation.
Gulp
Gulp is a task runner that automates repetitive tasks in the development workflow. It uses a code-over-configuration approach, allowing developers to write tasks in JavaScript. Gulp is particularly useful for tasks like minification, compilation, unit testing, and linting.
Here’s a simple Gulp setup:
const gulp = require('gulp');
const concat = require('gulp-concat');
const uglify = require('gulp-uglify');
gulp.task('scripts', function() {
return gulp.src('src/*.js')
.pipe(concat('all.js'))
.pipe(uglify())
.pipe(gulp.dest('dist'));
});
In this example, the scripts
task concatenates all JavaScript files in the src
directory, minifies them, and outputs the result to the dist
directory.
How do you use Babel in a JavaScript project?
Babel is a JavaScript compiler that allows developers to use the latest JavaScript features without worrying about browser compatibility. It transforms modern JavaScript (ES6+) into a version that can run in older browsers.
To use Babel in a project, you need to install it along with the necessary presets and plugins. Here’s how to set it up:
npm install --save-dev @babel/core @babel/cli @babel/preset-env
Next, create a Babel configuration file named .babelrc
in the root of your project:
{
"presets": ["@babel/preset-env"]
}
Now, you can use Babel to transpile your JavaScript files. For example, to transpile files in the src
directory and output them to the dist
directory, you can run:
npx babel src --out-dir dist
This command will convert all JavaScript files in the src
directory to a compatible version and place them in the dist
directory.
What are some popular JavaScript IDEs and editors?
Choosing the right Integrated Development Environment (IDE) or code editor can significantly enhance productivity and streamline the development process. Here are some popular JavaScript IDEs and editors:
- Visual Studio Code: A free, open-source code editor developed by Microsoft. It offers a rich ecosystem of extensions, integrated Git control, syntax highlighting, IntelliSense, and debugging capabilities, making it a favorite among JavaScript developers.
- WebStorm: A powerful commercial IDE from JetBrains specifically designed for JavaScript development. It provides advanced features like code completion, refactoring, and debugging tools, along with support for modern frameworks like React, Angular, and Vue.js.
- Sublime Text: A lightweight and fast text editor known for its speed and simplicity. It supports various programming languages and offers a wide range of plugins to enhance functionality.
- Atom: An open-source text editor developed by GitHub. It is highly customizable and has a built-in package manager, allowing developers to install themes and plugins easily.
- Brackets: A lightweight, yet powerful, modern text editor that is specifically designed for web development. It features a live preview, preprocessor support, and a strong focus on front-end development.
Each of these tools has its strengths and weaknesses, and the choice often depends on personal preference and project requirements. However, they all provide essential features that can help streamline JavaScript development and improve overall efficiency.
JavaScript Scenarios
How do you handle cross-browser compatibility?
Cross-browser compatibility refers to the ability of a website or web application to function correctly across different web browsers. Given the variety of browsers available, each with its own rendering engine and JavaScript engine, ensuring that your JavaScript code works seamlessly across all of them can be challenging.
To handle cross-browser compatibility, developers can follow several best practices:
- Use Feature Detection: Instead of checking for specific browsers, use feature detection libraries like Modernizr. This allows you to determine if a browser supports a particular feature and adjust your code accordingly.
- Polyfills: For features not supported in older browsers, consider using polyfills. A polyfill is a piece of code that provides the functionality of a newer feature in older browsers. For example, you can use
Array.prototype.includes
in older browsers by including a polyfill. - CSS Resets: Different browsers have different default styles. Using a CSS reset or a normalize stylesheet can help ensure a consistent look across browsers.
- Testing: Regularly test your application in multiple browsers and devices. Tools like BrowserStack or CrossBrowserTesting can help automate this process.
- Graceful Degradation and Progressive Enhancement: Design your application to work in older browsers while enhancing the experience for users with modern browsers. This approach ensures that all users can access your content, regardless of their browser capabilities.
Explain the concept of single-page applications (SPAs).
A Single-Page Application (SPA) is a web application that interacts with the user by dynamically rewriting the current page, rather than loading entire new pages from the server. This approach provides a more fluid and responsive user experience, similar to that of a desktop application.
Key characteristics of SPAs include:
- Dynamic Content Loading: SPAs load content dynamically using AJAX requests, which allows for faster interactions without the need for full page reloads.
- Client-Side Routing: SPAs often use client-side routing libraries (like React Router or Vue Router) to manage navigation within the application without triggering a full page refresh.
- State Management: SPAs typically require a robust state management solution (like Redux or Vuex) to handle the application state across various components.
- Improved Performance: By loading only the necessary resources and data, SPAs can significantly improve performance and reduce server load.
Popular frameworks for building SPAs include React, Vue.js, and Angular. These frameworks provide tools and libraries that simplify the development of SPAs, making it easier to manage components, state, and routing.
How do you implement authentication in a JavaScript application?
Implementing authentication in a JavaScript application is crucial for securing user data and ensuring that only authorized users can access certain features. There are several methods to implement authentication, and the choice often depends on the specific requirements of the application.
Here are common approaches to authentication in JavaScript applications:
- Token-Based Authentication: This is a popular method where the server generates a token (usually a JSON Web Token, or JWT) upon successful login. The client stores this token (in local storage or cookies) and includes it in the headers of subsequent requests to authenticate the user. Libraries like jsonwebtoken can be used to create and verify tokens.
- Session-Based Authentication: In this approach, the server creates a session for the user after login and stores the session ID in a cookie. The client sends this cookie with each request, allowing the server to identify the user. This method is often used in traditional web applications.
- OAuth: For applications that require third-party authentication (like logging in with Google or Facebook), OAuth is a widely used protocol. It allows users to authenticate using their existing accounts on other platforms, simplifying the login process.
Regardless of the method chosen, it is essential to implement secure practices, such as:
- Using HTTPS to encrypt data in transit.
- Implementing proper session expiration and renewal mechanisms.
- Validating user input to prevent attacks like SQL injection and XSS.
What are some common use cases for JavaScript in web development?
JavaScript is a versatile language that plays a crucial role in modern web development. Here are some common use cases:
- Dynamic Content Updates: JavaScript allows developers to update the content of a web page dynamically without requiring a full page reload. This is commonly used in applications like news feeds, social media platforms, and e-commerce sites.
- Form Validation: JavaScript can be used to validate user input in forms before submission, providing immediate feedback and improving user experience.
- Interactive User Interfaces: JavaScript frameworks and libraries (like React, Vue.js, and Angular) enable developers to create rich, interactive user interfaces that respond to user actions in real-time.
- Animations and Effects: JavaScript can be used to create animations and visual effects, enhancing the overall user experience. Libraries like GSAP and Anime.js are popular for this purpose.
- API Interactions: JavaScript is commonly used to make asynchronous requests to APIs, allowing applications to fetch and display data from external sources without reloading the page.
How do you integrate JavaScript with backend technologies?
Integrating JavaScript with backend technologies is essential for building full-stack applications. Here are some common methods and technologies used for this integration:
- RESTful APIs: One of the most common ways to integrate JavaScript with backend technologies is through RESTful APIs. The backend (built with technologies like Node.js, Express, Django, or Ruby on Rails) exposes endpoints that the JavaScript frontend can call using AJAX or Fetch API to retrieve or send data.
- GraphQL: An alternative to REST, GraphQL allows clients to request only the data they need. This can lead to more efficient data fetching and a better developer experience. Libraries like Apollo Client can be used to integrate GraphQL with JavaScript applications.
- WebSockets: For real-time applications (like chat apps or live notifications), WebSockets provide a way to establish a persistent connection between the client and server. This allows for bi-directional communication, enabling instant data updates.
- Server-Side Rendering (SSR): Frameworks like Next.js and Nuxt.js allow developers to render JavaScript applications on the server side, improving performance and SEO. This approach integrates JavaScript with backend technologies by generating HTML on the server before sending it to the client.
Integrating JavaScript with backend technologies involves using APIs, real-time communication protocols, and server-side rendering techniques to create seamless and efficient web applications.
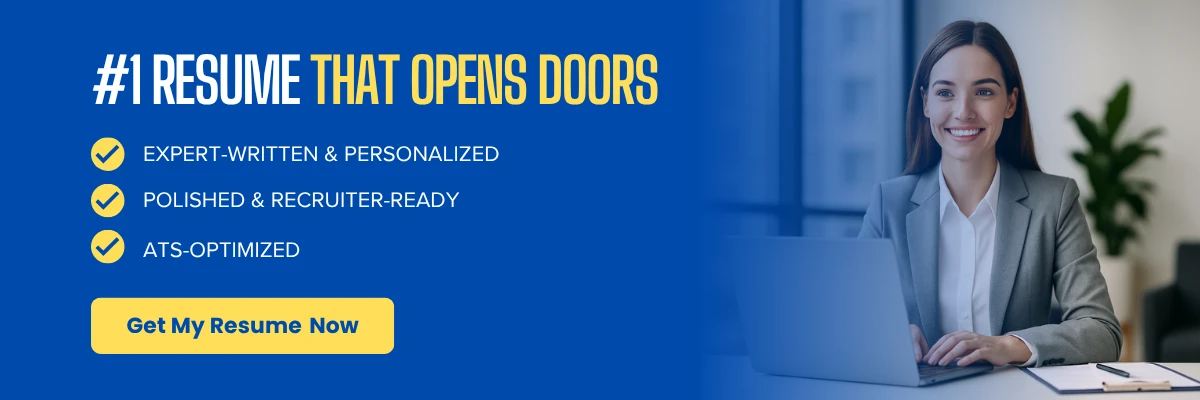
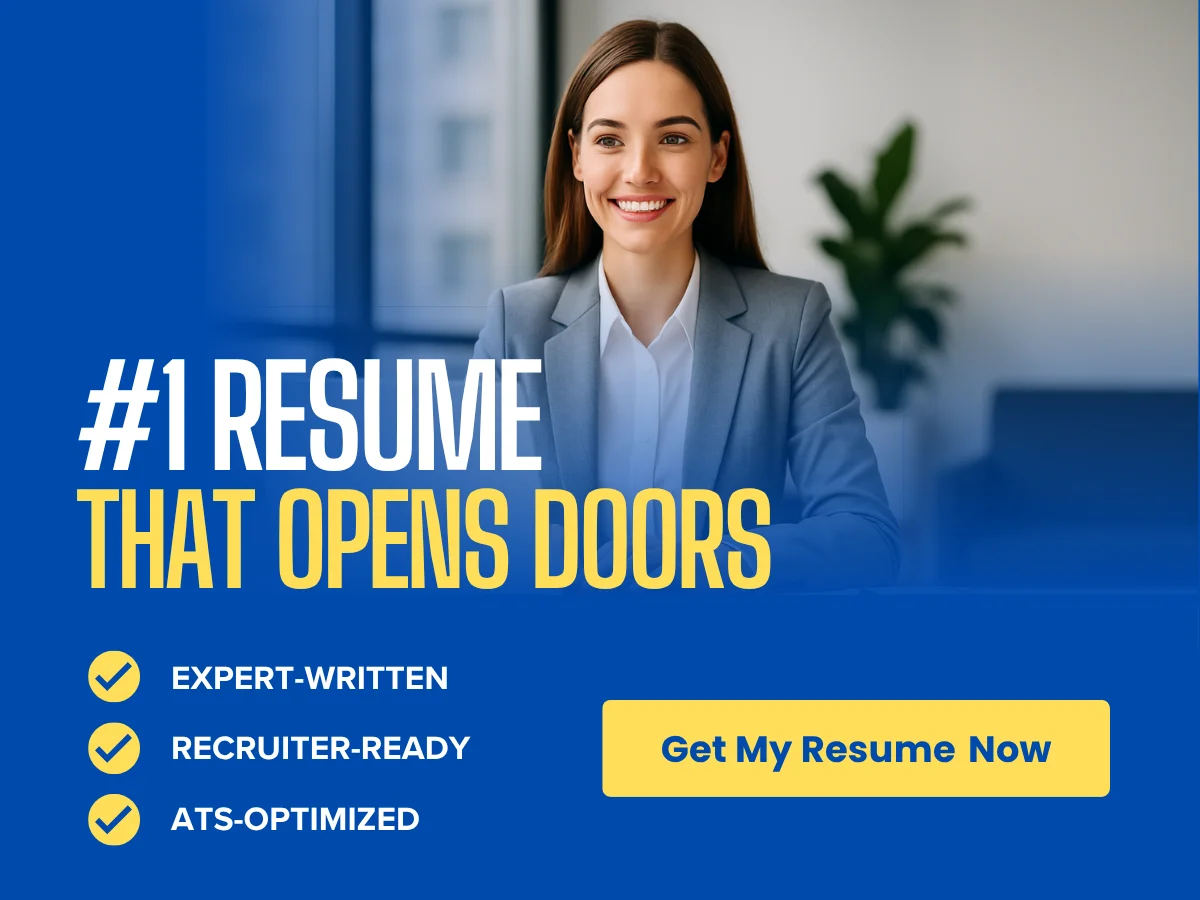