Excel remains a cornerstone for managing and analyzing information. However, as the volume of data grows, so does the need for efficiency and automation. Enter Python—a powerful programming language that can transform the way you interact with Excel sheets. Whether you’re a business analyst, a data enthusiast, or simply someone looking to streamline repetitive tasks, learning to automate Excel with Python can save you countless hours and reduce the risk of human error.
This guide is designed specifically for beginners, breaking down the process of automating Excel sheets into manageable steps. You’ll discover how to leverage popular libraries like pandas and openpyxl to manipulate data, create reports, and perform complex calculations with ease. By the end of this article, you’ll not only have a solid understanding of how to automate your Excel tasks but also the confidence to apply these skills in real-world scenarios.
Join us on this journey to unlock the full potential of your data and elevate your productivity through Python automation. Let’s dive in!
Prerequisites
Before diving into the world of automating Excel sheets using Python, it’s essential to ensure you have a solid foundation in a few key areas. This section will outline the prerequisites necessary for beginners to effectively follow along and implement automation in their Excel workflows.
Basic Knowledge of Python
To automate Excel sheets using Python, a fundamental understanding of the Python programming language is crucial. Here are some key concepts you should be familiar with:
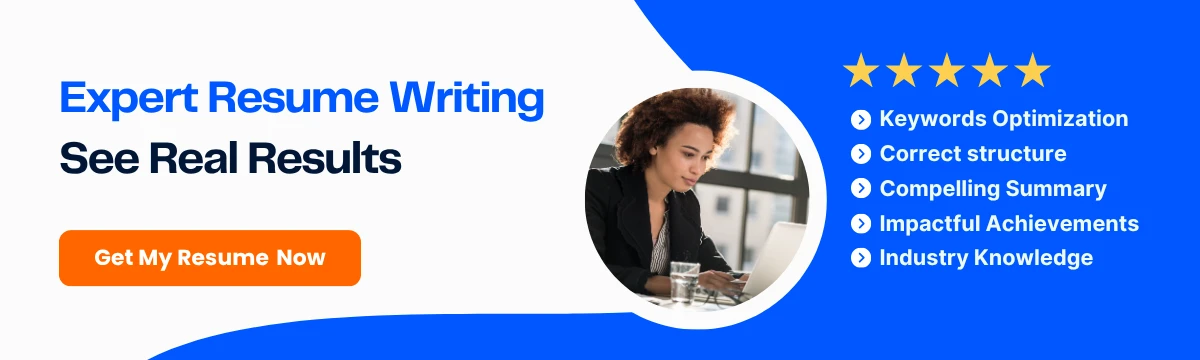
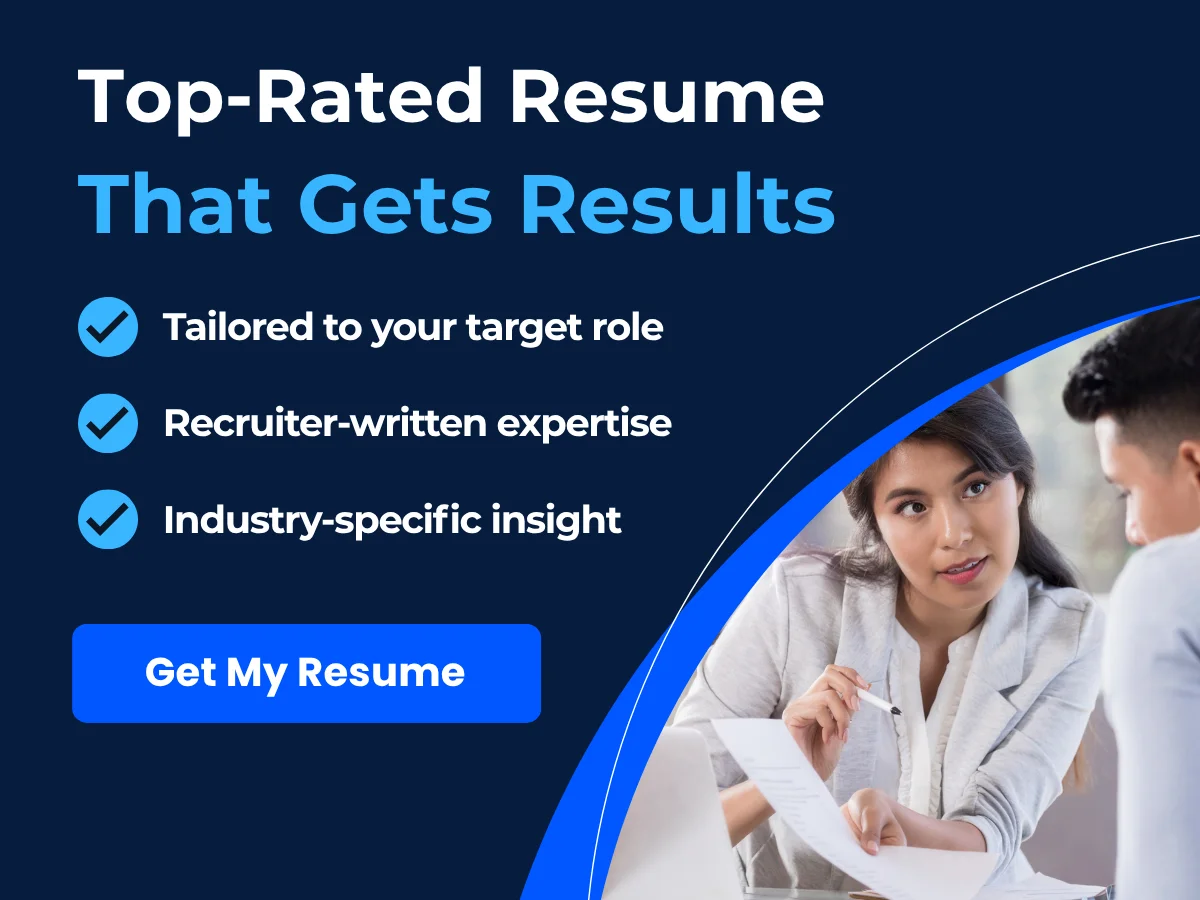
- Variables and Data Types: Understand how to declare variables and the different data types available in Python, such as integers, floats, strings, and lists.
- Control Structures: Familiarity with conditional statements (if-else) and loops (for, while) will help you manipulate data effectively.
- Functions: Knowing how to define and call functions will allow you to organize your code and reuse it efficiently.
- Modules and Libraries: Understanding how to import and use external libraries is essential, as we will be using specific libraries to interact with Excel files.
If you are new to Python, consider taking an introductory course or following online tutorials to build your skills. Websites like Codecademy, Coursera, and freeCodeCamp offer excellent resources for beginners.
Exploring Excel
Having a basic understanding of Microsoft Excel is equally important. Familiarity with Excel’s interface, features, and functionalities will help you better understand how to manipulate data programmatically. Here are some key areas to focus on:
- Excel Interface: Get comfortable navigating the Excel interface, including the ribbon, menus, and various tools available for data manipulation.
- Formulas and Functions: Learn how to use basic Excel formulas and functions, such as SUM, AVERAGE, and VLOOKUP. This knowledge will help you understand how to automate these tasks using Python.
- Data Types: Understand the different data types in Excel, including text, numbers, dates, and how they are formatted.
- Data Organization: Familiarize yourself with how to organize data in rows and columns, use filters, and create tables. This will be crucial when you start automating data entry and manipulation.
Consider practicing with Excel by creating sample spreadsheets, entering data, and using various functions. This hands-on experience will be invaluable when you start automating tasks.
Required Software and Tools
To automate Excel sheets using Python, you will need to install a few software tools and libraries. Below is a list of the essential components you should have set up before proceeding:
- Python: Ensure you have Python installed on your computer. You can download the latest version from the official Python website. During installation, make sure to check the box that says “Add Python to PATH” to make it easier to run Python from the command line.
- IDE or Text Editor: Choose an Integrated Development Environment (IDE) or text editor for writing your Python scripts. Popular options include:
- PyCharm: A powerful IDE specifically designed for Python development.
- Visual Studio Code: A lightweight and versatile code editor with excellent support for Python.
- Jupyter Notebook: An interactive environment that allows you to write and execute Python code in a notebook format, which is great for data analysis and visualization.
- Libraries: You will need to install specific Python libraries to work with Excel files. The most commonly used libraries for Excel automation are:
- pandas: A powerful data manipulation library that provides data structures and functions needed to work with structured data.
- openpyxl: A library for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files.
- xlrd: A library for reading data and formatting information from Excel files in the historical .xls format.
- xlwt: A library for writing data and formatting information to Excel files in the .xls format.
To install these libraries, you can use pip, Python’s package installer. Open your command line or terminal and run the following commands:
pip install pandas openpyxl xlrd xlwt
Once you have installed Python and the necessary libraries, you are ready to start automating Excel sheets. Make sure to test your installation by running a simple Python script that imports these libraries:
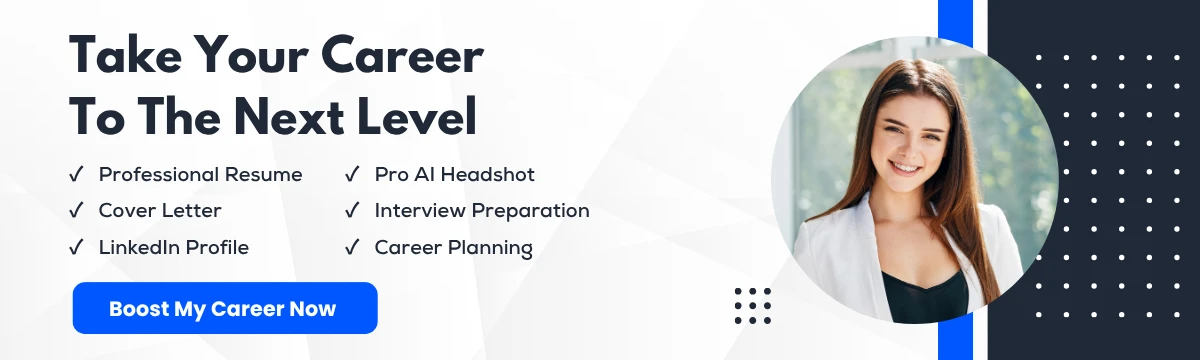
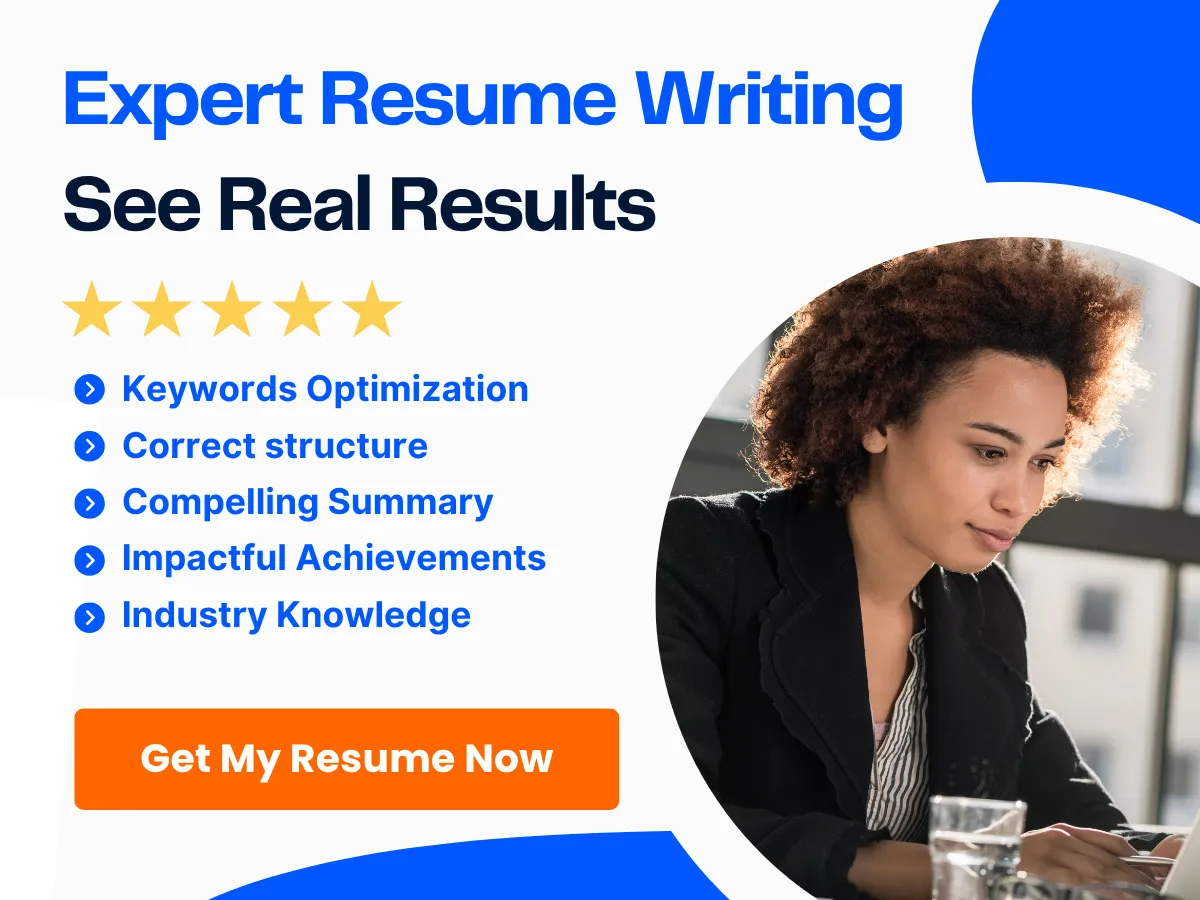
import pandas as pd
import openpyxl
import xlrd
import xlwt
print("Libraries imported successfully!")
If you see the message “Libraries imported successfully!” in your console, you are all set to begin your journey into Excel automation with Python.
With these prerequisites in place, you will be well-equipped to follow along with the upcoming sections of this guide. The combination of Python programming skills, Excel knowledge, and the right tools will empower you to automate repetitive tasks, analyze data efficiently, and enhance your productivity significantly.
Setting Up Your Environment
Before diving into automating Excel sheets with Python, it’s essential to set up your environment correctly. This section will guide you through the necessary steps, including installing Python, required libraries, and setting up a virtual environment. By the end of this section, you will have a fully functional setup ready for Excel automation.
Installing Python
Python is a versatile programming language that is widely used for data manipulation and automation tasks. To get started, you need to install Python on your machine. Follow these steps:
- Download Python: Visit the official Python website and download the latest version of Python. Make sure to choose the version that is compatible with your operating system (Windows, macOS, or Linux).
- Run the Installer: Once the download is complete, run the installer. During the installation process, ensure that you check the box that says Add Python to PATH. This step is crucial as it allows you to run Python from the command line.
-
Verify Installation: After installation, open your command prompt (Windows) or terminal (macOS/Linux) and type the following command:
python --version
If Python is installed correctly, you should see the version number displayed.
Installing Required Libraries
To automate Excel sheets, you will need several Python libraries. The most commonly used libraries for this purpose are pandas, openpyxl, and xlrd. Here’s how to install them:
- Open Command Prompt/Terminal: Launch your command prompt or terminal.
-
Install pip: Pip is the package installer for Python. It usually comes pre-installed with Python. To check if pip is installed, run:
pip --version
If pip is not installed, you can install it by following the instructions on the pip installation page.
-
Install Libraries: Use pip to install the required libraries by running the following commands:
pip install pandas openpyxl xlrd
This command will download and install the latest versions of pandas, openpyxl, and xlrd from the Python Package Index (PyPI).
Understanding the Libraries
Here’s a brief overview of the libraries you just installed:

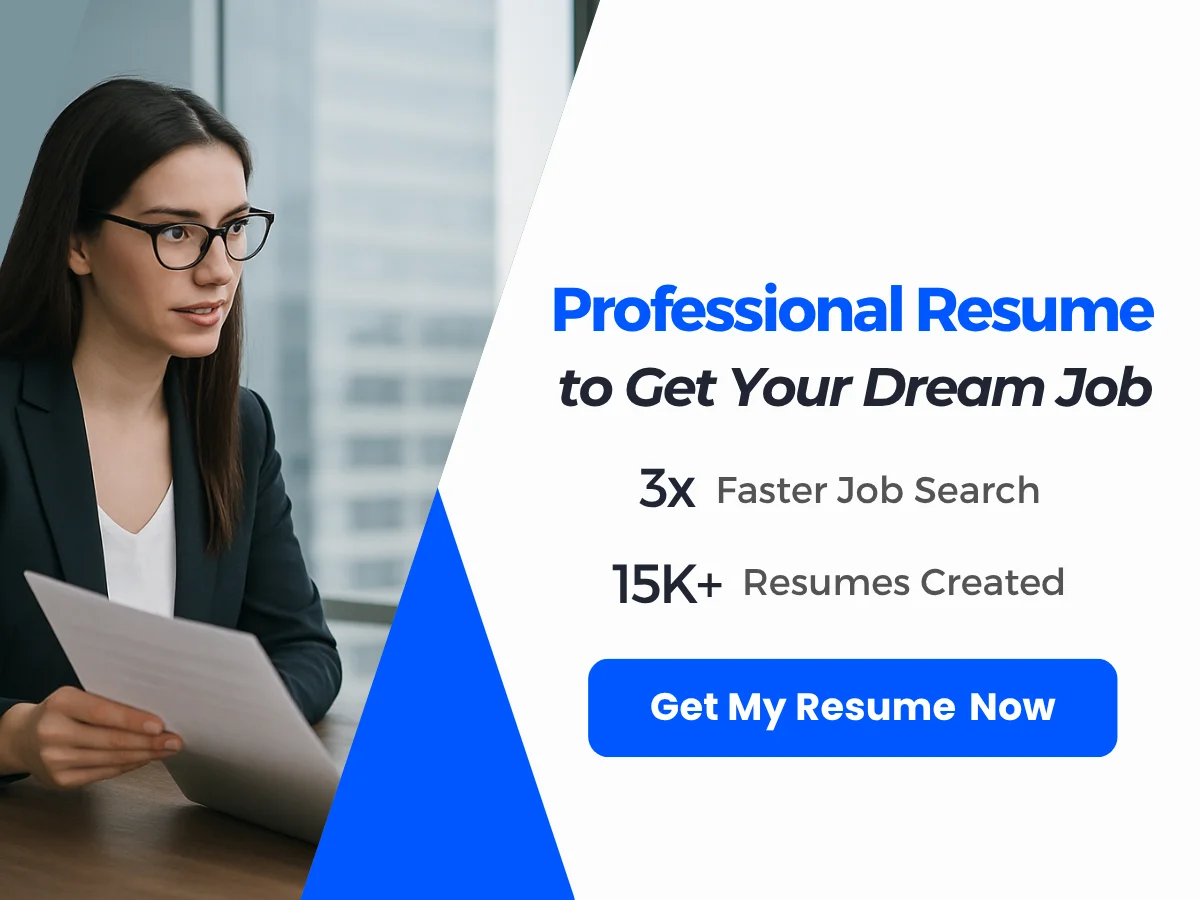
- pandas: A powerful data manipulation and analysis library that provides data structures like DataFrames, which are perfect for handling tabular data such as Excel sheets.
- openpyxl: A library used for reading and writing Excel files in the .xlsx format. It allows you to create, modify, and extract data from Excel files.
- xlrd: A library for reading data from Excel files in the .xls format. While it is less commonly used now due to the prevalence of .xlsx files, it is still useful for legacy Excel files.
Setting Up a Virtual Environment
A virtual environment is a self-contained directory that contains a Python installation for a particular version of Python, plus several additional packages. Using a virtual environment is a best practice as it allows you to manage dependencies for different projects separately. Here’s how to set one up:
-
Install virtualenv: If you don’t have virtualenv installed, you can install it using pip:
pip install virtualenv
-
Create a Virtual Environment: Navigate to your project directory in the command prompt or terminal and run:
virtualenv myenv
Replace myenv with your desired environment name. This command creates a new directory with the specified name, containing a fresh Python installation.
-
Activate the Virtual Environment: To start using the virtual environment, you need to activate it. The command varies based on your operating system:
- Windows:
myenvScriptsactivate
- macOS/Linux:
source myenv/bin/activate
Once activated, your command prompt or terminal will show the name of the virtual environment, indicating that you are now working within it.
- Windows:
-
Install Libraries in the Virtual Environment: With the virtual environment activated, you can now install the required libraries without affecting your global Python installation:
pip install pandas openpyxl xlrd
Deactivating the Virtual Environment
When you are done working in the virtual environment, you can deactivate it by simply running:
deactivate
This command will return you to your global Python environment.
Summary
Setting up your environment is a crucial first step in automating Excel sheets with Python. By installing Python, the necessary libraries, and creating a virtual environment, you ensure that your project is organized and that dependencies are managed effectively. With your environment ready, you can now proceed to explore the exciting world of Excel automation using Python!
Exploring Excel Files
Excel File Formats (XLSX, XLS, CSV)
When working with Excel files in Python, it’s essential to understand the different file formats you may encounter. The most common formats are XLSX, XLS, and CSV.
-
XLSX
The XLSX format is the default file format for Microsoft Excel since the release of Excel 2007. It is based on the Open XML format and allows for a wide range of features, including support for multiple sheets, rich formatting, and the ability to store complex data types. The XLSX format is widely used due to its compatibility with various software applications and libraries.
-
XLS
The XLS format is the older binary file format used by Excel prior to 2007. While it is still supported by modern versions of Excel, it lacks some of the advanced features found in XLSX files. If you are working with legacy systems or older Excel files, you may encounter this format.
-
CSV
CSV (Comma-Separated Values) is a plain text format that uses commas to separate values. It is a simple and widely supported format for storing tabular data. While CSV files are easy to read and write, they do not support advanced Excel features such as multiple sheets, formatting, or formulas. CSV is often used for data exchange between different applications due to its simplicity.
Structure of an Excel Workbook
An Excel workbook is a file that contains one or more worksheets. Understanding the structure of a workbook is crucial for automating tasks in Excel using Python.
-
Workbook
The workbook is the main file that contains all the data and sheets. In Python, when you open an Excel file, you are essentially opening a workbook. Each workbook can contain multiple sheets, which can be accessed programmatically.
-
Worksheet
A worksheet is a single tab within a workbook. Each worksheet consists of a grid of cells organized into rows and columns. You can think of a worksheet as a single page in a notebook where you can enter and manipulate data.
-
Cells
Cells are the individual boxes in a worksheet where data is stored. Each cell is identified by its column letter and row number (e.g., A1, B2). Cells can contain various types of data, including text, numbers, dates, and formulas.
Sheets, Rows, and Columns
To effectively manipulate Excel files using Python, it’s important to understand how sheets, rows, and columns are structured and accessed.
-
Sheets
Each workbook can contain multiple sheets, which can be accessed by their names or indices. In Python, libraries like openpyxl and pandas allow you to read and write data to specific sheets. For example, if you want to access a sheet named “Sales Data,” you can do so by specifying the sheet name in your code.
-
Rows
Rows are horizontal collections of cells in a worksheet. Each row is identified by a number, starting from 1. In Python, you can access rows using their index. For instance, to access the first row of a worksheet, you would use index 0 in most Python libraries, as they are zero-indexed.
-
Columns
Columns are vertical collections of cells in a worksheet. Each column is identified by a letter (A, B, C, etc.). In Python, you can access columns by their letter or index. For example, to access the first column (A), you can use the letter ‘A’ or the index 0, depending on the library you are using.
Accessing Excel Files in Python
To work with Excel files in Python, you will typically use libraries such as openpyxl for XLSX files, xlrd for XLS files, and pandas for both XLSX and CSV files. Below, we will explore how to use these libraries to access and manipulate Excel files.
Using openpyxl
The openpyxl library is a popular choice for reading and writing XLSX files. To get started, you need to install the library:
pip install openpyxl
Here’s a simple example of how to read data from an Excel file using openpyxl:
import openpyxl
# Load the workbook
workbook = openpyxl.load_workbook('example.xlsx')
# Select a sheet
sheet = workbook['Sheet1']
# Access a specific cell
cell_value = sheet['A1'].value
print(f'The value in A1 is: {cell_value}')
# Iterate through rows
for row in sheet.iter_rows(min_row=2, max_col=2, max_row=sheet.max_row):
for cell in row:
print(cell.value)
Using pandas
The pandas library is another powerful tool for data manipulation and analysis. It provides a simple interface for reading and writing Excel files, as well as CSV files. To use pandas, you need to install it along with openpyxl:
pip install pandas openpyxl
Here’s how to read an Excel file using pandas:
import pandas as pd
# Read the Excel file
df = pd.read_excel('example.xlsx', sheet_name='Sheet1')
# Display the first few rows
print(df.head())
Pandas also allows you to write data back to Excel files easily:
df.to_excel('output.xlsx', index=False)
Reading Excel Files
Excel files are a staple in data management and analysis, and Python provides powerful libraries to interact with these files seamlessly. We will explore how to read Excel files using Python, focusing on the pandas
library, which is widely used for data manipulation and analysis. We will cover how to read entire Excel files, access specific sheets, and handle large Excel files efficiently.
Using pandas to Read Excel Files
The pandas
library is a powerful tool for data analysis in Python. It provides a simple and efficient way to read Excel files using the read_excel()
function. Before we dive into the code, ensure you have pandas
and openpyxl
installed, as openpyxl
is required for reading Excel files in the .xlsx format. You can install these libraries using pip:
pip install pandas openpyxl
Once you have the libraries installed, you can start reading Excel files. Here’s a basic example:
import pandas as pd
# Read an Excel file
df = pd.read_excel('path/to/your/file.xlsx')
# Display the first few rows of the DataFrame
print(df.head())
In this example, we import the pandas
library and use the read_excel()
function to read an Excel file located at the specified path. The data is loaded into a DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types. The head()
method is then used to display the first five rows of the DataFrame.
Reading Specific Sheets
Excel files can contain multiple sheets, and you may want to read data from a specific sheet. The read_excel()
function allows you to specify the sheet name or index. Here’s how you can do it:
# Read a specific sheet by name
df_sheet1 = pd.read_excel('path/to/your/file.xlsx', sheet_name='Sheet1')
# Read a specific sheet by index (0 for the first sheet)
df_sheet2 = pd.read_excel('path/to/your/file.xlsx', sheet_name=0)
# Display the first few rows of the specified sheets
print(df_sheet1.head())
print(df_sheet2.head())
In this example, we read two different sheets from the same Excel file. The first DataFrame, df_sheet1
, contains data from a sheet named “Sheet1”, while the second DataFrame, df_sheet2
, contains data from the first sheet (index 0). You can replace the sheet name or index with the appropriate values based on your Excel file.
Handling Large Excel Files
When working with large Excel files, loading the entire file into memory can be inefficient and may lead to performance issues. Fortunately, pandas
provides options to handle large files more effectively. Here are some strategies:
1. Reading a Specific Range of Rows
If you only need a subset of the data, you can specify the rows to read using the nrows
and skiprows
parameters:
# Read only the first 100 rows
df_limited = pd.read_excel('path/to/your/file.xlsx', nrows=100)
# Skip the first 10 rows and read the next 100 rows
df_skipped = pd.read_excel('path/to/your/file.xlsx', skiprows=10, nrows=100)
# Display the DataFrames
print(df_limited.head())
print(df_skipped.head())
In this example, we read only the first 100 rows of the Excel file and also demonstrated how to skip the first 10 rows before reading the next 100 rows. This can significantly reduce memory usage when dealing with large datasets.
2. Using Chunking
Another effective method for handling large Excel files is to read the data in chunks. This is particularly useful when you want to process the data incrementally. You can use the chunksize
parameter to specify the number of rows to read at a time:
# Read the Excel file in chunks of 100 rows
chunk_size = 100
for chunk in pd.read_excel('path/to/your/file.xlsx', chunksize=chunk_size):
# Process each chunk
print(chunk.head())
In this example, we read the Excel file in chunks of 100 rows. The for
loop iterates over each chunk, allowing you to process the data without loading the entire file into memory at once. This is particularly useful for data analysis tasks where you can perform operations on each chunk sequentially.
3. Using the usecols
Parameter
If you only need specific columns from a large Excel file, you can use the usecols
parameter to read only the necessary columns:
# Read specific columns by name
df_columns = pd.read_excel('path/to/your/file.xlsx', usecols=['A', 'C', 'E'])
# Read specific columns by index
df_columns_index = pd.read_excel('path/to/your/file.xlsx', usecols=[0, 2, 4])
# Display the DataFrames
print(df_columns.head())
print(df_columns_index.head())
In this example, we read only columns A, C, and E from the Excel file. This can significantly reduce memory usage and improve performance when working with large datasets.
Writing to Excel Files
Automating Excel sheets using Python can significantly enhance productivity, especially when dealing with large datasets. We will explore how to create new Excel files, write DataFrames to Excel, and format cells and sheets. We will utilize the popular pandas
library along with openpyxl
or xlsxwriter
for advanced formatting options. Let’s dive into each of these topics step-by-step.
Creating a New Excel File
To create a new Excel file in Python, we can use the pandas
library, which provides a simple interface for handling data. First, ensure you have the necessary libraries installed. You can install them using pip:
pip install pandas openpyxl
Once you have the libraries installed, you can create a new Excel file as follows:
import pandas as pd
# Create a simple DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
# Write the DataFrame to a new Excel file
df.to_excel('new_file.xlsx', index=False, engine='openpyxl')
In this example, we created a DataFrame with three columns: Name, Age, and City. The to_excel
method is then used to write this DataFrame to a new Excel file named new_file.xlsx
. The index=False
argument prevents pandas from writing row indices to the file.
Writing DataFrames to Excel
Writing DataFrames to Excel is straightforward with pandas. You can write multiple DataFrames to different sheets within the same Excel file using the ExcelWriter
class. Here’s how you can do it:
# Create another DataFrame
data2 = {
'Product': ['Laptop', 'Tablet', 'Smartphone'],
'Price': [1000, 500, 300],
'Stock': [50, 150, 200]
}
df2 = pd.DataFrame(data2)
# Write both DataFrames to the same Excel file with different sheets
with pd.ExcelWriter('multiple_sheets.xlsx', engine='openpyxl') as writer:
df.to_excel(writer, sheet_name='People', index=False)
df2.to_excel(writer, sheet_name='Products', index=False)
In this code snippet, we created a second DataFrame containing product information. Using the ExcelWriter
context manager, we wrote both DataFrames to a single Excel file named multiple_sheets.xlsx
, with each DataFrame occupying its own sheet.
Formatting Cells and Sheets
Excel files can be formatted to improve readability and presentation. The openpyxl
library allows for extensive formatting options, such as changing font styles, colors, and cell borders. Below are some examples of how to format cells and sheets in an Excel file.
Basic Formatting
Let’s start with some basic formatting options:
from openpyxl import Workbook
from openpyxl.styles import Font, Color, Alignment
# Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
# Add some data
ws['A1'] = 'Name'
ws['B1'] = 'Age'
ws['C1'] = 'City'
# Format the header row
header_font = Font(bold=True, color='FFFFFF')
header_fill = Color(rgb='0000FF')
for cell in ws["1:1"]:
cell.font = header_font
cell.fill = header_fill
cell.alignment = Alignment(horizontal='center')
# Add data
data = [
['Alice', 25, 'New York'],
['Bob', 30, 'Los Angeles'],
['Charlie', 35, 'Chicago']
]
for row in data:
ws.append(row)
# Save the workbook
wb.save('formatted_file.xlsx')
In this example, we created a new workbook and added a header row with bold text and a blue background. The Font
and Color
classes from openpyxl.styles
are used to customize the appearance of the cells. The Alignment
class is used to center the text in the header cells.
Advanced Formatting
For more advanced formatting, you can apply styles to specific cells, set column widths, and even add borders:
from openpyxl.styles import Border, Side
# Define a border style
thin_border = Border(left=Side(style='thin'),
right=Side(style='thin'),
top=Side(style='thin'),
bottom=Side(style='thin'))
# Apply border to all cells in the data range
for row in ws.iter_rows(min_row=1, max_col=3, max_row=4):
for cell in row:
cell.border = thin_border
# Set column widths
ws.column_dimensions['A'].width = 20
ws.column_dimensions['B'].width = 10
ws.column_dimensions['C'].width = 15
# Save the workbook
wb.save('advanced_formatted_file.xlsx')
In this code, we defined a thin border style and applied it to all cells in the specified range. We also set the widths of the columns to ensure that the content fits well. This level of customization can make your Excel files not only functional but also visually appealing.
Conditional Formatting
Conditional formatting allows you to apply styles based on the values in the cells. For example, you might want to highlight cells that meet certain criteria. Here’s how to apply conditional formatting using openpyxl
:
from openpyxl.formatting.rule import ColorScaleRule
# Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
# Add some data
data = [
['Name', 'Score'],
['Alice', 85],
['Bob', 90],
['Charlie', 70],
['David', 95]
]
for row in data:
ws.append(row)
# Apply conditional formatting to the Score column
color_scale_rule = ColorScaleRule(start_type='num', start_value=70, start_color='FF0000',
end_type='num', end_value=100, end_color='00FF00')
ws.conditional_formatting.add('B2:B5', color_scale_rule)
# Save the workbook
wb.save('conditional_formatted_file.xlsx')
In this example, we created a simple dataset with names and scores. We then applied a color scale conditional formatting rule to the Score column, which will color the cells from red to green based on the score values. This visual representation can help quickly identify high and low performers.
By mastering these techniques, you can automate the process of writing to Excel files in Python, making your data management tasks more efficient and effective. Whether you are creating reports, analyzing data, or simply organizing information, Python provides powerful tools to help you achieve your goals.
Modifying Existing Excel Files
When working with Excel files in Python, one of the most common tasks is modifying existing spreadsheets. This can include adding or deleting sheets, inserting or deleting rows and columns, and updating cell values. We will explore these operations in detail using the openpyxl
library, which is a powerful tool for reading and writing Excel files in the .xlsx format.
Adding and Deleting Sheets
Excel allows users to organize data across multiple sheets within a single workbook. With Python’s openpyxl
library, you can easily add new sheets or delete existing ones. Let’s go through the steps to perform these actions.
Adding a New Sheet
To add a new sheet to an existing Excel workbook, you can use the create_sheet()
method. Here’s how you can do it:
import openpyxl
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
# Create a new sheet
new_sheet = workbook.create_sheet(title='NewSheet')
# Save the workbook
workbook.save('example.xlsx')
In this example, we first load an existing workbook named example.xlsx
. We then create a new sheet titled NewSheet
and save the workbook to retain the changes.
Deleting a Sheet
To delete a sheet, you can use the remove()
method. Here’s how to delete a sheet:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
# Remove the sheet
workbook.remove(workbook['NewSheet'])
# Save the workbook
workbook.save('example.xlsx')
In this code snippet, we load the workbook and remove the sheet named NewSheet
. After making the change, we save the workbook again.
Inserting and Deleting Rows and Columns
Modifying the structure of your data can often require inserting or deleting rows and columns. The openpyxl
library provides straightforward methods to accomplish this.
Inserting Rows
To insert a new row, you can use the insert_rows()
method. Here’s an example:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Insert a new row at index 2
sheet.insert_rows(2)
# Save the workbook
workbook.save('example.xlsx')
This code inserts a new row at the second position of the active sheet. The existing rows will shift down to accommodate the new row.
Deleting Rows
To delete a row, you can use the delete_rows()
method. Here’s how to delete a specific row:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Delete the row at index 2
sheet.delete_rows(2)
# Save the workbook
workbook.save('example.xlsx')
In this example, we delete the row at index 2, and the subsequent rows will shift up accordingly.
Inserting Columns
Similar to inserting rows, you can insert a new column using the insert_cols()
method:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Insert a new column at index 2
sheet.insert_cols(2)
# Save the workbook
workbook.save('example.xlsx')
This code snippet inserts a new column at the second position of the active sheet, shifting existing columns to the right.
Deleting Columns
To delete a column, you can use the delete_cols()
method. Here’s how to delete a specific column:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Delete the column at index 2
sheet.delete_cols(2)
# Save the workbook
workbook.save('example.xlsx')
In this example, we delete the column at index 2, and the subsequent columns will shift left.
Updating Cell Values
Updating cell values is one of the most common tasks when modifying Excel files. You can easily change the value of a specific cell using the cell()
method. Here’s how to do it:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Update the value of a specific cell
sheet.cell(row=1, column=1, value='Updated Value')
# Save the workbook
workbook.save('example.xlsx')
In this example, we update the value of the cell located at row 1, column 1 (which corresponds to cell A1) to Updated Value
.
Updating Multiple Cells
If you need to update multiple cells, you can loop through a range of cells. Here’s an example of how to update a range of cells:
# Load the existing workbook
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook.active
# Update multiple cells
for row in range(1, 4): # Rows 1 to 3
for col in range(1, 4): # Columns 1 to 3
sheet.cell(row=row, column=col, value=f'Row {row}, Col {col}')
# Save the workbook
workbook.save('example.xlsx')
This code updates the first three rows and columns of the active sheet with a formatted string indicating their position.
Best Practices for Modifying Excel Files
When modifying Excel files, it’s essential to follow best practices to ensure data integrity and avoid errors:
- Backup Your Files: Always create a backup of your Excel files before making modifications, especially if they contain critical data.
- Use Context Managers: Consider using context managers (the
with
statement) when working with files to ensure they are properly closed after operations. - Validate Changes: After making changes, validate that the modifications were successful by reading back the data.
- Document Your Code: Comment your code to explain the purpose of each operation, making it easier for others (or yourself) to understand later.
By following these guidelines and utilizing the openpyxl
library, you can efficiently modify existing Excel files in Python, enhancing your data manipulation capabilities.
Advanced Data Manipulation
Once you have a basic understanding of how to automate Excel sheets using Python, you can take your skills to the next level by mastering advanced data manipulation techniques. This section will cover three key areas: filtering and sorting data, merging and splitting sheets, and using formulas and functions. By the end of this guide, you will be equipped with the knowledge to handle complex data tasks efficiently.
Filtering and Sorting Data
Filtering and sorting data are essential tasks when working with large datasets. Python, particularly with the help of the pandas
library, makes these tasks straightforward and efficient.
Filtering Data
Filtering allows you to display only the rows that meet certain criteria. For example, if you have a dataset of sales records and you want to see only the sales that exceed a certain amount, you can easily filter the data using pandas
.
import pandas as pd
# Load the Excel file
df = pd.read_excel('sales_data.xlsx')
# Filter the data for sales greater than $500
filtered_data = df[df['Sales'] > 500]
# Display the filtered data
print(filtered_data)
In this example, we load an Excel file containing sales data and filter it to show only those records where the sales amount exceeds $500. The result is a new DataFrame containing only the relevant rows.
Sorting Data
Sorting data is another critical operation that allows you to organize your dataset in a meaningful way. You can sort data by one or more columns in ascending or descending order.
# Sort the data by the 'Sales' column in descending order
sorted_data = df.sort_values(by='Sales', ascending=False)
# Display the sorted data
print(sorted_data)
In this snippet, we sort the sales data in descending order based on the ‘Sales’ column. You can also sort by multiple columns by passing a list to the by
parameter:
# Sort by 'Region' and then by 'Sales'
sorted_data = df.sort_values(by=['Region', 'Sales'], ascending=[True, False])
This will first sort the data by ‘Region’ in ascending order and then by ‘Sales’ in descending order within each region.
Merging and Splitting Sheets
When working with multiple datasets, you often need to merge or split sheets to create a cohesive dataset. The pandas
library provides powerful functions to handle these tasks.
Merging Sheets
Merging allows you to combine two or more DataFrames based on a common column. This is particularly useful when you have related data spread across different sheets.
# Load two Excel sheets
df1 = pd.read_excel('sales_data.xlsx', sheet_name='Q1')
df2 = pd.read_excel('sales_data.xlsx', sheet_name='Q2')
# Merge the two DataFrames on the 'Product ID' column
merged_data = pd.merge(df1, df2, on='Product ID', how='outer')
# Display the merged data
print(merged_data)
In this example, we load two sheets from the same Excel file and merge them based on the ‘Product ID’ column. The how='outer'
parameter ensures that all records from both sheets are included, even if there are no matching ‘Product ID’ values.
Splitting Sheets
Sometimes, you may need to split a DataFrame into multiple sheets based on certain criteria. For instance, if you want to create separate sheets for each region in your sales data, you can do so using the following approach:
# Create a Pandas Excel writer using XlsxWriter as the engine
with pd.ExcelWriter('sales_by_region.xlsx') as writer:
for region, group in df.groupby('Region'):
group.to_excel(writer, sheet_name=region, index=False)
In this code, we use the groupby
function to group the data by ‘Region’. We then iterate over each group and write it to a separate sheet in a new Excel file called ‘sales_by_region.xlsx’. Each sheet will be named after the corresponding region.
Using Formulas and Functions
Excel is known for its powerful formulas and functions, and you can leverage these capabilities in Python as well. The openpyxl
library allows you to read and write Excel files while also enabling you to use Excel formulas.
Writing Formulas
To write a formula into a cell, you can use the openpyxl
library. Here’s how you can do it:
from openpyxl import Workbook
# Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
# Write some data
ws['A1'] = 'Sales'
ws['A2'] = 100
ws['A3'] = 200
# Write a formula to sum the sales
ws['A4'] = '=SUM(A2:A3)'
# Save the workbook
wb.save('sales_with_formula.xlsx')
In this example, we create a new Excel workbook, write some sales data, and then write a formula to sum the sales in cell A4. When you open the resulting Excel file, you will see the calculated sum in cell A4.
Using Functions
In addition to writing formulas, you can also use built-in functions from pandas
to perform calculations on your DataFrame. For example, you can calculate the average sales:
# Calculate the average sales
average_sales = df['Sales'].mean()
# Display the average sales
print(f'Average Sales: {average_sales}')
This code calculates the average of the ‘Sales’ column and prints the result. You can use various other functions like sum()
, max()
, min()
, and count()
to perform different calculations on your data.
By mastering these advanced data manipulation techniques, you can significantly enhance your ability to work with Excel sheets in Python. Whether you are filtering and sorting data, merging and splitting sheets, or using formulas and functions, these skills will empower you to handle complex data tasks with ease.
Automating Repetitive Tasks
In the world of data management, Excel is a powerful tool that many professionals rely on for organizing, analyzing, and presenting data. However, performing repetitive tasks in Excel can be time-consuming and prone to human error. Fortunately, Python offers a robust solution for automating these tasks, allowing users to streamline their workflows and focus on more critical aspects of their work. We will explore how to automate repetitive tasks in Excel using Python, covering key areas such as looping through files and sheets, automating data entry, and automating data analysis.
Looping Through Files and Sheets
One of the most common tasks in Excel automation is processing multiple files or sheets. For instance, you may have a folder filled with Excel files that need to be consolidated into a single report. Python, with libraries like pandas
and openpyxl
, makes it easy to loop through these files and perform operations on each one.
Setting Up Your Environment
Before we dive into the code, ensure you have the necessary libraries installed. You can install them using pip:
pip install pandas openpyxl
Example: Looping Through Excel Files
Let’s say you have multiple Excel files in a directory, and you want to read data from each file and combine it into a single DataFrame. Here’s how you can do it:
import pandas as pd
import os
# Define the directory containing the Excel files
directory = 'path/to/excel/files'
# Create an empty list to store DataFrames
dataframes = []
# Loop through each file in the directory
for filename in os.listdir(directory):
if filename.endswith('.xlsx'):
file_path = os.path.join(directory, filename)
# Read the Excel file
df = pd.read_excel(file_path)
# Append the DataFrame to the list
dataframes.append(df)
# Concatenate all DataFrames into a single DataFrame
combined_df = pd.concat(dataframes, ignore_index=True)
# Display the combined DataFrame
print(combined_df)
In this example, we first import the necessary libraries and define the directory containing our Excel files. We then loop through each file, read it into a DataFrame, and append it to a list. Finally, we concatenate all DataFrames into a single DataFrame, which can be used for further analysis or reporting.
Automating Data Entry
Data entry is another area where automation can save significant time and reduce errors. Instead of manually entering data into Excel, you can use Python to automate this process. This is particularly useful when dealing with large datasets or when data needs to be updated frequently.
Example: Writing Data to Excel
Let’s consider a scenario where you have a list of new entries that need to be added to an existing Excel sheet. Here’s how you can automate this process:
import pandas as pd
# Define the path to the existing Excel file
file_path = 'path/to/existing/file.xlsx'
# Load the existing Excel file
df_existing = pd.read_excel(file_path)
# Create a new DataFrame with the data to be added
new_data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df_new = pd.DataFrame(new_data)
# Append the new data to the existing DataFrame
df_combined = pd.concat([df_existing, df_new], ignore_index=True)
# Write the updated DataFrame back to the Excel file
df_combined.to_excel(file_path, index=False)
print("Data entry automated successfully!")
In this example, we first load the existing Excel file into a DataFrame. We then create a new DataFrame containing the data we want to add. By using pd.concat()
, we combine the existing and new DataFrames and write the updated DataFrame back to the original Excel file. This process eliminates the need for manual data entry, ensuring accuracy and efficiency.
Automating Data Analysis
Data analysis is a critical function in many organizations, and automating this process can lead to faster insights and better decision-making. Python’s pandas
library provides powerful tools for data manipulation and analysis, making it an excellent choice for automating these tasks.
Example: Performing Data Analysis
Let’s say you have a dataset containing sales data, and you want to analyze the total sales by product category. Here’s how you can automate this analysis:
import pandas as pd
# Load the sales data from an Excel file
file_path = 'path/to/sales_data.xlsx'
df_sales = pd.read_excel(file_path)
# Group the data by product category and calculate total sales
sales_summary = df_sales.groupby('Category')['Sales'].sum().reset_index()
# Write the summary to a new Excel file
sales_summary.to_excel('path/to/sales_summary.xlsx', index=False)
print("Data analysis automated successfully!")
In this example, we load the sales data from an Excel file and use the groupby()
function to group the data by product category. We then calculate the total sales for each category and write the summary to a new Excel file. This automation not only saves time but also ensures that the analysis is consistent and repeatable.
Advanced Data Analysis Techniques
Beyond basic aggregations, Python allows for more advanced data analysis techniques, such as pivot tables, statistical analysis, and data visualization. For instance, you can use the pivot_table()
function in pandas to create pivot tables directly from your DataFrame:
pivot_table = df_sales.pivot_table(values='Sales', index='Category', columns='Region', aggfunc='sum', fill_value=0)
# Write the pivot table to an Excel file
pivot_table.to_excel('path/to/pivot_table.xlsx')
print("Pivot table created successfully!")
This code snippet creates a pivot table that summarizes sales by category and region, filling in any missing values with zero. The resulting pivot table can provide valuable insights into sales performance across different regions and categories.
Error Handling and Debugging
When automating Excel sheets using Python, encountering errors is a common occurrence. Whether it’s due to incorrect data types, file paths, or library-specific issues, understanding how to handle these errors effectively is crucial for a smooth automation process. We will explore common errors, debugging techniques, and best practices for logging and monitoring your Python scripts.
Common Errors and How to Fix Them
As you work with Python libraries like pandas
and openpyxl
to manipulate Excel files, you may run into several common errors. Here are some of the most frequent issues and how to resolve them:
-
FileNotFoundError:
This error occurs when the specified Excel file cannot be found. It often results from an incorrect file path or filename. To fix this, ensure that the file path is correct and that the file exists in the specified location. You can use the
os
library to check if the file exists:import os if not os.path.exists('path/to/your/file.xlsx'): print("File not found!")
-
ValueError:
This error can occur when you try to perform operations on data that is not in the expected format. For example, if you attempt to convert a string to a float but the string contains non-numeric characters, a ValueError will be raised. To handle this, you can use
try-except
blocks:try: value = float("some_string") except ValueError: print("Could not convert to float.")
-
KeyError:
A KeyError occurs when you try to access a dictionary key or DataFrame column that does not exist. To avoid this, always check if the key or column is present before accessing it:
if 'column_name' in df.columns: print(df['column_name']) else: print("Column not found.")
-
PermissionError:
This error arises when you try to open a file that is already open in another program or when you lack the necessary permissions to access the file. Ensure that the file is closed in other applications and that you have the right permissions to access it.
Debugging Tips and Tricks
Debugging is an essential skill for any programmer. Here are some effective tips and tricks to help you debug your Python scripts when automating Excel sheets:
-
Use Print Statements:
One of the simplest ways to debug your code is by inserting print statements at various points in your script. This allows you to track the flow of execution and inspect variable values:
print("Current value of variable:", variable_name)
-
Utilize Python’s Built-in Debugger:
Python comes with a built-in debugger called
pdb
. You can set breakpoints in your code and step through it line by line. To use it, insert the following line where you want to start debugging:import pdb; pdb.set_trace()
This will pause execution and allow you to inspect variables and control the flow of the program.
-
Check Data Types:
Many errors arise from incorrect data types. Use the
type()
function to check the data types of your variables and ensure they match the expected types:print(type(variable_name))
-
Use Assertions:
Assertions are a great way to enforce conditions in your code. If an assertion fails, it raises an
AssertionError
, which can help you identify issues early:assert isinstance(variable_name, expected_type), "Variable is not of expected type!"
Logging and Monitoring
Effective logging and monitoring are vital for maintaining and troubleshooting your Python scripts. Here’s how to implement logging in your Excel automation projects:
-
Using the Logging Module:
Python’s built-in
logging
module provides a flexible framework for emitting log messages from Python programs. You can log messages to different destinations, including the console and files. Here’s a basic setup:import logging # Configure logging logging.basicConfig(filename='automation.log', level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s') # Log an info message logging.info('Starting the Excel automation script.')
-
Log Levels:
The logging module supports different log levels, including DEBUG, INFO, WARNING, ERROR, and CRITICAL. Use these levels to categorize your log messages:
logging.debug('This is a debug message.') logging.warning('This is a warning message.') logging.error('This is an error message.')
-
Monitoring Script Execution:
In addition to logging, you can monitor the execution of your script by logging the start and end times of significant operations. This can help you identify performance bottlenecks:
import time start_time = time.time() # Your code here end_time = time.time() logging.info(f'Execution time: {end_time - start_time} seconds')
By implementing these error handling and debugging techniques, you can significantly improve the reliability and maintainability of your Python scripts for automating Excel sheets. Remember that debugging is an iterative process, and the more you practice, the better you will become at identifying and resolving issues quickly.
Integrating with Other Tools
In the world of data management and analysis, Excel is a powerful tool that many professionals rely on. However, to maximize its potential, integrating Excel with other tools can significantly enhance your workflow. We will explore how to automate Excel sheets in Python by sending emails with Excel attachments, connecting to databases, and using APIs to fetch and update data. Each of these integrations will be explained step-by-step, providing you with practical examples to implement in your projects.
Sending Emails with Excel Attachments
One of the most common tasks in data management is sharing reports or data analysis results via email. Python makes it easy to automate this process, allowing you to send Excel files as email attachments. We will use the pandas
library to create an Excel file and the smtplib
library to send the email.
Step 1: Install Required Libraries
First, ensure you have the necessary libraries installed. You can install them using pip:
pip install pandas openpyxl
Step 2: Create an Excel File
Let’s create a simple Excel file using pandas
. Here’s an example of how to create a DataFrame and save it as an Excel file:
import pandas as pd
# Sample data
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
# Create DataFrame
df = pd.DataFrame(data)
# Save to Excel
excel_file = 'sample_data.xlsx'
df.to_excel(excel_file, index=False)
Step 3: Send Email with Attachment
Now that we have our Excel file, we can send it via email. Below is a simple function to send an email with the Excel file attached:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
def send_email(subject, body, to_email, attachment):
from_email = '[email protected]'
password = 'your_password'
# Create the email
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# Attach the body
msg.attach(MIMEText(body, 'plain'))
# Attach the Excel file
with open(attachment, 'rb') as file:
part = MIMEBase('application', 'octet-stream')
part.set_payload(file.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={attachment}')
msg.attach(part)
# Send the email
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, password)
server.send_message(msg)
# Usage
send_email('Monthly Report', 'Please find the attached report.', '[email protected]', excel_file)
Make sure to replace [email protected]
, your_password
, and smtp.example.com
with your actual email credentials and SMTP server details. This function will send an email with the specified subject and body, along with the Excel file as an attachment.
Connecting to Databases
Another powerful integration is connecting Excel to databases. This allows you to pull data directly from a database into your Excel sheets or push data from Excel back to the database. We will use the SQLAlchemy
library to facilitate this connection.
Step 1: Install SQLAlchemy
First, install the SQLAlchemy library:
pip install sqlalchemy
Step 2: Connect to a Database
Here’s how to connect to a SQLite database and read data into a pandas DataFrame:
from sqlalchemy import create_engine
# Create a database connection
engine = create_engine('sqlite:///example.db')
# Read data from a table
df = pd.read_sql('SELECT * FROM your_table', con=engine)
# Display the DataFrame
print(df)
Replace your_table
with the name of the table you want to query. This code will read the data from the specified table and load it into a pandas DataFrame.
Step 3: Write Data Back to the Database
You can also write data from a DataFrame back to the database. Here’s how to do it:
# Assuming df is your DataFrame
df.to_sql('your_table', con=engine, if_exists='replace', index=False)
This command will replace the existing table with the DataFrame data. You can change if_exists='replace'
to if_exists='append'
if you want to add data instead of replacing it.
Using APIs to Fetch and Update Data
APIs (Application Programming Interfaces) are another excellent way to integrate Excel with external data sources. You can use APIs to fetch data from web services and update your Excel sheets accordingly. We will use the requests
library to interact with an API.
Step 1: Install the Requests Library
First, install the requests library:
pip install requests
Step 2: Fetch Data from an API
Here’s an example of how to fetch data from a public API and load it into a DataFrame:
import requests
# Fetch data from an API
response = requests.get('https://api.example.com/data')
data = response.json()
# Load data into a DataFrame
df = pd.DataFrame(data)
# Display the DataFrame
print(df)
Replace https://api.example.com/data
with the actual API endpoint you want to use. This code will fetch the data in JSON format and convert it into a pandas DataFrame.
Step 3: Update Data via API
To update data using an API, you can use the requests.post()
method. Here’s an example:
# Data to be updated
update_data = {'key': 'value'}
# Send a POST request to update data
response = requests.post('https://api.example.com/update', json=update_data)
# Check the response
print(response.status_code, response.json())
Again, replace https://api.example.com/update
with the actual API endpoint for updating data. This code sends a POST request with the data you want to update.
By integrating Excel with email, databases, and APIs, you can automate many tasks that would otherwise be time-consuming. These integrations not only save time but also reduce the risk of errors associated with manual data entry and reporting. As you become more comfortable with these techniques, you can explore more complex workflows and further enhance your data management capabilities.
Best Practices
Writing Clean and Maintainable Code
When automating Excel sheets in Python, writing clean and maintainable code is crucial for long-term success. Clean code not only makes it easier for you to understand your own work later but also allows others to collaborate effectively. Here are some best practices to consider:
- Use Meaningful Variable Names: Choose variable names that clearly describe their purpose. For example, instead of using
data
, usesales_data
oremployee_records
. This practice enhances readability and helps others understand your code quickly. - Comment Your Code: While your code should be self-explanatory, adding comments can clarify complex logic or important decisions. Use comments to explain why certain choices were made, especially if they are not immediately obvious.
- Organize Your Code: Structure your code into functions and classes. This modular approach allows you to reuse code and makes it easier to test individual components. For instance, if you have a function that processes data, keep it separate from the function that generates reports.
- Follow PEP 8 Guidelines: Adhering to Python’s PEP 8 style guide will help maintain consistency in your code. This includes proper indentation, line length, and spacing. Tools like
flake8
can help you check your code against these standards. - Version Control: Use version control systems like Git to track changes in your code. This practice allows you to revert to previous versions if necessary and facilitates collaboration with others.
Optimizing Performance
Performance optimization is essential when working with large datasets in Excel. Inefficient code can lead to slow execution times, which can be frustrating and counterproductive. Here are some strategies to optimize your Python code when automating Excel tasks:
- Use Efficient Libraries: Libraries like
pandas
andopenpyxl
are optimized for handling large datasets. For instance,pandas
provides powerful data manipulation capabilities and can read and write Excel files efficiently. Always choose the right library for your specific needs. - Batch Processing: Instead of processing data row by row, consider batch processing. For example, if you need to write data to an Excel sheet, collect all the data in a list and write it in one go. This reduces the number of write operations and speeds up the process.
- Minimize Data Copies: When manipulating data, avoid unnecessary copies. For instance, if you are filtering a DataFrame, use the
inplace=True
parameter to modify the original DataFrame instead of creating a new one. - Profile Your Code: Use profiling tools like
cProfile
to identify bottlenecks in your code. This allows you to focus your optimization efforts on the parts of the code that will yield the most significant performance improvements. - Use Vectorized Operations: In libraries like
pandas
, prefer vectorized operations over loops. Vectorized operations are implemented in C and are much faster than Python loops. For example, instead of iterating through a DataFrame to perform calculations, use built-in functions that operate on entire columns.
Ensuring Data Security
Data security is a critical consideration when automating Excel sheets, especially if you are handling sensitive information. Here are some best practices to ensure the security of your data:
- Use Secure Libraries: When working with Excel files, ensure that you are using libraries that support secure file handling. For example,
openpyxl
allows you to set passwords for Excel files, adding an extra layer of security. - Limit Access: If your automated scripts are running on a shared server or environment, ensure that only authorized users have access to the scripts and the data they handle. Use file permissions and user roles to restrict access.
- Encrypt Sensitive Data: If your Excel files contain sensitive information, consider encrypting the data before writing it to the file. You can use libraries like
cryptography
to encrypt data in Python. This ensures that even if someone gains access to the file, they cannot read the sensitive information without the encryption key. - Regular Backups: Implement a backup strategy to protect your data from loss. Regularly back up your Excel files and any databases you may be using. This practice ensures that you can recover your data in case of accidental deletion or corruption.
- Validate Input Data: Always validate the data you are processing. This includes checking for data types, ranges, and formats. By validating input data, you can prevent errors and potential security vulnerabilities that may arise from unexpected data.
By following these best practices, you can ensure that your Python scripts for automating Excel sheets are clean, efficient, and secure. This not only enhances your productivity but also contributes to the overall integrity and reliability of your data management processes.
Examples
Automating Financial Reports
Financial reporting is a critical task for businesses, and automating this process can save significant time and reduce errors. Python, with its powerful libraries, can help you automate the generation of financial reports from raw data stored in Excel sheets.
To illustrate this, let’s consider a scenario where you have a monthly sales data Excel file that includes columns for Date, Product, Sales Amount, and Region. You want to create a financial report that summarizes total sales by product and region.
import pandas as pd
# Load the Excel file
file_path = 'monthly_sales_data.xlsx'
data = pd.read_excel(file_path)
# Grouping the data by Product and Region
report = data.groupby(['Product', 'Region'])['Sales Amount'].sum().reset_index()
# Saving the report to a new Excel file
report.to_excel('financial_report.xlsx', index=False)
In this example, we use the pandas
library to read the Excel file, group the data by Product and Region, and then sum the Sales Amount. Finally, we save the summarized report to a new Excel file. This automation not only speeds up the reporting process but also ensures accuracy in calculations.
Generating Sales Dashboards
Sales dashboards are essential for visualizing key performance indicators (KPIs) and making data-driven decisions. Automating the generation of these dashboards can provide real-time insights into sales performance.
Let’s say you want to create a dashboard that visualizes sales trends over time. You can use Python along with libraries like matplotlib or seaborn to create visualizations directly from your Excel data.
import pandas as pd
import matplotlib.pyplot as plt
# Load the sales data
data = pd.read_excel('monthly_sales_data.xlsx')
# Convert the Date column to datetime format
data['Date'] = pd.to_datetime(data['Date'])
# Grouping the data by month
monthly_sales = data.resample('M', on='Date')['Sales Amount'].sum()
# Plotting the sales trend
plt.figure(figsize=(10, 5))
plt.plot(monthly_sales.index, monthly_sales.values, marker='o')
plt.title('Monthly Sales Trend')
plt.xlabel('Month')
plt.ylabel('Sales Amount')
plt.grid()
plt.savefig('sales_dashboard.png')
plt.show()
In this code snippet, we first load the sales data and convert the Date column to a datetime format. We then resample the data to get monthly sales totals and plot the sales trend using matplotlib
. The resulting graph can be saved as an image file, which can be included in reports or presentations. Automating this process allows for quick updates to the dashboard as new data becomes available.
Data Cleaning and Transformation
Data cleaning and transformation are crucial steps in data analysis, especially when dealing with large datasets. Python provides robust tools to automate these processes, ensuring that your data is clean and ready for analysis.
Consider a scenario where you have an Excel sheet containing customer data, but it includes missing values, inconsistent formatting, and duplicate entries. You can automate the cleaning process using Python.
import pandas as pd
# Load the customer data
data = pd.read_excel('customer_data.xlsx')
# Display the first few rows of the data
print(data.head())
# Handling missing values
data.fillna({'Email': '[email protected]', 'Phone': 'N/A'}, inplace=True)
# Standardizing the format of the 'Name' column
data['Name'] = data['Name'].str.title()
# Removing duplicate entries
data.drop_duplicates(subset='Email', keep='first', inplace=True)
# Saving the cleaned data to a new Excel file
data.to_excel('cleaned_customer_data.xlsx', index=False)
In this example, we load the customer data and handle missing values by filling them with default values. We also standardize the Name column to ensure consistent formatting and remove duplicate entries based on the Email column. Finally, we save the cleaned data to a new Excel file. Automating these cleaning tasks not only saves time but also enhances the quality of your data, making it more reliable for analysis.
By leveraging Python for automating financial reports, generating sales dashboards, and cleaning data, businesses can streamline their operations, improve accuracy, and make informed decisions based on reliable data. The examples provided here are just a starting point; the possibilities for automation in Excel with Python are vast and can be tailored to meet specific business needs.
Frequently Asked Questions (FAQs)
Common Questions and Answers
1. What libraries do I need to automate Excel with Python?
To automate Excel sheets in Python, the most commonly used libraries are pandas and openpyxl. Pandas is excellent for data manipulation and analysis, while openpyxl allows you to read and write Excel files in the .xlsx format. Another useful library is xlrd, which is used for reading data from Excel files, particularly older .xls formats. You can install these libraries using pip:
pip install pandas openpyxl xlrd
2. Can I automate Excel without installing Excel on my machine?
Yes, you can automate Excel without having Microsoft Excel installed on your machine by using libraries like pandas and openpyxl. These libraries allow you to create, read, and manipulate Excel files directly in Python. However, if you need to use Excel-specific features or macros, you will need Excel installed, or you can use alternatives like LibreOffice or Google Sheets API.
3. How do I read an Excel file using Python?
Reading an Excel file in Python is straightforward with the pandas library. Here’s a simple example:
import pandas as pd
# Load the Excel file
df = pd.read_excel('path_to_your_file.xlsx', sheet_name='Sheet1')
# Display the first few rows of the dataframe
print(df.head())
In this example, replace path_to_your_file.xlsx
with the actual path to your Excel file. The sheet_name
parameter allows you to specify which sheet to read.
4. How can I write data to an Excel file?
Writing data to an Excel file can also be done easily with pandas. Here’s how you can do it:
import pandas as pd
# Create a sample DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
# Write the DataFrame to an Excel file
df.to_excel('output_file.xlsx', index=False, sheet_name='Sheet1')
This code creates a new Excel file named output_file.xlsx
and writes the DataFrame to it. The index=False
argument prevents pandas from writing row indices to the file.
5. Can I format Excel files using Python?
Yes, you can format Excel files using the openpyxl library. This library allows you to change font styles, colors, and cell formats. Here’s an example of how to format cells:
from openpyxl import Workbook
from openpyxl.styles import Font, Color
# Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
# Write data to the worksheet
ws['A1'] = 'Name'
ws['B1'] = 'Age'
# Apply bold font to the header
header_font = Font(bold=True)
ws['A1'].font = header_font
ws['B1'].font = header_font
# Save the workbook
wb.save('formatted_output.xlsx')
This code creates a new Excel file with bold headers for the first row. You can explore more formatting options in the openpyxl documentation.
6. How do I handle large Excel files in Python?
When dealing with large Excel files, it’s essential to manage memory efficiently. The pandas library provides options to read and write data in chunks. For example, you can read a large Excel file in smaller parts using the chunksize
parameter:
for chunk in pd.read_excel('large_file.xlsx', chunksize=1000):
# Process each chunk
print(chunk.head())
This approach allows you to process large datasets without loading the entire file into memory at once.
7. What if I encounter errors while automating Excel?
Errors can occur for various reasons, such as file not found, incorrect file format, or issues with data types. Here are some common troubleshooting tips:
- File Not Found: Ensure that the file path is correct and that the file exists in the specified location.
- Invalid File Format: Make sure you are using the correct file format. For example,
openpyxl
works with .xlsx files, whilexlrd
is used for .xls files. - Data Type Issues: If you encounter errors related to data types, check the data in your Excel file. Ensure that numeric columns contain only numbers and that date columns are formatted correctly.
- Library Compatibility: Ensure that you are using compatible versions of the libraries. Sometimes, updating to the latest version can resolve issues.
8. Can I automate Excel tasks using Python scripts?
Absolutely! You can write Python scripts to automate repetitive Excel tasks such as data entry, formatting, and analysis. For example, you can create a script that reads data from one Excel file, processes it, and writes the results to another file. Here’s a simple example:
import pandas as pd
# Read data from an Excel file
df = pd.read_excel('input_file.xlsx')
# Perform some data processing
df['New_Column'] = df['Existing_Column'] * 2
# Write the processed data to a new Excel file
df.to_excel('output_file.xlsx', index=False)
This script reads data from input_file.xlsx
, processes it by creating a new column, and saves the results to output_file.xlsx
.
Troubleshooting Tips
1. Common Errors and Their Solutions
When automating Excel with Python, you may encounter several common errors. Here are some solutions:
- ImportError: If you see an ImportError, ensure that the required libraries are installed. You can install them using pip as mentioned earlier.
- ValueError: This error often occurs when there are issues with data types. Check your data for inconsistencies, such as mixing strings and numbers in the same column.
- PermissionError: If you cannot open or write to a file, ensure that the file is not open in another program and that you have the necessary permissions to access it.
2. Debugging Tips
Debugging your Python scripts can help identify issues quickly. Here are some tips:
- Print Statements: Use print statements to output variable values at different stages of your script. This can help you understand where things might be going wrong.
- Try-Except Blocks: Implement try-except blocks to catch exceptions and handle errors gracefully. This can prevent your script from crashing and provide useful error messages.
- Logging: Consider using the logging module to log messages and errors. This can be especially useful for long-running scripts.
3. Resources for Further Learning
If you want to deepen your understanding of automating Excel with Python, consider exploring the following resources:
- Pandas Documentation – Comprehensive guide to using pandas for data manipulation.
- OpenPyXL Documentation – Detailed documentation for the openpyxl library.
- Real Python – Pandas DataFrame Tutorial – A practical guide to working with DataFrames in pandas.
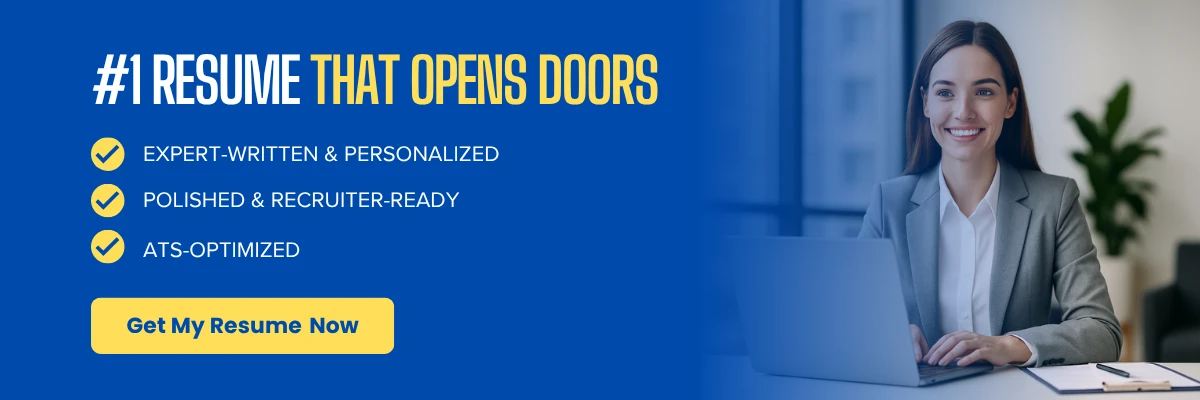
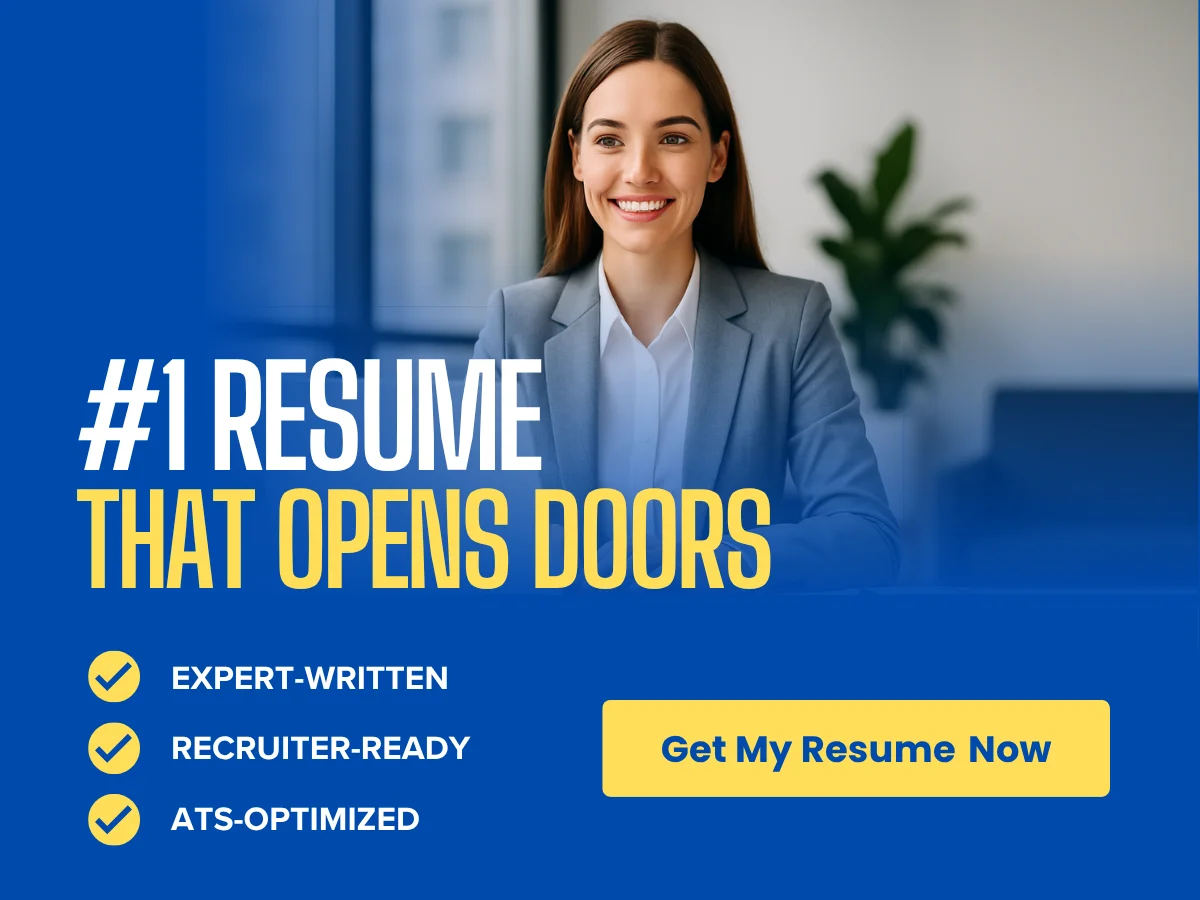